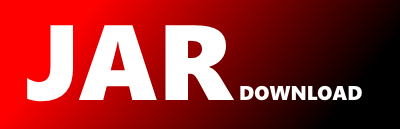
com.pulumi.aws.cloudcontrol.Resource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudcontrol;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.cloudcontrol.ResourceArgs;
import com.pulumi.aws.cloudcontrol.inputs.ResourceState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages a Cloud Control API Resource. The configuration and lifecycle handling of these resources is proxied through Cloud Control API handlers to the backend service.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudcontrol.Resource;
* import com.pulumi.aws.cloudcontrol.ResourceArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Resource("example", ResourceArgs.builder()
* .typeName("AWS::ECS::Cluster")
* .desiredState(serializeJson(
* jsonObject(
* jsonProperty("ClusterName", "example"),
* jsonProperty("Tags", jsonArray(jsonObject(
* jsonProperty("Key", "CostCenter"),
* jsonProperty("Value", "IT")
* )))
* )))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
*/
@ResourceType(type="aws:cloudcontrol/resource:Resource")
public class Resource extends com.pulumi.resources.CustomResource {
/**
* JSON string matching the CloudFormation resource type schema with desired configuration.
*
*/
@Export(name="desiredState", refs={String.class}, tree="[0]")
private Output desiredState;
/**
* @return JSON string matching the CloudFormation resource type schema with desired configuration.
*
*/
public Output desiredState() {
return this.desiredState;
}
/**
* JSON string matching the CloudFormation resource type schema with current configuration. Underlying attributes can be referenced via the `jsondecode()` function, for example, `jsondecode(data.aws_cloudcontrolapi_resource.example.properties)["example"]`.
*
*/
@Export(name="properties", refs={String.class}, tree="[0]")
private Output properties;
/**
* @return JSON string matching the CloudFormation resource type schema with current configuration. Underlying attributes can be referenced via the `jsondecode()` function, for example, `jsondecode(data.aws_cloudcontrolapi_resource.example.properties)["example"]`.
*
*/
public Output properties() {
return this.properties;
}
/**
* Amazon Resource Name (ARN) of the IAM Role to assume for operations.
*
*/
@Export(name="roleArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> roleArn;
/**
* @return Amazon Resource Name (ARN) of the IAM Role to assume for operations.
*
*/
public Output> roleArn() {
return Codegen.optional(this.roleArn);
}
/**
* JSON string of the CloudFormation resource type schema which is used for plan time validation where possible. Automatically fetched if not provided. In large scale environments with multiple resources using the same `type_name`, it is recommended to fetch the schema once via the `aws.cloudformation.CloudFormationType` data source and use this argument to reduce `DescribeType` API operation throttling. This value is marked sensitive only to prevent large plan differences from showing.
*
*/
@Export(name="schema", refs={String.class}, tree="[0]")
private Output schema;
/**
* @return JSON string of the CloudFormation resource type schema which is used for plan time validation where possible. Automatically fetched if not provided. In large scale environments with multiple resources using the same `type_name`, it is recommended to fetch the schema once via the `aws.cloudformation.CloudFormationType` data source and use this argument to reduce `DescribeType` API operation throttling. This value is marked sensitive only to prevent large plan differences from showing.
*
*/
public Output schema() {
return this.schema;
}
/**
* CloudFormation resource type name. For example, `AWS::EC2::VPC`.
*
* The following arguments are optional:
*
*/
@Export(name="typeName", refs={String.class}, tree="[0]")
private Output typeName;
/**
* @return CloudFormation resource type name. For example, `AWS::EC2::VPC`.
*
* The following arguments are optional:
*
*/
public Output typeName() {
return this.typeName;
}
/**
* Identifier of the CloudFormation resource type version.
*
*/
@Export(name="typeVersionId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> typeVersionId;
/**
* @return Identifier of the CloudFormation resource type version.
*
*/
public Output> typeVersionId() {
return Codegen.optional(this.typeVersionId);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Resource(java.lang.String name) {
this(name, ResourceArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Resource(java.lang.String name, ResourceArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Resource(java.lang.String name, ResourceArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:cloudcontrol/resource:Resource", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Resource(java.lang.String name, Output id, @Nullable ResourceState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:cloudcontrol/resource:Resource", name, state, makeResourceOptions(options, id), false);
}
private static ResourceArgs makeArgs(ResourceArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ResourceArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.additionalSecretOutputs(List.of(
"schema"
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Resource get(java.lang.String name, Output id, @Nullable ResourceState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Resource(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy