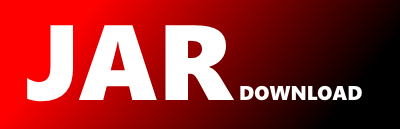
com.pulumi.aws.customerprofiles.inputs.ProfileState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.customerprofiles.inputs;
import com.pulumi.aws.customerprofiles.inputs.ProfileAddressArgs;
import com.pulumi.aws.customerprofiles.inputs.ProfileBillingAddressArgs;
import com.pulumi.aws.customerprofiles.inputs.ProfileMailingAddressArgs;
import com.pulumi.aws.customerprofiles.inputs.ProfileShippingAddressArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ProfileState extends com.pulumi.resources.ResourceArgs {
public static final ProfileState Empty = new ProfileState();
/**
* A unique account number that you have given to the customer.
*
*/
@Import(name="accountNumber")
private @Nullable Output accountNumber;
/**
* @return A unique account number that you have given to the customer.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy