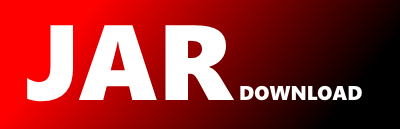
com.pulumi.aws.ec2.Fleet Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.ec2.FleetArgs;
import com.pulumi.aws.ec2.inputs.FleetState;
import com.pulumi.aws.ec2.outputs.FleetFleetInstanceSet;
import com.pulumi.aws.ec2.outputs.FleetLaunchTemplateConfig;
import com.pulumi.aws.ec2.outputs.FleetOnDemandOptions;
import com.pulumi.aws.ec2.outputs.FleetSpotOptions;
import com.pulumi.aws.ec2.outputs.FleetTargetCapacitySpecification;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a resource to manage EC2 Fleets.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.Fleet;
* import com.pulumi.aws.ec2.FleetArgs;
* import com.pulumi.aws.ec2.inputs.FleetLaunchTemplateConfigArgs;
* import com.pulumi.aws.ec2.inputs.FleetLaunchTemplateConfigLaunchTemplateSpecificationArgs;
* import com.pulumi.aws.ec2.inputs.FleetTargetCapacitySpecificationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Fleet("example", FleetArgs.builder()
* .launchTemplateConfigs(FleetLaunchTemplateConfigArgs.builder()
* .launchTemplateSpecification(FleetLaunchTemplateConfigLaunchTemplateSpecificationArgs.builder()
* .launchTemplateId(exampleAwsLaunchTemplate.id())
* .version(exampleAwsLaunchTemplate.latestVersion())
* .build())
* .build())
* .targetCapacitySpecification(FleetTargetCapacitySpecificationArgs.builder()
* .defaultTargetCapacityType("spot")
* .totalTargetCapacity(5)
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import `aws_ec2_fleet` using the Fleet identifier. For example:
*
* ```sh
* $ pulumi import aws:ec2/fleet:Fleet example fleet-b9b55d27-c5fc-41ac-a6f3-48fcc91f080c
* ```
*
*/
@ResourceType(type="aws:ec2/fleet:Fleet")
public class Fleet extends com.pulumi.resources.CustomResource {
/**
* The ARN of the fleet
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The ARN of the fleet
*
*/
public Output arn() {
return this.arn;
}
/**
* Reserved.
*
*/
@Export(name="context", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> context;
/**
* @return Reserved.
*
*/
public Output> context() {
return Codegen.optional(this.context);
}
/**
* Whether running instances should be terminated if the total target capacity of the EC2 Fleet is decreased below the current size of the EC2. Valid values: `no-termination`, `termination`. Defaults to `termination`. Supported only for fleets of type `maintain`.
*
*/
@Export(name="excessCapacityTerminationPolicy", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> excessCapacityTerminationPolicy;
/**
* @return Whether running instances should be terminated if the total target capacity of the EC2 Fleet is decreased below the current size of the EC2. Valid values: `no-termination`, `termination`. Defaults to `termination`. Supported only for fleets of type `maintain`.
*
*/
public Output> excessCapacityTerminationPolicy() {
return Codegen.optional(this.excessCapacityTerminationPolicy);
}
/**
* Information about the instances that were launched by the fleet. Available only when `type` is set to `instant`.
*
*/
@Export(name="fleetInstanceSets", refs={List.class,FleetFleetInstanceSet.class}, tree="[0,1]")
private Output> fleetInstanceSets;
/**
* @return Information about the instances that were launched by the fleet. Available only when `type` is set to `instant`.
*
*/
public Output> fleetInstanceSets() {
return this.fleetInstanceSets;
}
/**
* The state of the EC2 Fleet.
*
*/
@Export(name="fleetState", refs={String.class}, tree="[0]")
private Output fleetState;
/**
* @return The state of the EC2 Fleet.
*
*/
public Output fleetState() {
return this.fleetState;
}
/**
* The number of units fulfilled by this request compared to the set target capacity.
*
*/
@Export(name="fulfilledCapacity", refs={Double.class}, tree="[0]")
private Output fulfilledCapacity;
/**
* @return The number of units fulfilled by this request compared to the set target capacity.
*
*/
public Output fulfilledCapacity() {
return this.fulfilledCapacity;
}
/**
* The number of units fulfilled by this request compared to the set target On-Demand capacity.
*
*/
@Export(name="fulfilledOnDemandCapacity", refs={Double.class}, tree="[0]")
private Output fulfilledOnDemandCapacity;
/**
* @return The number of units fulfilled by this request compared to the set target On-Demand capacity.
*
*/
public Output fulfilledOnDemandCapacity() {
return this.fulfilledOnDemandCapacity;
}
/**
* Nested argument containing EC2 Launch Template configurations. Defined below.
*
*/
@Export(name="launchTemplateConfigs", refs={List.class,FleetLaunchTemplateConfig.class}, tree="[0,1]")
private Output> launchTemplateConfigs;
/**
* @return Nested argument containing EC2 Launch Template configurations. Defined below.
*
*/
public Output> launchTemplateConfigs() {
return this.launchTemplateConfigs;
}
/**
* Nested argument containing On-Demand configurations. Defined below.
*
*/
@Export(name="onDemandOptions", refs={FleetOnDemandOptions.class}, tree="[0]")
private Output* @Nullable */ FleetOnDemandOptions> onDemandOptions;
/**
* @return Nested argument containing On-Demand configurations. Defined below.
*
*/
public Output> onDemandOptions() {
return Codegen.optional(this.onDemandOptions);
}
/**
* Whether EC2 Fleet should replace unhealthy instances. Defaults to `false`. Supported only for fleets of type `maintain`.
*
*/
@Export(name="replaceUnhealthyInstances", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> replaceUnhealthyInstances;
/**
* @return Whether EC2 Fleet should replace unhealthy instances. Defaults to `false`. Supported only for fleets of type `maintain`.
*
*/
public Output> replaceUnhealthyInstances() {
return Codegen.optional(this.replaceUnhealthyInstances);
}
/**
* Nested argument containing Spot configurations. Defined below.
*
*/
@Export(name="spotOptions", refs={FleetSpotOptions.class}, tree="[0]")
private Output* @Nullable */ FleetSpotOptions> spotOptions;
/**
* @return Nested argument containing Spot configurations. Defined below.
*
*/
public Output> spotOptions() {
return Codegen.optional(this.spotOptions);
}
/**
* Map of Fleet tags. To tag instances at launch, specify the tags in the Launch Template. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Map of Fleet tags. To tag instances at launch, specify the tags in the Launch Template. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy