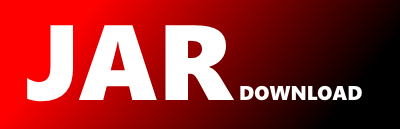
com.pulumi.aws.directconnect.Connection Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.directconnect;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.directconnect.ConnectionArgs;
import com.pulumi.aws.directconnect.inputs.ConnectionState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a Connection of Direct Connect.
*
* ## Example Usage
*
* ### Create a connection
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.directconnect.Connection;
* import com.pulumi.aws.directconnect.ConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var hoge = new Connection("hoge", ConnectionArgs.builder()
* .name("tf-dx-connection")
* .bandwidth("1Gbps")
* .location("EqDC2")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Request a MACsec-capable connection
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.directconnect.Connection;
* import com.pulumi.aws.directconnect.ConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Connection("example", ConnectionArgs.builder()
* .name("tf-dx-connection")
* .bandwidth("10Gbps")
* .location("EqDA2")
* .requestMacsec(true)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Configure encryption mode for MACsec-capable connections
*
* > **NOTE:** You can only specify the `encryption_mode` argument once the connection is in an `Available` state.
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.directconnect.Connection;
* import com.pulumi.aws.directconnect.ConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Connection("example", ConnectionArgs.builder()
* .name("tf-dx-connection")
* .bandwidth("10Gbps")
* .location("EqDC2")
* .requestMacsec(true)
* .encryptionMode("must_encrypt")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import Direct Connect connections using the connection `id`. For example:
*
* ```sh
* $ pulumi import aws:directconnect/connection:Connection test_connection dxcon-ffre0ec3
* ```
*
*/
@ResourceType(type="aws:directconnect/connection:Connection")
public class Connection extends com.pulumi.resources.CustomResource {
/**
* The ARN of the connection.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The ARN of the connection.
*
*/
public Output arn() {
return this.arn;
}
/**
* The Direct Connect endpoint on which the physical connection terminates.
*
*/
@Export(name="awsDevice", refs={String.class}, tree="[0]")
private Output awsDevice;
/**
* @return The Direct Connect endpoint on which the physical connection terminates.
*
*/
public Output awsDevice() {
return this.awsDevice;
}
/**
* The bandwidth of the connection. Valid values for dedicated connections: 1Gbps, 10Gbps. Valid values for hosted connections: 50Mbps, 100Mbps, 200Mbps, 300Mbps, 400Mbps, 500Mbps, 1Gbps, 2Gbps, 5Gbps, 10Gbps and 100Gbps. Case sensitive.
*
*/
@Export(name="bandwidth", refs={String.class}, tree="[0]")
private Output bandwidth;
/**
* @return The bandwidth of the connection. Valid values for dedicated connections: 1Gbps, 10Gbps. Valid values for hosted connections: 50Mbps, 100Mbps, 200Mbps, 300Mbps, 400Mbps, 500Mbps, 1Gbps, 2Gbps, 5Gbps, 10Gbps and 100Gbps. Case sensitive.
*
*/
public Output bandwidth() {
return this.bandwidth;
}
/**
* The connection MAC Security (MACsec) encryption mode. MAC Security (MACsec) is only available on dedicated connections. Valid values are `no_encrypt`, `should_encrypt`, and `must_encrypt`.
*
*/
@Export(name="encryptionMode", refs={String.class}, tree="[0]")
private Output encryptionMode;
/**
* @return The connection MAC Security (MACsec) encryption mode. MAC Security (MACsec) is only available on dedicated connections. Valid values are `no_encrypt`, `should_encrypt`, and `must_encrypt`.
*
*/
public Output encryptionMode() {
return this.encryptionMode;
}
/**
* Indicates whether the connection supports a secondary BGP peer in the same address family (IPv4/IPv6).
*
*/
@Export(name="hasLogicalRedundancy", refs={String.class}, tree="[0]")
private Output hasLogicalRedundancy;
/**
* @return Indicates whether the connection supports a secondary BGP peer in the same address family (IPv4/IPv6).
*
*/
public Output hasLogicalRedundancy() {
return this.hasLogicalRedundancy;
}
/**
* Boolean value representing if jumbo frames have been enabled for this connection.
*
*/
@Export(name="jumboFrameCapable", refs={Boolean.class}, tree="[0]")
private Output jumboFrameCapable;
/**
* @return Boolean value representing if jumbo frames have been enabled for this connection.
*
*/
public Output jumboFrameCapable() {
return this.jumboFrameCapable;
}
/**
* The AWS Direct Connect location where the connection is located. See [DescribeLocations](https://docs.aws.amazon.com/directconnect/latest/APIReference/API_DescribeLocations.html) for the list of AWS Direct Connect locations. Use `locationCode`.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The AWS Direct Connect location where the connection is located. See [DescribeLocations](https://docs.aws.amazon.com/directconnect/latest/APIReference/API_DescribeLocations.html) for the list of AWS Direct Connect locations. Use `locationCode`.
*
*/
public Output location() {
return this.location;
}
/**
* Boolean value indicating whether the connection supports MAC Security (MACsec).
*
*/
@Export(name="macsecCapable", refs={Boolean.class}, tree="[0]")
private Output macsecCapable;
/**
* @return Boolean value indicating whether the connection supports MAC Security (MACsec).
*
*/
public Output macsecCapable() {
return this.macsecCapable;
}
/**
* The name of the connection.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the connection.
*
*/
public Output name() {
return this.name;
}
/**
* The ID of the AWS account that owns the connection.
*
*/
@Export(name="ownerAccountId", refs={String.class}, tree="[0]")
private Output ownerAccountId;
/**
* @return The ID of the AWS account that owns the connection.
*
*/
public Output ownerAccountId() {
return this.ownerAccountId;
}
/**
* The name of the AWS Direct Connect service provider associated with the connection.
*
*/
@Export(name="partnerName", refs={String.class}, tree="[0]")
private Output partnerName;
/**
* @return The name of the AWS Direct Connect service provider associated with the connection.
*
*/
public Output partnerName() {
return this.partnerName;
}
/**
* The MAC Security (MACsec) port link status of the connection.
*
*/
@Export(name="portEncryptionStatus", refs={String.class}, tree="[0]")
private Output portEncryptionStatus;
/**
* @return The MAC Security (MACsec) port link status of the connection.
*
*/
public Output portEncryptionStatus() {
return this.portEncryptionStatus;
}
/**
* The name of the service provider associated with the connection.
*
*/
@Export(name="providerName", refs={String.class}, tree="[0]")
private Output providerName;
/**
* @return The name of the service provider associated with the connection.
*
*/
public Output providerName() {
return this.providerName;
}
/**
* Boolean value indicating whether you want the connection to support MAC Security (MACsec). MAC Security (MACsec) is only available on dedicated connections. See [MACsec prerequisites](https://docs.aws.amazon.com/directconnect/latest/UserGuide/direct-connect-mac-sec-getting-started.html#mac-sec-prerequisites) for more information about MAC Security (MACsec) prerequisites. Default value: `false`.
*
* > **NOTE:** Changing the value of `request_macsec` will cause the resource to be destroyed and re-created.
*
*/
@Export(name="requestMacsec", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> requestMacsec;
/**
* @return Boolean value indicating whether you want the connection to support MAC Security (MACsec). MAC Security (MACsec) is only available on dedicated connections. See [MACsec prerequisites](https://docs.aws.amazon.com/directconnect/latest/UserGuide/direct-connect-mac-sec-getting-started.html#mac-sec-prerequisites) for more information about MAC Security (MACsec) prerequisites. Default value: `false`.
*
* > **NOTE:** Changing the value of `request_macsec` will cause the resource to be destroyed and re-created.
*
*/
public Output> requestMacsec() {
return Codegen.optional(this.requestMacsec);
}
/**
* Set to true if you do not wish the connection to be deleted at destroy time, and instead just removed from the state.
*
*/
@Export(name="skipDestroy", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> skipDestroy;
/**
* @return Set to true if you do not wish the connection to be deleted at destroy time, and instead just removed from the state.
*
*/
public Output> skipDestroy() {
return Codegen.optional(this.skipDestroy);
}
/**
* A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy