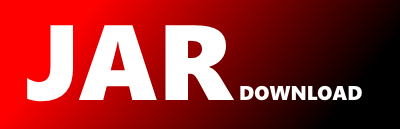
com.pulumi.aws.cloudhsmv2.inputs.ClusterState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudhsmv2.inputs;
import com.pulumi.aws.cloudhsmv2.inputs.ClusterClusterCertificateArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ClusterState extends com.pulumi.resources.ResourceArgs {
public static final ClusterState Empty = new ClusterState();
/**
* The list of cluster certificates.
*
*/
@Import(name="clusterCertificates")
private @Nullable Output> clusterCertificates;
/**
* @return The list of cluster certificates.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy