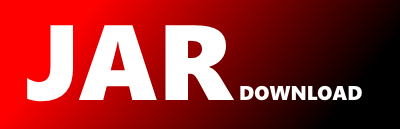
com.pulumi.aws.emr.StudioArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.emr;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class StudioArgs extends com.pulumi.resources.ResourceArgs {
public static final StudioArgs Empty = new StudioArgs();
/**
* Specifies whether the Studio authenticates users using IAM or Amazon Web Services SSO. Valid values are `SSO` or `IAM`.
*
*/
@Import(name="authMode", required=true)
private Output authMode;
/**
* @return Specifies whether the Studio authenticates users using IAM or Amazon Web Services SSO. Valid values are `SSO` or `IAM`.
*
*/
public Output authMode() {
return this.authMode;
}
/**
* The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
*
*/
@Import(name="defaultS3Location", required=true)
private Output defaultS3Location;
/**
* @return The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
*
*/
public Output defaultS3Location() {
return this.defaultS3Location;
}
/**
* A detailed description of the Amazon EMR Studio.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return A detailed description of the Amazon EMR Studio.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy