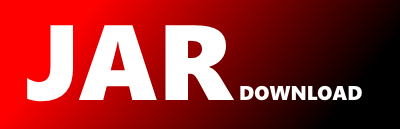
com.pulumi.aws.costexplorer.CostCategory Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.costexplorer;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.costexplorer.CostCategoryArgs;
import com.pulumi.aws.costexplorer.inputs.CostCategoryState;
import com.pulumi.aws.costexplorer.outputs.CostCategoryRule;
import com.pulumi.aws.costexplorer.outputs.CostCategorySplitChargeRule;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a CE Cost Category.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.costexplorer.CostCategory;
* import com.pulumi.aws.costexplorer.CostCategoryArgs;
* import com.pulumi.aws.costexplorer.inputs.CostCategoryRuleArgs;
* import com.pulumi.aws.costexplorer.inputs.CostCategoryRuleRuleArgs;
* import com.pulumi.aws.costexplorer.inputs.CostCategoryRuleRuleDimensionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var test = new CostCategory("test", CostCategoryArgs.builder()
* .name("NAME")
* .ruleVersion("CostCategoryExpression.v1")
* .rules(
* CostCategoryRuleArgs.builder()
* .value("production")
* .rule(CostCategoryRuleRuleArgs.builder()
* .dimension(CostCategoryRuleRuleDimensionArgs.builder()
* .key("LINKED_ACCOUNT_NAME")
* .values("-prod")
* .matchOptions("ENDS_WITH")
* .build())
* .build())
* .build(),
* CostCategoryRuleArgs.builder()
* .value("staging")
* .rule(CostCategoryRuleRuleArgs.builder()
* .dimension(CostCategoryRuleRuleDimensionArgs.builder()
* .key("LINKED_ACCOUNT_NAME")
* .values("-stg")
* .matchOptions("ENDS_WITH")
* .build())
* .build())
* .build(),
* CostCategoryRuleArgs.builder()
* .value("testing")
* .rule(CostCategoryRuleRuleArgs.builder()
* .dimension(CostCategoryRuleRuleDimensionArgs.builder()
* .key("LINKED_ACCOUNT_NAME")
* .values("-dev")
* .matchOptions("ENDS_WITH")
* .build())
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import `aws_ce_cost_category` using the id. For example:
*
* ```sh
* $ pulumi import aws:costexplorer/costCategory:CostCategory example costCategoryARN
* ```
*
*/
@ResourceType(type="aws:costexplorer/costCategory:CostCategory")
public class CostCategory extends com.pulumi.resources.CustomResource {
/**
* ARN of the cost category.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the cost category.
*
*/
public Output arn() {
return this.arn;
}
/**
* Default value for the cost category.
*
*/
@Export(name="defaultValue", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> defaultValue;
/**
* @return Default value for the cost category.
*
*/
public Output> defaultValue() {
return Codegen.optional(this.defaultValue);
}
/**
* Effective end data of your Cost Category.
*
*/
@Export(name="effectiveEnd", refs={String.class}, tree="[0]")
private Output effectiveEnd;
/**
* @return Effective end data of your Cost Category.
*
*/
public Output effectiveEnd() {
return this.effectiveEnd;
}
/**
* The Cost Category's effective start date. It can only be a billing start date (first day of the month). If the date isn't provided, it's the first day of the current month. Dates can't be before the previous twelve months, or in the future. For example `2022-11-01T00:00:00Z`.
*
* The following arguments are optional:
*
*/
@Export(name="effectiveStart", refs={String.class}, tree="[0]")
private Output effectiveStart;
/**
* @return The Cost Category's effective start date. It can only be a billing start date (first day of the month). If the date isn't provided, it's the first day of the current month. Dates can't be before the previous twelve months, or in the future. For example `2022-11-01T00:00:00Z`.
*
* The following arguments are optional:
*
*/
public Output effectiveStart() {
return this.effectiveStart;
}
/**
* Unique name for the Cost Category.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Unique name for the Cost Category.
*
*/
public Output name() {
return this.name;
}
/**
* Rule schema version in this particular Cost Category.
*
*/
@Export(name="ruleVersion", refs={String.class}, tree="[0]")
private Output ruleVersion;
/**
* @return Rule schema version in this particular Cost Category.
*
*/
public Output ruleVersion() {
return this.ruleVersion;
}
/**
* Configuration block for the Cost Category rules used to categorize costs. See below.
*
*/
@Export(name="rules", refs={List.class,CostCategoryRule.class}, tree="[0,1]")
private Output> rules;
/**
* @return Configuration block for the Cost Category rules used to categorize costs. See below.
*
*/
public Output> rules() {
return this.rules;
}
/**
* Configuration block for the split charge rules used to allocate your charges between your Cost Category values. See below.
*
*/
@Export(name="splitChargeRules", refs={List.class,CostCategorySplitChargeRule.class}, tree="[0,1]")
private Output* @Nullable */ List> splitChargeRules;
/**
* @return Configuration block for the split charge rules used to allocate your charges between your Cost Category values. See below.
*
*/
public Output>> splitChargeRules() {
return Codegen.optional(this.splitChargeRules);
}
/**
* Key-value mapping of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value mapping of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy