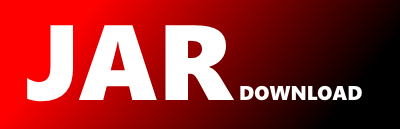
com.pulumi.aws.iam.SshKey Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.iam;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.iam.SshKeyArgs;
import com.pulumi.aws.iam.inputs.SshKeyState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import javax.annotation.Nullable;
/**
* Uploads an SSH public key and associates it with the specified IAM user.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.User;
* import com.pulumi.aws.iam.UserArgs;
* import com.pulumi.aws.iam.SshKey;
* import com.pulumi.aws.iam.SshKeyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App }{{@code
* public static void main(String[] args) }{{@code
* Pulumi.run(App::stack);
* }}{@code
*
* public static void stack(Context ctx) }{{@code
* var user = new User("user", UserArgs.builder()
* .name("test-user")
* .path("/")
* .build());
*
* var userSshKey = new SshKey("userSshKey", SshKeyArgs.builder()
* .username(user.name())
* .encoding("SSH")
* .publicKey("ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQD3F6tyPEFEzV0LX3X8BsXdMsQz1x2cEikKDEY0aIj41qgxMCP/iteneqXSIFZBp5vizPvaoIR3Um9xK7PGoW8giupGn+EPuxIA4cDM4vzOqOkiMPhz5XK0whEjkVzTo4+S0puvDZuwIsdiW9mxhJc7tgBNL0cYlWSYVkz4G/fslNfRPW5mYAM49f4fhtxPb5ok4Q2Lg9dPKVHO/Bgeu5woMc7RY0p1ej6D4CKFE6lymSDJpW0YHX/wqE9+cfEauh7xZcG0q9t2ta6F6fmX0agvpFyZo8aFbXeUBr7osSCJNgvavWbM/06niWrOvYX2xwWdhXmXSrbX8ZbabVohBK41 mytest}{@literal @}{@code mydomain.com")
* .build());
*
* }}{@code
* }}{@code
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import SSH public keys using the `username`, `ssh_public_key_id`, and `encoding`. For example:
*
* ```sh
* $ pulumi import aws:iam/sshKey:SshKey user user:APKAJNCNNJICVN7CFKCA:SSH
* ```
*
*/
@ResourceType(type="aws:iam/sshKey:SshKey")
public class SshKey extends com.pulumi.resources.CustomResource {
/**
* Specifies the public key encoding format to use in the response. To retrieve the public key in ssh-rsa format, use `SSH`. To retrieve the public key in PEM format, use `PEM`.
*
*/
@Export(name="encoding", refs={String.class}, tree="[0]")
private Output encoding;
/**
* @return Specifies the public key encoding format to use in the response. To retrieve the public key in ssh-rsa format, use `SSH`. To retrieve the public key in PEM format, use `PEM`.
*
*/
public Output encoding() {
return this.encoding;
}
/**
* The MD5 message digest of the SSH public key.
*
*/
@Export(name="fingerprint", refs={String.class}, tree="[0]")
private Output fingerprint;
/**
* @return The MD5 message digest of the SSH public key.
*
*/
public Output fingerprint() {
return this.fingerprint;
}
/**
* The SSH public key. The public key must be encoded in ssh-rsa format or PEM format.
*
*/
@Export(name="publicKey", refs={String.class}, tree="[0]")
private Output publicKey;
/**
* @return The SSH public key. The public key must be encoded in ssh-rsa format or PEM format.
*
*/
public Output publicKey() {
return this.publicKey;
}
/**
* The unique identifier for the SSH public key.
*
*/
@Export(name="sshPublicKeyId", refs={String.class}, tree="[0]")
private Output sshPublicKeyId;
/**
* @return The unique identifier for the SSH public key.
*
*/
public Output sshPublicKeyId() {
return this.sshPublicKeyId;
}
/**
* The status to assign to the SSH public key. Active means the key can be used for authentication with an AWS CodeCommit repository. Inactive means the key cannot be used. Default is `active`.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return The status to assign to the SSH public key. Active means the key can be used for authentication with an AWS CodeCommit repository. Inactive means the key cannot be used. Default is `active`.
*
*/
public Output status() {
return this.status;
}
/**
* The name of the IAM user to associate the SSH public key with.
*
*/
@Export(name="username", refs={String.class}, tree="[0]")
private Output username;
/**
* @return The name of the IAM user to associate the SSH public key with.
*
*/
public Output username() {
return this.username;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public SshKey(java.lang.String name) {
this(name, SshKeyArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public SshKey(java.lang.String name, SshKeyArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public SshKey(java.lang.String name, SshKeyArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:iam/sshKey:SshKey", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private SshKey(java.lang.String name, Output id, @Nullable SshKeyState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:iam/sshKey:SshKey", name, state, makeResourceOptions(options, id), false);
}
private static SshKeyArgs makeArgs(SshKeyArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? SshKeyArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static SshKey get(java.lang.String name, Output id, @Nullable SshKeyState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new SshKey(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy