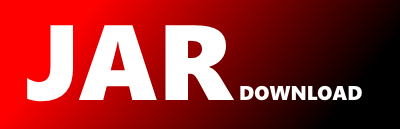
com.pulumi.aws.elasticsearch.outputs.GetDomainClusterConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.elasticsearch.outputs;
import com.pulumi.aws.elasticsearch.outputs.GetDomainClusterConfigColdStorageOption;
import com.pulumi.aws.elasticsearch.outputs.GetDomainClusterConfigZoneAwarenessConfig;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetDomainClusterConfig {
/**
* @return Configuration block containing cold storage configuration.
*
*/
private List coldStorageOptions;
/**
* @return Number of dedicated master nodes in the cluster.
*
*/
private Integer dedicatedMasterCount;
/**
* @return Indicates whether dedicated master nodes are enabled for the cluster.
*
*/
private Boolean dedicatedMasterEnabled;
/**
* @return Instance type of the dedicated master nodes in the cluster.
*
*/
private String dedicatedMasterType;
/**
* @return Number of instances in the cluster.
*
*/
private Integer instanceCount;
/**
* @return Instance type of data nodes in the cluster.
*
*/
private String instanceType;
/**
* @return The number of warm nodes in the cluster.
*
*/
private Integer warmCount;
/**
* @return Warm storage is enabled.
*
*/
private Boolean warmEnabled;
/**
* @return The instance type for the Elasticsearch cluster's warm nodes.
*
*/
private String warmType;
/**
* @return Configuration block containing zone awareness settings.
*
*/
private List zoneAwarenessConfigs;
/**
* @return Indicates whether zone awareness is enabled.
*
*/
private Boolean zoneAwarenessEnabled;
private GetDomainClusterConfig() {}
/**
* @return Configuration block containing cold storage configuration.
*
*/
public List coldStorageOptions() {
return this.coldStorageOptions;
}
/**
* @return Number of dedicated master nodes in the cluster.
*
*/
public Integer dedicatedMasterCount() {
return this.dedicatedMasterCount;
}
/**
* @return Indicates whether dedicated master nodes are enabled for the cluster.
*
*/
public Boolean dedicatedMasterEnabled() {
return this.dedicatedMasterEnabled;
}
/**
* @return Instance type of the dedicated master nodes in the cluster.
*
*/
public String dedicatedMasterType() {
return this.dedicatedMasterType;
}
/**
* @return Number of instances in the cluster.
*
*/
public Integer instanceCount() {
return this.instanceCount;
}
/**
* @return Instance type of data nodes in the cluster.
*
*/
public String instanceType() {
return this.instanceType;
}
/**
* @return The number of warm nodes in the cluster.
*
*/
public Integer warmCount() {
return this.warmCount;
}
/**
* @return Warm storage is enabled.
*
*/
public Boolean warmEnabled() {
return this.warmEnabled;
}
/**
* @return The instance type for the Elasticsearch cluster's warm nodes.
*
*/
public String warmType() {
return this.warmType;
}
/**
* @return Configuration block containing zone awareness settings.
*
*/
public List zoneAwarenessConfigs() {
return this.zoneAwarenessConfigs;
}
/**
* @return Indicates whether zone awareness is enabled.
*
*/
public Boolean zoneAwarenessEnabled() {
return this.zoneAwarenessEnabled;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDomainClusterConfig defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List coldStorageOptions;
private Integer dedicatedMasterCount;
private Boolean dedicatedMasterEnabled;
private String dedicatedMasterType;
private Integer instanceCount;
private String instanceType;
private Integer warmCount;
private Boolean warmEnabled;
private String warmType;
private List zoneAwarenessConfigs;
private Boolean zoneAwarenessEnabled;
public Builder() {}
public Builder(GetDomainClusterConfig defaults) {
Objects.requireNonNull(defaults);
this.coldStorageOptions = defaults.coldStorageOptions;
this.dedicatedMasterCount = defaults.dedicatedMasterCount;
this.dedicatedMasterEnabled = defaults.dedicatedMasterEnabled;
this.dedicatedMasterType = defaults.dedicatedMasterType;
this.instanceCount = defaults.instanceCount;
this.instanceType = defaults.instanceType;
this.warmCount = defaults.warmCount;
this.warmEnabled = defaults.warmEnabled;
this.warmType = defaults.warmType;
this.zoneAwarenessConfigs = defaults.zoneAwarenessConfigs;
this.zoneAwarenessEnabled = defaults.zoneAwarenessEnabled;
}
@CustomType.Setter
public Builder coldStorageOptions(List coldStorageOptions) {
if (coldStorageOptions == null) {
throw new MissingRequiredPropertyException("GetDomainClusterConfig", "coldStorageOptions");
}
this.coldStorageOptions = coldStorageOptions;
return this;
}
public Builder coldStorageOptions(GetDomainClusterConfigColdStorageOption... coldStorageOptions) {
return coldStorageOptions(List.of(coldStorageOptions));
}
@CustomType.Setter
public Builder dedicatedMasterCount(Integer dedicatedMasterCount) {
if (dedicatedMasterCount == null) {
throw new MissingRequiredPropertyException("GetDomainClusterConfig", "dedicatedMasterCount");
}
this.dedicatedMasterCount = dedicatedMasterCount;
return this;
}
@CustomType.Setter
public Builder dedicatedMasterEnabled(Boolean dedicatedMasterEnabled) {
if (dedicatedMasterEnabled == null) {
throw new MissingRequiredPropertyException("GetDomainClusterConfig", "dedicatedMasterEnabled");
}
this.dedicatedMasterEnabled = dedicatedMasterEnabled;
return this;
}
@CustomType.Setter
public Builder dedicatedMasterType(String dedicatedMasterType) {
if (dedicatedMasterType == null) {
throw new MissingRequiredPropertyException("GetDomainClusterConfig", "dedicatedMasterType");
}
this.dedicatedMasterType = dedicatedMasterType;
return this;
}
@CustomType.Setter
public Builder instanceCount(Integer instanceCount) {
if (instanceCount == null) {
throw new MissingRequiredPropertyException("GetDomainClusterConfig", "instanceCount");
}
this.instanceCount = instanceCount;
return this;
}
@CustomType.Setter
public Builder instanceType(String instanceType) {
if (instanceType == null) {
throw new MissingRequiredPropertyException("GetDomainClusterConfig", "instanceType");
}
this.instanceType = instanceType;
return this;
}
@CustomType.Setter
public Builder warmCount(Integer warmCount) {
if (warmCount == null) {
throw new MissingRequiredPropertyException("GetDomainClusterConfig", "warmCount");
}
this.warmCount = warmCount;
return this;
}
@CustomType.Setter
public Builder warmEnabled(Boolean warmEnabled) {
if (warmEnabled == null) {
throw new MissingRequiredPropertyException("GetDomainClusterConfig", "warmEnabled");
}
this.warmEnabled = warmEnabled;
return this;
}
@CustomType.Setter
public Builder warmType(String warmType) {
if (warmType == null) {
throw new MissingRequiredPropertyException("GetDomainClusterConfig", "warmType");
}
this.warmType = warmType;
return this;
}
@CustomType.Setter
public Builder zoneAwarenessConfigs(List zoneAwarenessConfigs) {
if (zoneAwarenessConfigs == null) {
throw new MissingRequiredPropertyException("GetDomainClusterConfig", "zoneAwarenessConfigs");
}
this.zoneAwarenessConfigs = zoneAwarenessConfigs;
return this;
}
public Builder zoneAwarenessConfigs(GetDomainClusterConfigZoneAwarenessConfig... zoneAwarenessConfigs) {
return zoneAwarenessConfigs(List.of(zoneAwarenessConfigs));
}
@CustomType.Setter
public Builder zoneAwarenessEnabled(Boolean zoneAwarenessEnabled) {
if (zoneAwarenessEnabled == null) {
throw new MissingRequiredPropertyException("GetDomainClusterConfig", "zoneAwarenessEnabled");
}
this.zoneAwarenessEnabled = zoneAwarenessEnabled;
return this;
}
public GetDomainClusterConfig build() {
final var _resultValue = new GetDomainClusterConfig();
_resultValue.coldStorageOptions = coldStorageOptions;
_resultValue.dedicatedMasterCount = dedicatedMasterCount;
_resultValue.dedicatedMasterEnabled = dedicatedMasterEnabled;
_resultValue.dedicatedMasterType = dedicatedMasterType;
_resultValue.instanceCount = instanceCount;
_resultValue.instanceType = instanceType;
_resultValue.warmCount = warmCount;
_resultValue.warmEnabled = warmEnabled;
_resultValue.warmType = warmType;
_resultValue.zoneAwarenessConfigs = zoneAwarenessConfigs;
_resultValue.zoneAwarenessEnabled = zoneAwarenessEnabled;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy