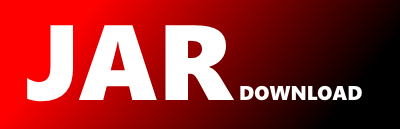
com.pulumi.aws.elasticache.outputs.GetReplicationGroupResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.elasticache.outputs;
import com.pulumi.aws.elasticache.outputs.GetReplicationGroupLogDeliveryConfiguration;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetReplicationGroupResult {
/**
* @return ARN of the created ElastiCache Replication Group.
*
*/
private String arn;
/**
* @return Whether an AuthToken (password) is enabled.
*
*/
private Boolean authTokenEnabled;
/**
* @return A flag whether a read-only replica will be automatically promoted to read/write primary if the existing primary fails.
*
*/
private Boolean automaticFailoverEnabled;
/**
* @return Whether cluster mode is enabled or disabled.
*
*/
private String clusterMode;
/**
* @return The configuration endpoint address to allow host discovery.
*
*/
private String configurationEndpointAddress;
/**
* @return Description of the replication group.
*
*/
private String description;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return Redis [SLOWLOG](https://redis.io/commands/slowlog) or Redis [Engine Log](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/Log_Delivery.html#Log_contents-engine-log) delivery settings.
*
*/
private List logDeliveryConfigurations;
/**
* @return Identifiers of all the nodes that are part of this replication group.
*
*/
private List memberClusters;
/**
* @return Whether Multi-AZ Support is enabled for the replication group.
*
*/
private Boolean multiAzEnabled;
/**
* @return The cluster node type.
*
*/
private String nodeType;
/**
* @return The number of cache clusters that the replication group has.
*
*/
private Integer numCacheClusters;
/**
* @return Number of node groups (shards) for the replication group.
*
*/
private Integer numNodeGroups;
/**
* @return The port number on which the configuration endpoint will accept connections.
*
*/
private Integer port;
/**
* @return The endpoint of the primary node in this node group (shard).
*
*/
private String primaryEndpointAddress;
/**
* @return The endpoint of the reader node in this node group (shard).
*
*/
private String readerEndpointAddress;
/**
* @return Number of replica nodes in each node group.
*
*/
private Integer replicasPerNodeGroup;
private String replicationGroupId;
/**
* @return The number of days for which ElastiCache retains automatic cache cluster snapshots before deleting them.
*
*/
private Integer snapshotRetentionLimit;
/**
* @return Daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group (shard).
*
*/
private String snapshotWindow;
private GetReplicationGroupResult() {}
/**
* @return ARN of the created ElastiCache Replication Group.
*
*/
public String arn() {
return this.arn;
}
/**
* @return Whether an AuthToken (password) is enabled.
*
*/
public Boolean authTokenEnabled() {
return this.authTokenEnabled;
}
/**
* @return A flag whether a read-only replica will be automatically promoted to read/write primary if the existing primary fails.
*
*/
public Boolean automaticFailoverEnabled() {
return this.automaticFailoverEnabled;
}
/**
* @return Whether cluster mode is enabled or disabled.
*
*/
public String clusterMode() {
return this.clusterMode;
}
/**
* @return The configuration endpoint address to allow host discovery.
*
*/
public String configurationEndpointAddress() {
return this.configurationEndpointAddress;
}
/**
* @return Description of the replication group.
*
*/
public String description() {
return this.description;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Redis [SLOWLOG](https://redis.io/commands/slowlog) or Redis [Engine Log](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/Log_Delivery.html#Log_contents-engine-log) delivery settings.
*
*/
public List logDeliveryConfigurations() {
return this.logDeliveryConfigurations;
}
/**
* @return Identifiers of all the nodes that are part of this replication group.
*
*/
public List memberClusters() {
return this.memberClusters;
}
/**
* @return Whether Multi-AZ Support is enabled for the replication group.
*
*/
public Boolean multiAzEnabled() {
return this.multiAzEnabled;
}
/**
* @return The cluster node type.
*
*/
public String nodeType() {
return this.nodeType;
}
/**
* @return The number of cache clusters that the replication group has.
*
*/
public Integer numCacheClusters() {
return this.numCacheClusters;
}
/**
* @return Number of node groups (shards) for the replication group.
*
*/
public Integer numNodeGroups() {
return this.numNodeGroups;
}
/**
* @return The port number on which the configuration endpoint will accept connections.
*
*/
public Integer port() {
return this.port;
}
/**
* @return The endpoint of the primary node in this node group (shard).
*
*/
public String primaryEndpointAddress() {
return this.primaryEndpointAddress;
}
/**
* @return The endpoint of the reader node in this node group (shard).
*
*/
public String readerEndpointAddress() {
return this.readerEndpointAddress;
}
/**
* @return Number of replica nodes in each node group.
*
*/
public Integer replicasPerNodeGroup() {
return this.replicasPerNodeGroup;
}
public String replicationGroupId() {
return this.replicationGroupId;
}
/**
* @return The number of days for which ElastiCache retains automatic cache cluster snapshots before deleting them.
*
*/
public Integer snapshotRetentionLimit() {
return this.snapshotRetentionLimit;
}
/**
* @return Daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group (shard).
*
*/
public String snapshotWindow() {
return this.snapshotWindow;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetReplicationGroupResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private Boolean authTokenEnabled;
private Boolean automaticFailoverEnabled;
private String clusterMode;
private String configurationEndpointAddress;
private String description;
private String id;
private List logDeliveryConfigurations;
private List memberClusters;
private Boolean multiAzEnabled;
private String nodeType;
private Integer numCacheClusters;
private Integer numNodeGroups;
private Integer port;
private String primaryEndpointAddress;
private String readerEndpointAddress;
private Integer replicasPerNodeGroup;
private String replicationGroupId;
private Integer snapshotRetentionLimit;
private String snapshotWindow;
public Builder() {}
public Builder(GetReplicationGroupResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.authTokenEnabled = defaults.authTokenEnabled;
this.automaticFailoverEnabled = defaults.automaticFailoverEnabled;
this.clusterMode = defaults.clusterMode;
this.configurationEndpointAddress = defaults.configurationEndpointAddress;
this.description = defaults.description;
this.id = defaults.id;
this.logDeliveryConfigurations = defaults.logDeliveryConfigurations;
this.memberClusters = defaults.memberClusters;
this.multiAzEnabled = defaults.multiAzEnabled;
this.nodeType = defaults.nodeType;
this.numCacheClusters = defaults.numCacheClusters;
this.numNodeGroups = defaults.numNodeGroups;
this.port = defaults.port;
this.primaryEndpointAddress = defaults.primaryEndpointAddress;
this.readerEndpointAddress = defaults.readerEndpointAddress;
this.replicasPerNodeGroup = defaults.replicasPerNodeGroup;
this.replicationGroupId = defaults.replicationGroupId;
this.snapshotRetentionLimit = defaults.snapshotRetentionLimit;
this.snapshotWindow = defaults.snapshotWindow;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder authTokenEnabled(Boolean authTokenEnabled) {
if (authTokenEnabled == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "authTokenEnabled");
}
this.authTokenEnabled = authTokenEnabled;
return this;
}
@CustomType.Setter
public Builder automaticFailoverEnabled(Boolean automaticFailoverEnabled) {
if (automaticFailoverEnabled == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "automaticFailoverEnabled");
}
this.automaticFailoverEnabled = automaticFailoverEnabled;
return this;
}
@CustomType.Setter
public Builder clusterMode(String clusterMode) {
if (clusterMode == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "clusterMode");
}
this.clusterMode = clusterMode;
return this;
}
@CustomType.Setter
public Builder configurationEndpointAddress(String configurationEndpointAddress) {
if (configurationEndpointAddress == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "configurationEndpointAddress");
}
this.configurationEndpointAddress = configurationEndpointAddress;
return this;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder logDeliveryConfigurations(List logDeliveryConfigurations) {
if (logDeliveryConfigurations == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "logDeliveryConfigurations");
}
this.logDeliveryConfigurations = logDeliveryConfigurations;
return this;
}
public Builder logDeliveryConfigurations(GetReplicationGroupLogDeliveryConfiguration... logDeliveryConfigurations) {
return logDeliveryConfigurations(List.of(logDeliveryConfigurations));
}
@CustomType.Setter
public Builder memberClusters(List memberClusters) {
if (memberClusters == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "memberClusters");
}
this.memberClusters = memberClusters;
return this;
}
public Builder memberClusters(String... memberClusters) {
return memberClusters(List.of(memberClusters));
}
@CustomType.Setter
public Builder multiAzEnabled(Boolean multiAzEnabled) {
if (multiAzEnabled == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "multiAzEnabled");
}
this.multiAzEnabled = multiAzEnabled;
return this;
}
@CustomType.Setter
public Builder nodeType(String nodeType) {
if (nodeType == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "nodeType");
}
this.nodeType = nodeType;
return this;
}
@CustomType.Setter
public Builder numCacheClusters(Integer numCacheClusters) {
if (numCacheClusters == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "numCacheClusters");
}
this.numCacheClusters = numCacheClusters;
return this;
}
@CustomType.Setter
public Builder numNodeGroups(Integer numNodeGroups) {
if (numNodeGroups == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "numNodeGroups");
}
this.numNodeGroups = numNodeGroups;
return this;
}
@CustomType.Setter
public Builder port(Integer port) {
if (port == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "port");
}
this.port = port;
return this;
}
@CustomType.Setter
public Builder primaryEndpointAddress(String primaryEndpointAddress) {
if (primaryEndpointAddress == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "primaryEndpointAddress");
}
this.primaryEndpointAddress = primaryEndpointAddress;
return this;
}
@CustomType.Setter
public Builder readerEndpointAddress(String readerEndpointAddress) {
if (readerEndpointAddress == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "readerEndpointAddress");
}
this.readerEndpointAddress = readerEndpointAddress;
return this;
}
@CustomType.Setter
public Builder replicasPerNodeGroup(Integer replicasPerNodeGroup) {
if (replicasPerNodeGroup == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "replicasPerNodeGroup");
}
this.replicasPerNodeGroup = replicasPerNodeGroup;
return this;
}
@CustomType.Setter
public Builder replicationGroupId(String replicationGroupId) {
if (replicationGroupId == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "replicationGroupId");
}
this.replicationGroupId = replicationGroupId;
return this;
}
@CustomType.Setter
public Builder snapshotRetentionLimit(Integer snapshotRetentionLimit) {
if (snapshotRetentionLimit == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "snapshotRetentionLimit");
}
this.snapshotRetentionLimit = snapshotRetentionLimit;
return this;
}
@CustomType.Setter
public Builder snapshotWindow(String snapshotWindow) {
if (snapshotWindow == null) {
throw new MissingRequiredPropertyException("GetReplicationGroupResult", "snapshotWindow");
}
this.snapshotWindow = snapshotWindow;
return this;
}
public GetReplicationGroupResult build() {
final var _resultValue = new GetReplicationGroupResult();
_resultValue.arn = arn;
_resultValue.authTokenEnabled = authTokenEnabled;
_resultValue.automaticFailoverEnabled = automaticFailoverEnabled;
_resultValue.clusterMode = clusterMode;
_resultValue.configurationEndpointAddress = configurationEndpointAddress;
_resultValue.description = description;
_resultValue.id = id;
_resultValue.logDeliveryConfigurations = logDeliveryConfigurations;
_resultValue.memberClusters = memberClusters;
_resultValue.multiAzEnabled = multiAzEnabled;
_resultValue.nodeType = nodeType;
_resultValue.numCacheClusters = numCacheClusters;
_resultValue.numNodeGroups = numNodeGroups;
_resultValue.port = port;
_resultValue.primaryEndpointAddress = primaryEndpointAddress;
_resultValue.readerEndpointAddress = readerEndpointAddress;
_resultValue.replicasPerNodeGroup = replicasPerNodeGroup;
_resultValue.replicationGroupId = replicationGroupId;
_resultValue.snapshotRetentionLimit = snapshotRetentionLimit;
_resultValue.snapshotWindow = snapshotWindow;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy