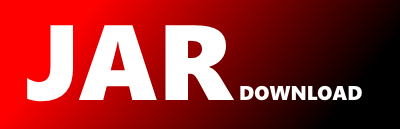
com.pulumi.aws.ec2.inputs.DefaultNetworkAclEgressArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DefaultNetworkAclEgressArgs extends com.pulumi.resources.ResourceArgs {
public static final DefaultNetworkAclEgressArgs Empty = new DefaultNetworkAclEgressArgs();
/**
* The action to take.
*
*/
@Import(name="action", required=true)
private Output action;
/**
* @return The action to take.
*
*/
public Output action() {
return this.action;
}
/**
* The CIDR block to match. This must be a valid network mask.
*
*/
@Import(name="cidrBlock")
private @Nullable Output cidrBlock;
/**
* @return The CIDR block to match. This must be a valid network mask.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy