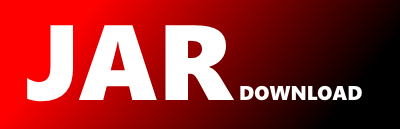
com.pulumi.aws.glue.TriggerArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.glue;
import com.pulumi.aws.glue.inputs.TriggerActionArgs;
import com.pulumi.aws.glue.inputs.TriggerEventBatchingConditionArgs;
import com.pulumi.aws.glue.inputs.TriggerPredicateArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class TriggerArgs extends com.pulumi.resources.ResourceArgs {
public static final TriggerArgs Empty = new TriggerArgs();
/**
* List of actions initiated by this trigger when it fires. See Actions Below.
*
*/
@Import(name="actions", required=true)
private Output> actions;
/**
* @return List of actions initiated by this trigger when it fires. See Actions Below.
*
*/
public Output> actions() {
return this.actions;
}
/**
* A description of the new trigger.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return A description of the new trigger.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy