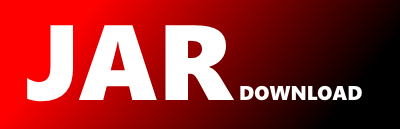
com.pulumi.aws.elasticbeanstalk.inputs.EnvironmentState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.elasticbeanstalk.inputs;
import com.pulumi.aws.elasticbeanstalk.inputs.EnvironmentAllSettingArgs;
import com.pulumi.aws.elasticbeanstalk.inputs.EnvironmentSettingArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class EnvironmentState extends com.pulumi.resources.ResourceArgs {
public static final EnvironmentState Empty = new EnvironmentState();
/**
* List of all option settings configured in this Environment. These
* are a combination of default settings and their overrides from `setting` in
* the configuration.
*
*/
@Import(name="allSettings")
private @Nullable Output> allSettings;
/**
* @return List of all option settings configured in this Environment. These
* are a combination of default settings and their overrides from `setting` in
* the configuration.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy