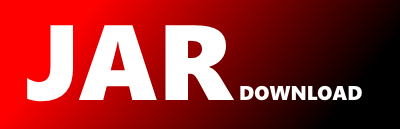
com.pulumi.aws.backup.ReportPlan Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.backup;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.backup.ReportPlanArgs;
import com.pulumi.aws.backup.inputs.ReportPlanState;
import com.pulumi.aws.backup.outputs.ReportPlanReportDeliveryChannel;
import com.pulumi.aws.backup.outputs.ReportPlanReportSetting;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an AWS Backup Report Plan resource.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.backup.ReportPlan;
* import com.pulumi.aws.backup.ReportPlanArgs;
* import com.pulumi.aws.backup.inputs.ReportPlanReportDeliveryChannelArgs;
* import com.pulumi.aws.backup.inputs.ReportPlanReportSettingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new ReportPlan("example", ReportPlanArgs.builder()
* .name("example_name")
* .description("example description")
* .reportDeliveryChannel(ReportPlanReportDeliveryChannelArgs.builder()
* .formats(
* "CSV",
* "JSON")
* .s3BucketName("example-bucket-name")
* .build())
* .reportSetting(ReportPlanReportSettingArgs.builder()
* .reportTemplate("RESTORE_JOB_REPORT")
* .build())
* .tags(Map.of("Name", "Example Report Plan"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import Backup Report Plan using the `id` which corresponds to the name of the Backup Report Plan. For example:
*
* ```sh
* $ pulumi import aws:backup/reportPlan:ReportPlan test <id>
* ```
*
*/
@ResourceType(type="aws:backup/reportPlan:ReportPlan")
public class ReportPlan extends com.pulumi.resources.CustomResource {
/**
* The ARN of the backup report plan.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The ARN of the backup report plan.
*
*/
public Output arn() {
return this.arn;
}
/**
* The date and time that a report plan is created, in Unix format and Coordinated Universal Time (UTC).
*
*/
@Export(name="creationTime", refs={String.class}, tree="[0]")
private Output creationTime;
/**
* @return The date and time that a report plan is created, in Unix format and Coordinated Universal Time (UTC).
*
*/
public Output creationTime() {
return this.creationTime;
}
/**
* The deployment status of a report plan. The statuses are: `CREATE_IN_PROGRESS` | `UPDATE_IN_PROGRESS` | `DELETE_IN_PROGRESS` | `COMPLETED`.
*
*/
@Export(name="deploymentStatus", refs={String.class}, tree="[0]")
private Output deploymentStatus;
/**
* @return The deployment status of a report plan. The statuses are: `CREATE_IN_PROGRESS` | `UPDATE_IN_PROGRESS` | `DELETE_IN_PROGRESS` | `COMPLETED`.
*
*/
public Output deploymentStatus() {
return this.deploymentStatus;
}
/**
* The description of the report plan with a maximum of 1,024 characters
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return The description of the report plan with a maximum of 1,024 characters
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* The unique name of the report plan. The name must be between 1 and 256 characters, starting with a letter, and consisting of letters, numbers, and underscores.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The unique name of the report plan. The name must be between 1 and 256 characters, starting with a letter, and consisting of letters, numbers, and underscores.
*
*/
public Output name() {
return this.name;
}
/**
* An object that contains information about where and how to deliver your reports, specifically your Amazon S3 bucket name, S3 key prefix, and the formats of your reports. Detailed below.
*
*/
@Export(name="reportDeliveryChannel", refs={ReportPlanReportDeliveryChannel.class}, tree="[0]")
private Output reportDeliveryChannel;
/**
* @return An object that contains information about where and how to deliver your reports, specifically your Amazon S3 bucket name, S3 key prefix, and the formats of your reports. Detailed below.
*
*/
public Output reportDeliveryChannel() {
return this.reportDeliveryChannel;
}
/**
* An object that identifies the report template for the report. Reports are built using a report template. Detailed below.
*
*/
@Export(name="reportSetting", refs={ReportPlanReportSetting.class}, tree="[0]")
private Output reportSetting;
/**
* @return An object that identifies the report template for the report. Reports are built using a report template. Detailed below.
*
*/
public Output reportSetting() {
return this.reportSetting;
}
/**
* Metadata that you can assign to help organize the report plans you create. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Metadata that you can assign to help organize the report plans you create. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy