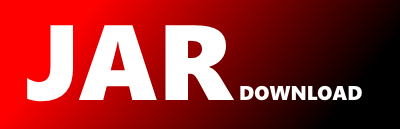
com.pulumi.aws.cloudwatch.MetricStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudwatch;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.cloudwatch.MetricStreamArgs;
import com.pulumi.aws.cloudwatch.inputs.MetricStreamState;
import com.pulumi.aws.cloudwatch.outputs.MetricStreamExcludeFilter;
import com.pulumi.aws.cloudwatch.outputs.MetricStreamIncludeFilter;
import com.pulumi.aws.cloudwatch.outputs.MetricStreamStatisticsConfiguration;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a CloudWatch Metric Stream resource.
*
* ## Example Usage
*
* ### Filters
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.kinesis.FirehoseDeliveryStream;
* import com.pulumi.aws.kinesis.FirehoseDeliveryStreamArgs;
* import com.pulumi.aws.kinesis.inputs.FirehoseDeliveryStreamExtendedS3ConfigurationArgs;
* import com.pulumi.aws.cloudwatch.MetricStream;
* import com.pulumi.aws.cloudwatch.MetricStreamArgs;
* import com.pulumi.aws.cloudwatch.inputs.MetricStreamIncludeFilterArgs;
* import com.pulumi.aws.iam.RolePolicy;
* import com.pulumi.aws.iam.RolePolicyArgs;
* import com.pulumi.aws.s3.BucketAclV2;
* import com.pulumi.aws.s3.BucketAclV2Args;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* // https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/CloudWatch-metric-streams-trustpolicy.html
* final var streamsAssumeRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("streams.metrics.cloudwatch.amazonaws.com")
* .build())
* .actions("sts:AssumeRole")
* .build())
* .build());
*
* var metricStreamToFirehoseRole = new Role("metricStreamToFirehoseRole", RoleArgs.builder()
* .name("metric_stream_to_firehose_role")
* .assumeRolePolicy(streamsAssumeRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
*
* var bucket = new BucketV2("bucket", BucketV2Args.builder()
* .bucket("metric-stream-test-bucket")
* .build());
*
* final var firehoseAssumeRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("firehose.amazonaws.com")
* .build())
* .actions("sts:AssumeRole")
* .build())
* .build());
*
* var firehoseToS3Role = new Role("firehoseToS3Role", RoleArgs.builder()
* .assumeRolePolicy(firehoseAssumeRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
*
* var s3Stream = new FirehoseDeliveryStream("s3Stream", FirehoseDeliveryStreamArgs.builder()
* .name("metric-stream-test-stream")
* .destination("extended_s3")
* .extendedS3Configuration(FirehoseDeliveryStreamExtendedS3ConfigurationArgs.builder()
* .roleArn(firehoseToS3Role.arn())
* .bucketArn(bucket.arn())
* .build())
* .build());
*
* var main = new MetricStream("main", MetricStreamArgs.builder()
* .name("my-metric-stream")
* .roleArn(metricStreamToFirehoseRole.arn())
* .firehoseArn(s3Stream.arn())
* .outputFormat("json")
* .includeFilters(
* MetricStreamIncludeFilterArgs.builder()
* .namespace("AWS/EC2")
* .metricNames(
* "CPUUtilization",
* "NetworkOut")
* .build(),
* MetricStreamIncludeFilterArgs.builder()
* .namespace("AWS/EBS")
* .metricNames()
* .build())
* .build());
*
* // https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/CloudWatch-metric-streams-trustpolicy.html
* final var metricStreamToFirehose = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions(
* "firehose:PutRecord",
* "firehose:PutRecordBatch")
* .resources(s3Stream.arn())
* .build())
* .build());
*
* var metricStreamToFirehoseRolePolicy = new RolePolicy("metricStreamToFirehoseRolePolicy", RolePolicyArgs.builder()
* .name("default")
* .role(metricStreamToFirehoseRole.id())
* .policy(metricStreamToFirehose.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult).applyValue(metricStreamToFirehose -> metricStreamToFirehose.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json())))
* .build());
*
* var bucketAcl = new BucketAclV2("bucketAcl", BucketAclV2Args.builder()
* .bucket(bucket.id())
* .acl("private")
* .build());
*
* final var firehoseToS3 = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions(
* "s3:AbortMultipartUpload",
* "s3:GetBucketLocation",
* "s3:GetObject",
* "s3:ListBucket",
* "s3:ListBucketMultipartUploads",
* "s3:PutObject")
* .resources(
* bucket.arn(),
* bucket.arn().applyValue(arn -> String.format("%s/*", arn)))
* .build())
* .build());
*
* var firehoseToS3RolePolicy = new RolePolicy("firehoseToS3RolePolicy", RolePolicyArgs.builder()
* .name("default")
* .role(firehoseToS3Role.id())
* .policy(firehoseToS3.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult).applyValue(firehoseToS3 -> firehoseToS3.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json())))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Additional Statistics
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.MetricStream;
* import com.pulumi.aws.cloudwatch.MetricStreamArgs;
* import com.pulumi.aws.cloudwatch.inputs.MetricStreamStatisticsConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var main = new MetricStream("main", MetricStreamArgs.builder()
* .name("my-metric-stream")
* .roleArn(metricStreamToFirehose.arn())
* .firehoseArn(s3Stream.arn())
* .outputFormat("json")
* .statisticsConfigurations(
* MetricStreamStatisticsConfigurationArgs.builder()
* .additionalStatistics(
* "p1",
* "tm99")
* .includeMetrics(MetricStreamStatisticsConfigurationIncludeMetricArgs.builder()
* .metricName("CPUUtilization")
* .namespace("AWS/EC2")
* .build())
* .build(),
* MetricStreamStatisticsConfigurationArgs.builder()
* .additionalStatistics("TS(50.5:)")
* .includeMetrics(MetricStreamStatisticsConfigurationIncludeMetricArgs.builder()
* .metricName("CPUUtilization")
* .namespace("AWS/EC2")
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import CloudWatch metric streams using the `name`. For example:
*
* ```sh
* $ pulumi import aws:cloudwatch/metricStream:MetricStream sample sample-stream-name
* ```
*
*/
@ResourceType(type="aws:cloudwatch/metricStream:MetricStream")
public class MetricStream extends com.pulumi.resources.CustomResource {
/**
* ARN of the metric stream.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the metric stream.
*
*/
public Output arn() {
return this.arn;
}
/**
* Date and time in [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8) that the metric stream was created.
*
*/
@Export(name="creationDate", refs={String.class}, tree="[0]")
private Output creationDate;
/**
* @return Date and time in [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8) that the metric stream was created.
*
*/
public Output creationDate() {
return this.creationDate;
}
/**
* List of exclusive metric filters. If you specify this parameter, the stream sends metrics from all metric namespaces except for the namespaces and the conditional metric names that you specify here. If you don't specify metric names or provide empty metric names whole metric namespace is excluded. Conflicts with `include_filter`.
*
*/
@Export(name="excludeFilters", refs={List.class,MetricStreamExcludeFilter.class}, tree="[0,1]")
private Output* @Nullable */ List> excludeFilters;
/**
* @return List of exclusive metric filters. If you specify this parameter, the stream sends metrics from all metric namespaces except for the namespaces and the conditional metric names that you specify here. If you don't specify metric names or provide empty metric names whole metric namespace is excluded. Conflicts with `include_filter`.
*
*/
public Output>> excludeFilters() {
return Codegen.optional(this.excludeFilters);
}
/**
* ARN of the Amazon Kinesis Firehose delivery stream to use for this metric stream.
*
*/
@Export(name="firehoseArn", refs={String.class}, tree="[0]")
private Output firehoseArn;
/**
* @return ARN of the Amazon Kinesis Firehose delivery stream to use for this metric stream.
*
*/
public Output firehoseArn() {
return this.firehoseArn;
}
/**
* List of inclusive metric filters. If you specify this parameter, the stream sends only the conditional metric names from the metric namespaces that you specify here. If you don't specify metric names or provide empty metric names whole metric namespace is included. Conflicts with `exclude_filter`.
*
*/
@Export(name="includeFilters", refs={List.class,MetricStreamIncludeFilter.class}, tree="[0,1]")
private Output* @Nullable */ List> includeFilters;
/**
* @return List of inclusive metric filters. If you specify this parameter, the stream sends only the conditional metric names from the metric namespaces that you specify here. If you don't specify metric names or provide empty metric names whole metric namespace is included. Conflicts with `exclude_filter`.
*
*/
public Output>> includeFilters() {
return Codegen.optional(this.includeFilters);
}
/**
* If you are creating a metric stream in a monitoring account, specify true to include metrics from source accounts that are linked to this monitoring account, in the metric stream. The default is false. For more information about linking accounts, see [CloudWatch cross-account observability](https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/CloudWatch-Unified-Cross-Account.html).
*
*/
@Export(name="includeLinkedAccountsMetrics", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> includeLinkedAccountsMetrics;
/**
* @return If you are creating a metric stream in a monitoring account, specify true to include metrics from source accounts that are linked to this monitoring account, in the metric stream. The default is false. For more information about linking accounts, see [CloudWatch cross-account observability](https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/CloudWatch-Unified-Cross-Account.html).
*
*/
public Output> includeLinkedAccountsMetrics() {
return Codegen.optional(this.includeLinkedAccountsMetrics);
}
/**
* Date and time in [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8) that the metric stream was last updated.
*
*/
@Export(name="lastUpdateDate", refs={String.class}, tree="[0]")
private Output lastUpdateDate;
/**
* @return Date and time in [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8) that the metric stream was last updated.
*
*/
public Output lastUpdateDate() {
return this.lastUpdateDate;
}
/**
* Friendly name of the metric stream. If omitted, the provider will assign a random, unique name. Conflicts with `name_prefix`.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Friendly name of the metric stream. If omitted, the provider will assign a random, unique name. Conflicts with `name_prefix`.
*
*/
public Output name() {
return this.name;
}
/**
* Creates a unique friendly name beginning with the specified prefix. Conflicts with `name`.
*
*/
@Export(name="namePrefix", refs={String.class}, tree="[0]")
private Output namePrefix;
/**
* @return Creates a unique friendly name beginning with the specified prefix. Conflicts with `name`.
*
*/
public Output namePrefix() {
return this.namePrefix;
}
/**
* Output format for the stream. Possible values are `json`, `opentelemetry0.7`, and `opentelemetry1.0`. For more information about output formats, see [Metric streams output formats](https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/CloudWatch-metric-streams-formats.html).
*
* The following arguments are optional:
*
*/
@Export(name="outputFormat", refs={String.class}, tree="[0]")
private Output outputFormat;
/**
* @return Output format for the stream. Possible values are `json`, `opentelemetry0.7`, and `opentelemetry1.0`. For more information about output formats, see [Metric streams output formats](https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/CloudWatch-metric-streams-formats.html).
*
* The following arguments are optional:
*
*/
public Output outputFormat() {
return this.outputFormat;
}
/**
* ARN of the IAM role that this metric stream will use to access Amazon Kinesis Firehose resources. For more information about role permissions, see [Trust between CloudWatch and Kinesis Data Firehose](https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/CloudWatch-metric-streams-trustpolicy.html).
*
*/
@Export(name="roleArn", refs={String.class}, tree="[0]")
private Output roleArn;
/**
* @return ARN of the IAM role that this metric stream will use to access Amazon Kinesis Firehose resources. For more information about role permissions, see [Trust between CloudWatch and Kinesis Data Firehose](https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/CloudWatch-metric-streams-trustpolicy.html).
*
*/
public Output roleArn() {
return this.roleArn;
}
/**
* State of the metric stream. Possible values are `running` and `stopped`.
*
*/
@Export(name="state", refs={String.class}, tree="[0]")
private Output state;
/**
* @return State of the metric stream. Possible values are `running` and `stopped`.
*
*/
public Output state() {
return this.state;
}
/**
* For each entry in this array, you specify one or more metrics and the list of additional statistics to stream for those metrics. The additional statistics that you can stream depend on the stream's `output_format`. If the OutputFormat is `json`, you can stream any additional statistic that is supported by CloudWatch, listed in [CloudWatch statistics definitions](https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/Statistics-definitions.html.html). If the OutputFormat is `opentelemetry0.7` or `opentelemetry1.0`, you can stream percentile statistics (p99 etc.). See details below.
*
*/
@Export(name="statisticsConfigurations", refs={List.class,MetricStreamStatisticsConfiguration.class}, tree="[0,1]")
private Output* @Nullable */ List> statisticsConfigurations;
/**
* @return For each entry in this array, you specify one or more metrics and the list of additional statistics to stream for those metrics. The additional statistics that you can stream depend on the stream's `output_format`. If the OutputFormat is `json`, you can stream any additional statistic that is supported by CloudWatch, listed in [CloudWatch statistics definitions](https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/Statistics-definitions.html.html). If the OutputFormat is `opentelemetry0.7` or `opentelemetry1.0`, you can stream percentile statistics (p99 etc.). See details below.
*
*/
public Output>> statisticsConfigurations() {
return Codegen.optional(this.statisticsConfigurations);
}
/**
* Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy