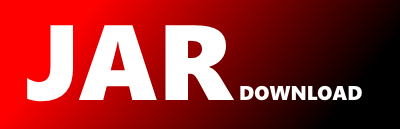
com.pulumi.aws.eks.NodeGroup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.eks;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.eks.NodeGroupArgs;
import com.pulumi.aws.eks.inputs.NodeGroupState;
import com.pulumi.aws.eks.outputs.NodeGroupLaunchTemplate;
import com.pulumi.aws.eks.outputs.NodeGroupRemoteAccess;
import com.pulumi.aws.eks.outputs.NodeGroupResource;
import com.pulumi.aws.eks.outputs.NodeGroupScalingConfig;
import com.pulumi.aws.eks.outputs.NodeGroupTaint;
import com.pulumi.aws.eks.outputs.NodeGroupUpdateConfig;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages an EKS Node Group, which can provision and optionally update an Auto Scaling Group of Kubernetes worker nodes compatible with EKS. Additional documentation about this functionality can be found in the [EKS User Guide](https://docs.aws.amazon.com/eks/latest/userguide/managed-node-groups.html).
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.eks.NodeGroup;
* import com.pulumi.aws.eks.NodeGroupArgs;
* import com.pulumi.aws.eks.inputs.NodeGroupScalingConfigArgs;
* import com.pulumi.aws.eks.inputs.NodeGroupUpdateConfigArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new NodeGroup("example", NodeGroupArgs.builder()
* .clusterName(exampleAwsEksCluster.name())
* .nodeGroupName("example")
* .nodeRoleArn(exampleAwsIamRole.arn())
* .subnetIds(exampleAwsSubnet.stream().map(element -> element.id()).collect(toList()))
* .scalingConfig(NodeGroupScalingConfigArgs.builder()
* .desiredSize(1)
* .maxSize(2)
* .minSize(1)
* .build())
* .updateConfig(NodeGroupUpdateConfigArgs.builder()
* .maxUnavailable(1)
* .build())
* .build(), CustomResourceOptions.builder()
* .dependsOn(
* example_AmazonEKSWorkerNodePolicy,
* example_AmazonEKSCNIPolicy,
* example_AmazonEC2ContainerRegistryReadOnly)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Ignoring Changes to Desired Size
*
* You can utilize [ignoreChanges](https://www.pulumi.com/docs/intro/concepts/programming-model/#ignorechanges) create an EKS Node Group with an initial size of running instances, then ignore any changes to that count caused externally (e.g. Application Autoscaling).
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.eks.NodeGroup;
* import com.pulumi.aws.eks.NodeGroupArgs;
* import com.pulumi.aws.eks.inputs.NodeGroupScalingConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new NodeGroup("example", NodeGroupArgs.builder()
* .scalingConfig(NodeGroupScalingConfigArgs.builder()
* .desiredSize(2)
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Example IAM Role for EKS Node Group
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.iam.RolePolicyAttachment;
* import com.pulumi.aws.iam.RolePolicyAttachmentArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Role("example", RoleArgs.builder()
* .name("eks-node-group-example")
* .assumeRolePolicy(serializeJson(
* jsonObject(
* jsonProperty("Statement", jsonArray(jsonObject(
* jsonProperty("Action", "sts:AssumeRole"),
* jsonProperty("Effect", "Allow"),
* jsonProperty("Principal", jsonObject(
* jsonProperty("Service", "ec2.amazonaws.com")
* ))
* ))),
* jsonProperty("Version", "2012-10-17")
* )))
* .build());
*
* var example_AmazonEKSWorkerNodePolicy = new RolePolicyAttachment("example-AmazonEKSWorkerNodePolicy", RolePolicyAttachmentArgs.builder()
* .policyArn("arn:aws:iam::aws:policy/AmazonEKSWorkerNodePolicy")
* .role(example.name())
* .build());
*
* var example_AmazonEKSCNIPolicy = new RolePolicyAttachment("example-AmazonEKSCNIPolicy", RolePolicyAttachmentArgs.builder()
* .policyArn("arn:aws:iam::aws:policy/AmazonEKS_CNI_Policy")
* .role(example.name())
* .build());
*
* var example_AmazonEC2ContainerRegistryReadOnly = new RolePolicyAttachment("example-AmazonEC2ContainerRegistryReadOnly", RolePolicyAttachmentArgs.builder()
* .policyArn("arn:aws:iam::aws:policy/AmazonEC2ContainerRegistryReadOnly")
* .role(example.name())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Example Subnets for EKS Node Group
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.AwsFunctions;
* import com.pulumi.aws.inputs.GetAvailabilityZonesArgs;
* import com.pulumi.aws.ec2.Subnet;
* import com.pulumi.aws.ec2.SubnetArgs;
* import com.pulumi.codegen.internal.KeyedValue;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var available = AwsFunctions.getAvailabilityZones(GetAvailabilityZonesArgs.builder()
* .state("available")
* .build());
*
* for (var i = 0; i < 2; i++) {
* new Subnet("example-" + i, SubnetArgs.builder()
* .availabilityZone(available.applyValue(getAvailabilityZonesResult -> getAvailabilityZonesResult.names())[range.value()])
* .cidrBlock(StdFunctions.cidrsubnet(CidrsubnetArgs.builder()
* .input(exampleAwsVpc.cidrBlock())
* .newbits(8)
* .netnum(range.value())
* .build()).result())
* .vpcId(exampleAwsVpc.id())
* .build());
*
*
* }
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import EKS Node Groups using the `cluster_name` and `node_group_name` separated by a colon (`:`). For example:
*
* ```sh
* $ pulumi import aws:eks/nodeGroup:NodeGroup my_node_group my_cluster:my_node_group
* ```
*
*/
@ResourceType(type="aws:eks/nodeGroup:NodeGroup")
public class NodeGroup extends com.pulumi.resources.CustomResource {
/**
* Type of Amazon Machine Image (AMI) associated with the EKS Node Group. See the [AWS documentation](https://docs.aws.amazon.com/eks/latest/APIReference/API_Nodegroup.html#AmazonEKS-Type-Nodegroup-amiType) for valid values. This provider will only perform drift detection if a configuration value is provided.
*
*/
@Export(name="amiType", refs={String.class}, tree="[0]")
private Output amiType;
/**
* @return Type of Amazon Machine Image (AMI) associated with the EKS Node Group. See the [AWS documentation](https://docs.aws.amazon.com/eks/latest/APIReference/API_Nodegroup.html#AmazonEKS-Type-Nodegroup-amiType) for valid values. This provider will only perform drift detection if a configuration value is provided.
*
*/
public Output amiType() {
return this.amiType;
}
/**
* Amazon Resource Name (ARN) of the EKS Node Group.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return Amazon Resource Name (ARN) of the EKS Node Group.
*
*/
public Output arn() {
return this.arn;
}
/**
* Type of capacity associated with the EKS Node Group. Valid values: `ON_DEMAND`, `SPOT`. This provider will only perform drift detection if a configuration value is provided.
*
*/
@Export(name="capacityType", refs={String.class}, tree="[0]")
private Output capacityType;
/**
* @return Type of capacity associated with the EKS Node Group. Valid values: `ON_DEMAND`, `SPOT`. This provider will only perform drift detection if a configuration value is provided.
*
*/
public Output capacityType() {
return this.capacityType;
}
/**
* Name of the EKS Cluster.
*
*/
@Export(name="clusterName", refs={String.class}, tree="[0]")
private Output clusterName;
/**
* @return Name of the EKS Cluster.
*
*/
public Output clusterName() {
return this.clusterName;
}
/**
* Disk size in GiB for worker nodes. Defaults to `50` for Windows, `20` all other node groups. The provider will only perform drift detection if a configuration value is provided.
*
*/
@Export(name="diskSize", refs={Integer.class}, tree="[0]")
private Output diskSize;
/**
* @return Disk size in GiB for worker nodes. Defaults to `50` for Windows, `20` all other node groups. The provider will only perform drift detection if a configuration value is provided.
*
*/
public Output diskSize() {
return this.diskSize;
}
/**
* Force version update if existing pods are unable to be drained due to a pod disruption budget issue.
*
*/
@Export(name="forceUpdateVersion", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> forceUpdateVersion;
/**
* @return Force version update if existing pods are unable to be drained due to a pod disruption budget issue.
*
*/
public Output> forceUpdateVersion() {
return Codegen.optional(this.forceUpdateVersion);
}
/**
* List of instance types associated with the EKS Node Group. Defaults to `["t3.medium"]`. The provider will only perform drift detection if a configuration value is provided.
*
*/
@Export(name="instanceTypes", refs={List.class,String.class}, tree="[0,1]")
private Output> instanceTypes;
/**
* @return List of instance types associated with the EKS Node Group. Defaults to `["t3.medium"]`. The provider will only perform drift detection if a configuration value is provided.
*
*/
public Output> instanceTypes() {
return this.instanceTypes;
}
/**
* Key-value map of Kubernetes labels. Only labels that are applied with the EKS API are managed by this argument. Other Kubernetes labels applied to the EKS Node Group will not be managed.
*
*/
@Export(name="labels", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> labels;
/**
* @return Key-value map of Kubernetes labels. Only labels that are applied with the EKS API are managed by this argument. Other Kubernetes labels applied to the EKS Node Group will not be managed.
*
*/
public Output>> labels() {
return Codegen.optional(this.labels);
}
/**
* Configuration block with Launch Template settings. See `launch_template` below for details. Conflicts with `remote_access`.
*
*/
@Export(name="launchTemplate", refs={NodeGroupLaunchTemplate.class}, tree="[0]")
private Output* @Nullable */ NodeGroupLaunchTemplate> launchTemplate;
/**
* @return Configuration block with Launch Template settings. See `launch_template` below for details. Conflicts with `remote_access`.
*
*/
public Output> launchTemplate() {
return Codegen.optional(this.launchTemplate);
}
/**
* Name of the EKS Node Group. If omitted, the provider will assign a random, unique name. Conflicts with `node_group_name_prefix`. The node group name can't be longer than 63 characters. It must start with a letter or digit, but can also include hyphens and underscores for the remaining characters.
*
*/
@Export(name="nodeGroupName", refs={String.class}, tree="[0]")
private Output nodeGroupName;
/**
* @return Name of the EKS Node Group. If omitted, the provider will assign a random, unique name. Conflicts with `node_group_name_prefix`. The node group name can't be longer than 63 characters. It must start with a letter or digit, but can also include hyphens and underscores for the remaining characters.
*
*/
public Output nodeGroupName() {
return this.nodeGroupName;
}
/**
* Creates a unique name beginning with the specified prefix. Conflicts with `node_group_name`.
*
*/
@Export(name="nodeGroupNamePrefix", refs={String.class}, tree="[0]")
private Output nodeGroupNamePrefix;
/**
* @return Creates a unique name beginning with the specified prefix. Conflicts with `node_group_name`.
*
*/
public Output nodeGroupNamePrefix() {
return this.nodeGroupNamePrefix;
}
/**
* Amazon Resource Name (ARN) of the IAM Role that provides permissions for the EKS Node Group.
*
*/
@Export(name="nodeRoleArn", refs={String.class}, tree="[0]")
private Output nodeRoleArn;
/**
* @return Amazon Resource Name (ARN) of the IAM Role that provides permissions for the EKS Node Group.
*
*/
public Output nodeRoleArn() {
return this.nodeRoleArn;
}
/**
* AMI version of the EKS Node Group. Defaults to latest version for Kubernetes version.
*
*/
@Export(name="releaseVersion", refs={String.class}, tree="[0]")
private Output releaseVersion;
/**
* @return AMI version of the EKS Node Group. Defaults to latest version for Kubernetes version.
*
*/
public Output releaseVersion() {
return this.releaseVersion;
}
/**
* Configuration block with remote access settings. See `remote_access` below for details. Conflicts with `launch_template`.
*
*/
@Export(name="remoteAccess", refs={NodeGroupRemoteAccess.class}, tree="[0]")
private Output* @Nullable */ NodeGroupRemoteAccess> remoteAccess;
/**
* @return Configuration block with remote access settings. See `remote_access` below for details. Conflicts with `launch_template`.
*
*/
public Output> remoteAccess() {
return Codegen.optional(this.remoteAccess);
}
/**
* List of objects containing information about underlying resources.
*
*/
@Export(name="resources", refs={List.class,NodeGroupResource.class}, tree="[0,1]")
private Output> resources;
/**
* @return List of objects containing information about underlying resources.
*
*/
public Output> resources() {
return this.resources;
}
/**
* Configuration block with scaling settings. See `scaling_config` below for details.
*
*/
@Export(name="scalingConfig", refs={NodeGroupScalingConfig.class}, tree="[0]")
private Output scalingConfig;
/**
* @return Configuration block with scaling settings. See `scaling_config` below for details.
*
*/
public Output scalingConfig() {
return this.scalingConfig;
}
/**
* Status of the EKS Node Group.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return Status of the EKS Node Group.
*
*/
public Output status() {
return this.status;
}
/**
* Identifiers of EC2 Subnets to associate with the EKS Node Group.
*
* The following arguments are optional:
*
*/
@Export(name="subnetIds", refs={List.class,String.class}, tree="[0,1]")
private Output> subnetIds;
/**
* @return Identifiers of EC2 Subnets to associate with the EKS Node Group.
*
* The following arguments are optional:
*
*/
public Output> subnetIds() {
return this.subnetIds;
}
/**
* Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy