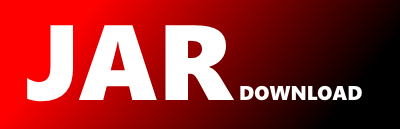
com.pulumi.aws.cloudwatch.inputs.EventConnectionAuthParametersArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudwatch.inputs;
import com.pulumi.aws.cloudwatch.inputs.EventConnectionAuthParametersApiKeyArgs;
import com.pulumi.aws.cloudwatch.inputs.EventConnectionAuthParametersBasicArgs;
import com.pulumi.aws.cloudwatch.inputs.EventConnectionAuthParametersInvocationHttpParametersArgs;
import com.pulumi.aws.cloudwatch.inputs.EventConnectionAuthParametersOauthArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class EventConnectionAuthParametersArgs extends com.pulumi.resources.ResourceArgs {
public static final EventConnectionAuthParametersArgs Empty = new EventConnectionAuthParametersArgs();
/**
* Parameters used for API_KEY authorization. An API key to include in the header for each authentication request. A maximum of 1 are allowed. Conflicts with `basic` and `oauth`. Documented below.
*
*/
@Import(name="apiKey")
private @Nullable Output apiKey;
/**
* @return Parameters used for API_KEY authorization. An API key to include in the header for each authentication request. A maximum of 1 are allowed. Conflicts with `basic` and `oauth`. Documented below.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy