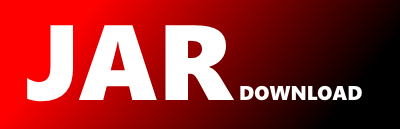
com.pulumi.aws.ecr.outputs.GetRepositoryCreationTemplateResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ecr.outputs;
import com.pulumi.aws.ecr.outputs.GetRepositoryCreationTemplateEncryptionConfiguration;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetRepositoryCreationTemplateResult {
/**
* @return Which features this template applies to. Contains one or more of `PULL_THROUGH_CACHE` or `REPLICATION`.
*
*/
private List appliedFors;
/**
* @return The ARN of the custom role used for repository creation.
*
*/
private String customRoleArn;
/**
* @return The description for this template.
*
*/
private String description;
/**
* @return Encryption configuration for any created repositories. See Encryption Configuration below.
*
*/
private List encryptionConfigurations;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return The tag mutability setting for any created repositories.
*
*/
private String imageTagMutability;
/**
* @return The lifecycle policy document to apply to any created repositories.
*
*/
private String lifecyclePolicy;
private String prefix;
/**
* @return The registry ID the repository creation template applies to.
*
*/
private String registryId;
/**
* @return The registry policy document to apply to any created repositories.
*
*/
private String repositoryPolicy;
/**
* @return A map of tags to assign to any created repositories.
*
*/
private Map resourceTags;
private GetRepositoryCreationTemplateResult() {}
/**
* @return Which features this template applies to. Contains one or more of `PULL_THROUGH_CACHE` or `REPLICATION`.
*
*/
public List appliedFors() {
return this.appliedFors;
}
/**
* @return The ARN of the custom role used for repository creation.
*
*/
public String customRoleArn() {
return this.customRoleArn;
}
/**
* @return The description for this template.
*
*/
public String description() {
return this.description;
}
/**
* @return Encryption configuration for any created repositories. See Encryption Configuration below.
*
*/
public List encryptionConfigurations() {
return this.encryptionConfigurations;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return The tag mutability setting for any created repositories.
*
*/
public String imageTagMutability() {
return this.imageTagMutability;
}
/**
* @return The lifecycle policy document to apply to any created repositories.
*
*/
public String lifecyclePolicy() {
return this.lifecyclePolicy;
}
public String prefix() {
return this.prefix;
}
/**
* @return The registry ID the repository creation template applies to.
*
*/
public String registryId() {
return this.registryId;
}
/**
* @return The registry policy document to apply to any created repositories.
*
*/
public String repositoryPolicy() {
return this.repositoryPolicy;
}
/**
* @return A map of tags to assign to any created repositories.
*
*/
public Map resourceTags() {
return this.resourceTags;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetRepositoryCreationTemplateResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List appliedFors;
private String customRoleArn;
private String description;
private List encryptionConfigurations;
private String id;
private String imageTagMutability;
private String lifecyclePolicy;
private String prefix;
private String registryId;
private String repositoryPolicy;
private Map resourceTags;
public Builder() {}
public Builder(GetRepositoryCreationTemplateResult defaults) {
Objects.requireNonNull(defaults);
this.appliedFors = defaults.appliedFors;
this.customRoleArn = defaults.customRoleArn;
this.description = defaults.description;
this.encryptionConfigurations = defaults.encryptionConfigurations;
this.id = defaults.id;
this.imageTagMutability = defaults.imageTagMutability;
this.lifecyclePolicy = defaults.lifecyclePolicy;
this.prefix = defaults.prefix;
this.registryId = defaults.registryId;
this.repositoryPolicy = defaults.repositoryPolicy;
this.resourceTags = defaults.resourceTags;
}
@CustomType.Setter
public Builder appliedFors(List appliedFors) {
if (appliedFors == null) {
throw new MissingRequiredPropertyException("GetRepositoryCreationTemplateResult", "appliedFors");
}
this.appliedFors = appliedFors;
return this;
}
public Builder appliedFors(String... appliedFors) {
return appliedFors(List.of(appliedFors));
}
@CustomType.Setter
public Builder customRoleArn(String customRoleArn) {
if (customRoleArn == null) {
throw new MissingRequiredPropertyException("GetRepositoryCreationTemplateResult", "customRoleArn");
}
this.customRoleArn = customRoleArn;
return this;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetRepositoryCreationTemplateResult", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder encryptionConfigurations(List encryptionConfigurations) {
if (encryptionConfigurations == null) {
throw new MissingRequiredPropertyException("GetRepositoryCreationTemplateResult", "encryptionConfigurations");
}
this.encryptionConfigurations = encryptionConfigurations;
return this;
}
public Builder encryptionConfigurations(GetRepositoryCreationTemplateEncryptionConfiguration... encryptionConfigurations) {
return encryptionConfigurations(List.of(encryptionConfigurations));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetRepositoryCreationTemplateResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder imageTagMutability(String imageTagMutability) {
if (imageTagMutability == null) {
throw new MissingRequiredPropertyException("GetRepositoryCreationTemplateResult", "imageTagMutability");
}
this.imageTagMutability = imageTagMutability;
return this;
}
@CustomType.Setter
public Builder lifecyclePolicy(String lifecyclePolicy) {
if (lifecyclePolicy == null) {
throw new MissingRequiredPropertyException("GetRepositoryCreationTemplateResult", "lifecyclePolicy");
}
this.lifecyclePolicy = lifecyclePolicy;
return this;
}
@CustomType.Setter
public Builder prefix(String prefix) {
if (prefix == null) {
throw new MissingRequiredPropertyException("GetRepositoryCreationTemplateResult", "prefix");
}
this.prefix = prefix;
return this;
}
@CustomType.Setter
public Builder registryId(String registryId) {
if (registryId == null) {
throw new MissingRequiredPropertyException("GetRepositoryCreationTemplateResult", "registryId");
}
this.registryId = registryId;
return this;
}
@CustomType.Setter
public Builder repositoryPolicy(String repositoryPolicy) {
if (repositoryPolicy == null) {
throw new MissingRequiredPropertyException("GetRepositoryCreationTemplateResult", "repositoryPolicy");
}
this.repositoryPolicy = repositoryPolicy;
return this;
}
@CustomType.Setter
public Builder resourceTags(Map resourceTags) {
if (resourceTags == null) {
throw new MissingRequiredPropertyException("GetRepositoryCreationTemplateResult", "resourceTags");
}
this.resourceTags = resourceTags;
return this;
}
public GetRepositoryCreationTemplateResult build() {
final var _resultValue = new GetRepositoryCreationTemplateResult();
_resultValue.appliedFors = appliedFors;
_resultValue.customRoleArn = customRoleArn;
_resultValue.description = description;
_resultValue.encryptionConfigurations = encryptionConfigurations;
_resultValue.id = id;
_resultValue.imageTagMutability = imageTagMutability;
_resultValue.lifecyclePolicy = lifecyclePolicy;
_resultValue.prefix = prefix;
_resultValue.registryId = registryId;
_resultValue.repositoryPolicy = repositoryPolicy;
_resultValue.resourceTags = resourceTags;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy