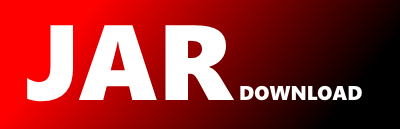
com.pulumi.aws.finspace.KxEnvironment Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.finspace;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.finspace.KxEnvironmentArgs;
import com.pulumi.aws.finspace.inputs.KxEnvironmentState;
import com.pulumi.aws.finspace.outputs.KxEnvironmentCustomDnsConfiguration;
import com.pulumi.aws.finspace.outputs.KxEnvironmentTransitGatewayConfiguration;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Resource for managing an AWS FinSpace Kx Environment.
*
* ## Example Usage
*
* ### Basic Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kms.Key;
* import com.pulumi.aws.kms.KeyArgs;
* import com.pulumi.aws.finspace.KxEnvironment;
* import com.pulumi.aws.finspace.KxEnvironmentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Key("example", KeyArgs.builder()
* .description("Sample KMS Key")
* .deletionWindowInDays(7)
* .build());
*
* var exampleKxEnvironment = new KxEnvironment("exampleKxEnvironment", KxEnvironmentArgs.builder()
* .name("my-tf-kx-environment")
* .kmsKeyId(example.arn())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### With Transit Gateway Configuration
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kms.Key;
* import com.pulumi.aws.kms.KeyArgs;
* import com.pulumi.aws.ec2transitgateway.TransitGateway;
* import com.pulumi.aws.ec2transitgateway.TransitGatewayArgs;
* import com.pulumi.aws.finspace.KxEnvironment;
* import com.pulumi.aws.finspace.KxEnvironmentArgs;
* import com.pulumi.aws.finspace.inputs.KxEnvironmentTransitGatewayConfigurationArgs;
* import com.pulumi.aws.finspace.inputs.KxEnvironmentCustomDnsConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Key("example", KeyArgs.builder()
* .description("Sample KMS Key")
* .deletionWindowInDays(7)
* .build());
*
* var exampleTransitGateway = new TransitGateway("exampleTransitGateway", TransitGatewayArgs.builder()
* .description("example")
* .build());
*
* var exampleEnv = new KxEnvironment("exampleEnv", KxEnvironmentArgs.builder()
* .name("my-tf-kx-environment")
* .description("Environment description")
* .kmsKeyId(example.arn())
* .transitGatewayConfiguration(KxEnvironmentTransitGatewayConfigurationArgs.builder()
* .transitGatewayId(exampleTransitGateway.id())
* .routableCidrSpace("100.64.0.0/26")
* .build())
* .customDnsConfigurations(KxEnvironmentCustomDnsConfigurationArgs.builder()
* .customDnsServerName("example.finspace.amazonaws.com")
* .customDnsServerIp("10.0.0.76")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### With Transit Gateway Attachment Network ACL Configuration
*
* <!--Start PulumiCodeChooser -->
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import an AWS FinSpace Kx Environment using the `id`. For example:
*
* ```sh
* $ pulumi import aws:finspace/kxEnvironment:KxEnvironment example n3ceo7wqxoxcti5tujqwzs
* ```
*
*/
@ResourceType(type="aws:finspace/kxEnvironment:KxEnvironment")
public class KxEnvironment extends com.pulumi.resources.CustomResource {
/**
* Amazon Resource Name (ARN) identifier of the KX environment.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return Amazon Resource Name (ARN) identifier of the KX environment.
*
*/
public Output arn() {
return this.arn;
}
/**
* AWS Availability Zone IDs that this environment is available in. Important when selecting VPC subnets to use in cluster creation.
*
*/
@Export(name="availabilityZones", refs={List.class,String.class}, tree="[0,1]")
private Output> availabilityZones;
/**
* @return AWS Availability Zone IDs that this environment is available in. Important when selecting VPC subnets to use in cluster creation.
*
*/
public Output> availabilityZones() {
return this.availabilityZones;
}
/**
* Timestamp at which the environment is created in FinSpace. Value determined as epoch time in seconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000.
*
*/
@Export(name="createdTimestamp", refs={String.class}, tree="[0]")
private Output createdTimestamp;
/**
* @return Timestamp at which the environment is created in FinSpace. Value determined as epoch time in seconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000.
*
*/
public Output createdTimestamp() {
return this.createdTimestamp;
}
/**
* List of DNS server name and server IP. This is used to set up Route-53 outbound resolvers. Defined below.
*
*/
@Export(name="customDnsConfigurations", refs={List.class,KxEnvironmentCustomDnsConfiguration.class}, tree="[0,1]")
private Output* @Nullable */ List> customDnsConfigurations;
/**
* @return List of DNS server name and server IP. This is used to set up Route-53 outbound resolvers. Defined below.
*
*/
public Output>> customDnsConfigurations() {
return Codegen.optional(this.customDnsConfigurations);
}
/**
* Description for the KX environment.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Description for the KX environment.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* Unique identifier for the AWS environment infrastructure account.
*
*/
@Export(name="infrastructureAccountId", refs={String.class}, tree="[0]")
private Output infrastructureAccountId;
/**
* @return Unique identifier for the AWS environment infrastructure account.
*
*/
public Output infrastructureAccountId() {
return this.infrastructureAccountId;
}
/**
* KMS key ID to encrypt your data in the FinSpace environment.
*
* The following arguments are optional:
*
*/
@Export(name="kmsKeyId", refs={String.class}, tree="[0]")
private Output kmsKeyId;
/**
* @return KMS key ID to encrypt your data in the FinSpace environment.
*
* The following arguments are optional:
*
*/
public Output kmsKeyId() {
return this.kmsKeyId;
}
/**
* Last timestamp at which the environment was updated in FinSpace. Value determined as epoch time in seconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000.
*
*/
@Export(name="lastModifiedTimestamp", refs={String.class}, tree="[0]")
private Output lastModifiedTimestamp;
/**
* @return Last timestamp at which the environment was updated in FinSpace. Value determined as epoch time in seconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000.
*
*/
public Output lastModifiedTimestamp() {
return this.lastModifiedTimestamp;
}
/**
* Name of the KX environment that you want to create.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name of the KX environment that you want to create.
*
*/
public Output name() {
return this.name;
}
/**
* Status of environment creation
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return Status of environment creation
*
*/
public Output status() {
return this.status;
}
/**
* Key-value mapping of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value mapping of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy