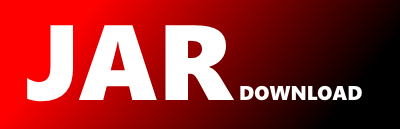
com.pulumi.aws.iam.AccessKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.iam;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.iam.AccessKeyArgs;
import com.pulumi.aws.iam.inputs.AccessKeyState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an IAM access key. This is a set of credentials that allow API requests to be made as an IAM user.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.User;
* import com.pulumi.aws.iam.UserArgs;
* import com.pulumi.aws.iam.AccessKey;
* import com.pulumi.aws.iam.AccessKeyArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.UserPolicy;
* import com.pulumi.aws.iam.UserPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var lbUser = new User("lbUser", UserArgs.builder()
* .name("loadbalancer")
* .path("/system/")
* .build());
*
* var lb = new AccessKey("lb", AccessKeyArgs.builder()
* .user(lbUser.name())
* .pgpKey("keybase:some_person_that_exists")
* .build());
*
* final var lbRo = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions("ec2:Describe*")
* .resources("*")
* .build())
* .build());
*
* var lbRoUserPolicy = new UserPolicy("lbRoUserPolicy", UserPolicyArgs.builder()
* .name("test")
* .user(lbUser.name())
* .policy(lbRo.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
*
* ctx.export("secret", lb.encryptedSecret());
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.User;
* import com.pulumi.aws.iam.UserArgs;
* import com.pulumi.aws.iam.AccessKey;
* import com.pulumi.aws.iam.AccessKeyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var test = new User("test", UserArgs.builder()
* .name("test")
* .path("/test/")
* .build());
*
* var testAccessKey = new AccessKey("testAccessKey", AccessKeyArgs.builder()
* .user(test.name())
* .build());
*
* ctx.export("awsIamSmtpPasswordV4", testAccessKey.sesSmtpPasswordV4());
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import IAM Access Keys using the identifier. For example:
*
* ```sh
* $ pulumi import aws:iam/accessKey:AccessKey example AKIA1234567890
* ```
* Resource attributes such as `encrypted_secret`, `key_fingerprint`, `pgp_key`, `secret`, `ses_smtp_password_v4`, and `encrypted_ses_smtp_password_v4` are not available for imported resources as this information cannot be read from the IAM API.
*
*/
@ResourceType(type="aws:iam/accessKey:AccessKey")
public class AccessKey extends com.pulumi.resources.CustomResource {
/**
* Date and time in [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8) that the access key was created.
*
*/
@Export(name="createDate", refs={String.class}, tree="[0]")
private Output createDate;
/**
* @return Date and time in [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8) that the access key was created.
*
*/
public Output createDate() {
return this.createDate;
}
/**
* Encrypted secret, base64 encoded, if `pgp_key` was specified. This attribute is not available for imported resources. The encrypted secret may be decrypted using the command line.
*
*/
@Export(name="encryptedSecret", refs={String.class}, tree="[0]")
private Output encryptedSecret;
/**
* @return Encrypted secret, base64 encoded, if `pgp_key` was specified. This attribute is not available for imported resources. The encrypted secret may be decrypted using the command line.
*
*/
public Output encryptedSecret() {
return this.encryptedSecret;
}
/**
* Encrypted SES SMTP password, base64 encoded, if `pgp_key` was specified. This attribute is not available for imported resources. The encrypted password may be decrypted using the command line.
*
*/
@Export(name="encryptedSesSmtpPasswordV4", refs={String.class}, tree="[0]")
private Output encryptedSesSmtpPasswordV4;
/**
* @return Encrypted SES SMTP password, base64 encoded, if `pgp_key` was specified. This attribute is not available for imported resources. The encrypted password may be decrypted using the command line.
*
*/
public Output encryptedSesSmtpPasswordV4() {
return this.encryptedSesSmtpPasswordV4;
}
/**
* Fingerprint of the PGP key used to encrypt the secret. This attribute is not available for imported resources.
*
*/
@Export(name="keyFingerprint", refs={String.class}, tree="[0]")
private Output keyFingerprint;
/**
* @return Fingerprint of the PGP key used to encrypt the secret. This attribute is not available for imported resources.
*
*/
public Output keyFingerprint() {
return this.keyFingerprint;
}
/**
* Either a base-64 encoded PGP public key, or a keybase username in the form `keybase:some_person_that_exists`, for use in the `encrypted_secret` output attribute. If providing a base-64 encoded PGP public key, make sure to provide the "raw" version and not the "armored" one (e.g. avoid passing the `-a` option to `gpg --export`).
*
*/
@Export(name="pgpKey", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> pgpKey;
/**
* @return Either a base-64 encoded PGP public key, or a keybase username in the form `keybase:some_person_that_exists`, for use in the `encrypted_secret` output attribute. If providing a base-64 encoded PGP public key, make sure to provide the "raw" version and not the "armored" one (e.g. avoid passing the `-a` option to `gpg --export`).
*
*/
public Output> pgpKey() {
return Codegen.optional(this.pgpKey);
}
/**
* Secret access key. This attribute is not available for imported resources. Note that this will be written to the state file. If you use this, please protect your backend state file judiciously. Alternatively, you may supply a `pgp_key` instead, which will prevent the secret from being stored in plaintext, at the cost of preventing the use of the secret key in automation.
*
*/
@Export(name="secret", refs={String.class}, tree="[0]")
private Output secret;
/**
* @return Secret access key. This attribute is not available for imported resources. Note that this will be written to the state file. If you use this, please protect your backend state file judiciously. Alternatively, you may supply a `pgp_key` instead, which will prevent the secret from being stored in plaintext, at the cost of preventing the use of the secret key in automation.
*
*/
public Output secret() {
return this.secret;
}
/**
* Secret access key converted into an SES SMTP password by applying [AWS's documented Sigv4 conversion algorithm](https://docs.aws.amazon.com/ses/latest/DeveloperGuide/smtp-credentials.html#smtp-credentials-convert). This attribute is not available for imported resources. As SigV4 is region specific, valid Provider regions are `ap-south-1`, `ap-southeast-2`, `eu-central-1`, `eu-west-1`, `us-east-1` and `us-west-2`. See current [AWS SES regions](https://docs.aws.amazon.com/general/latest/gr/rande.html#ses_region).
*
*/
@Export(name="sesSmtpPasswordV4", refs={String.class}, tree="[0]")
private Output sesSmtpPasswordV4;
/**
* @return Secret access key converted into an SES SMTP password by applying [AWS's documented Sigv4 conversion algorithm](https://docs.aws.amazon.com/ses/latest/DeveloperGuide/smtp-credentials.html#smtp-credentials-convert). This attribute is not available for imported resources. As SigV4 is region specific, valid Provider regions are `ap-south-1`, `ap-southeast-2`, `eu-central-1`, `eu-west-1`, `us-east-1` and `us-west-2`. See current [AWS SES regions](https://docs.aws.amazon.com/general/latest/gr/rande.html#ses_region).
*
*/
public Output sesSmtpPasswordV4() {
return this.sesSmtpPasswordV4;
}
/**
* Access key status to apply. Defaults to `Active`. Valid values are `Active` and `Inactive`.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> status;
/**
* @return Access key status to apply. Defaults to `Active`. Valid values are `Active` and `Inactive`.
*
*/
public Output> status() {
return Codegen.optional(this.status);
}
/**
* IAM user to associate with this access key.
*
*/
@Export(name="user", refs={String.class}, tree="[0]")
private Output user;
/**
* @return IAM user to associate with this access key.
*
*/
public Output user() {
return this.user;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public AccessKey(java.lang.String name) {
this(name, AccessKeyArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public AccessKey(java.lang.String name, AccessKeyArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public AccessKey(java.lang.String name, AccessKeyArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:iam/accessKey:AccessKey", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private AccessKey(java.lang.String name, Output id, @Nullable AccessKeyState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:iam/accessKey:AccessKey", name, state, makeResourceOptions(options, id), false);
}
private static AccessKeyArgs makeArgs(AccessKeyArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? AccessKeyArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.additionalSecretOutputs(List.of(
"secret",
"sesSmtpPasswordV4"
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static AccessKey get(java.lang.String name, Output id, @Nullable AccessKeyState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new AccessKey(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy