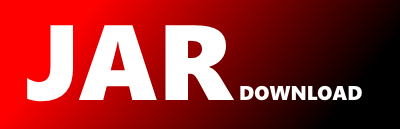
com.pulumi.aws.cloudformation.inputs.StackSetInstanceOperationPreferencesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudformation.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class StackSetInstanceOperationPreferencesArgs extends com.pulumi.resources.ResourceArgs {
public static final StackSetInstanceOperationPreferencesArgs Empty = new StackSetInstanceOperationPreferencesArgs();
/**
* Specifies how the concurrency level behaves during the operation execution. Valid values are `STRICT_FAILURE_TOLERANCE` and `SOFT_FAILURE_TOLERANCE`.
*
*/
@Import(name="concurrencyMode")
private @Nullable Output concurrencyMode;
/**
* @return Specifies how the concurrency level behaves during the operation execution. Valid values are `STRICT_FAILURE_TOLERANCE` and `SOFT_FAILURE_TOLERANCE`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy