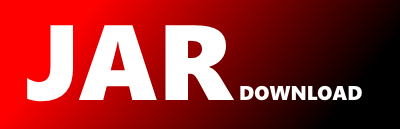
com.pulumi.aws.autoscaling.inputs.LifecycleHookState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.autoscaling.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class LifecycleHookState extends com.pulumi.resources.ResourceArgs {
public static final LifecycleHookState Empty = new LifecycleHookState();
/**
* Name of the Auto Scaling group to which you want to assign the lifecycle hook
*
*/
@Import(name="autoscalingGroupName")
private @Nullable Output autoscalingGroupName;
/**
* @return Name of the Auto Scaling group to which you want to assign the lifecycle hook
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy