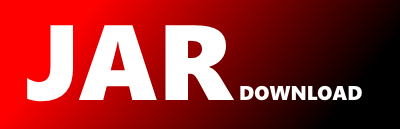
com.pulumi.aws.kinesis.outputs.FirehoseDeliveryStreamExtendedS3Configuration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.kinesis.outputs;
import com.pulumi.aws.kinesis.outputs.FirehoseDeliveryStreamExtendedS3ConfigurationCloudwatchLoggingOptions;
import com.pulumi.aws.kinesis.outputs.FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfiguration;
import com.pulumi.aws.kinesis.outputs.FirehoseDeliveryStreamExtendedS3ConfigurationDynamicPartitioningConfiguration;
import com.pulumi.aws.kinesis.outputs.FirehoseDeliveryStreamExtendedS3ConfigurationProcessingConfiguration;
import com.pulumi.aws.kinesis.outputs.FirehoseDeliveryStreamExtendedS3ConfigurationS3BackupConfiguration;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class FirehoseDeliveryStreamExtendedS3Configuration {
/**
* @return The ARN of the S3 bucket
*
*/
private String bucketArn;
private @Nullable Integer bufferingInterval;
private @Nullable Integer bufferingSize;
private @Nullable FirehoseDeliveryStreamExtendedS3ConfigurationCloudwatchLoggingOptions cloudwatchLoggingOptions;
/**
* @return The compression format. If no value is specified, the default is `UNCOMPRESSED`. Other supported values are `GZIP`, `ZIP`, `Snappy`, & `HADOOP_SNAPPY`.
*
*/
private @Nullable String compressionFormat;
/**
* @return The time zone you prefer. Valid values are `UTC` or a non-3-letter IANA time zones (for example, `America/Los_Angeles`). Default value is `UTC`.
*
*/
private @Nullable String customTimeZone;
/**
* @return Nested argument for the serializer, deserializer, and schema for converting data from the JSON format to the Parquet or ORC format before writing it to Amazon S3. See `data_format_conversion_configuration` block below for details.
*
*/
private @Nullable FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfiguration dataFormatConversionConfiguration;
/**
* @return The configuration for dynamic partitioning. Required when using [dynamic partitioning](https://docs.aws.amazon.com/firehose/latest/dev/dynamic-partitioning.html). See `dynamic_partitioning_configuration` block below for details.
*
*/
private @Nullable FirehoseDeliveryStreamExtendedS3ConfigurationDynamicPartitioningConfiguration dynamicPartitioningConfiguration;
/**
* @return Prefix added to failed records before writing them to S3. Not currently supported for `redshift` destination. This prefix appears immediately following the bucket name. For information about how to specify this prefix, see [Custom Prefixes for Amazon S3 Objects](https://docs.aws.amazon.com/firehose/latest/dev/s3-prefixes.html).
*
*/
private @Nullable String errorOutputPrefix;
/**
* @return The file extension to override the default file extension (for example, `.json`).
*
*/
private @Nullable String fileExtension;
/**
* @return Specifies the KMS key ARN the stream will use to encrypt data. If not set, no encryption will
* be used.
*
*/
private @Nullable String kmsKeyArn;
/**
* @return The "YYYY/MM/DD/HH" time format prefix is automatically used for delivered S3 files. You can specify an extra prefix to be added in front of the time format prefix. Note that if the prefix ends with a slash, it appears as a folder in the S3 bucket
*
*/
private @Nullable String prefix;
/**
* @return The data processing configuration. See `processing_configuration` block below for details.
*
*/
private @Nullable FirehoseDeliveryStreamExtendedS3ConfigurationProcessingConfiguration processingConfiguration;
private String roleArn;
/**
* @return The configuration for backup in Amazon S3. Required if `s3_backup_mode` is `Enabled`. Supports the same fields as `s3_configuration` object.
*
*/
private @Nullable FirehoseDeliveryStreamExtendedS3ConfigurationS3BackupConfiguration s3BackupConfiguration;
/**
* @return The Amazon S3 backup mode. Valid values are `Disabled` and `Enabled`. Default value is `Disabled`.
*
*/
private @Nullable String s3BackupMode;
private FirehoseDeliveryStreamExtendedS3Configuration() {}
/**
* @return The ARN of the S3 bucket
*
*/
public String bucketArn() {
return this.bucketArn;
}
public Optional bufferingInterval() {
return Optional.ofNullable(this.bufferingInterval);
}
public Optional bufferingSize() {
return Optional.ofNullable(this.bufferingSize);
}
public Optional cloudwatchLoggingOptions() {
return Optional.ofNullable(this.cloudwatchLoggingOptions);
}
/**
* @return The compression format. If no value is specified, the default is `UNCOMPRESSED`. Other supported values are `GZIP`, `ZIP`, `Snappy`, & `HADOOP_SNAPPY`.
*
*/
public Optional compressionFormat() {
return Optional.ofNullable(this.compressionFormat);
}
/**
* @return The time zone you prefer. Valid values are `UTC` or a non-3-letter IANA time zones (for example, `America/Los_Angeles`). Default value is `UTC`.
*
*/
public Optional customTimeZone() {
return Optional.ofNullable(this.customTimeZone);
}
/**
* @return Nested argument for the serializer, deserializer, and schema for converting data from the JSON format to the Parquet or ORC format before writing it to Amazon S3. See `data_format_conversion_configuration` block below for details.
*
*/
public Optional dataFormatConversionConfiguration() {
return Optional.ofNullable(this.dataFormatConversionConfiguration);
}
/**
* @return The configuration for dynamic partitioning. Required when using [dynamic partitioning](https://docs.aws.amazon.com/firehose/latest/dev/dynamic-partitioning.html). See `dynamic_partitioning_configuration` block below for details.
*
*/
public Optional dynamicPartitioningConfiguration() {
return Optional.ofNullable(this.dynamicPartitioningConfiguration);
}
/**
* @return Prefix added to failed records before writing them to S3. Not currently supported for `redshift` destination. This prefix appears immediately following the bucket name. For information about how to specify this prefix, see [Custom Prefixes for Amazon S3 Objects](https://docs.aws.amazon.com/firehose/latest/dev/s3-prefixes.html).
*
*/
public Optional errorOutputPrefix() {
return Optional.ofNullable(this.errorOutputPrefix);
}
/**
* @return The file extension to override the default file extension (for example, `.json`).
*
*/
public Optional fileExtension() {
return Optional.ofNullable(this.fileExtension);
}
/**
* @return Specifies the KMS key ARN the stream will use to encrypt data. If not set, no encryption will
* be used.
*
*/
public Optional kmsKeyArn() {
return Optional.ofNullable(this.kmsKeyArn);
}
/**
* @return The "YYYY/MM/DD/HH" time format prefix is automatically used for delivered S3 files. You can specify an extra prefix to be added in front of the time format prefix. Note that if the prefix ends with a slash, it appears as a folder in the S3 bucket
*
*/
public Optional prefix() {
return Optional.ofNullable(this.prefix);
}
/**
* @return The data processing configuration. See `processing_configuration` block below for details.
*
*/
public Optional processingConfiguration() {
return Optional.ofNullable(this.processingConfiguration);
}
public String roleArn() {
return this.roleArn;
}
/**
* @return The configuration for backup in Amazon S3. Required if `s3_backup_mode` is `Enabled`. Supports the same fields as `s3_configuration` object.
*
*/
public Optional s3BackupConfiguration() {
return Optional.ofNullable(this.s3BackupConfiguration);
}
/**
* @return The Amazon S3 backup mode. Valid values are `Disabled` and `Enabled`. Default value is `Disabled`.
*
*/
public Optional s3BackupMode() {
return Optional.ofNullable(this.s3BackupMode);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(FirehoseDeliveryStreamExtendedS3Configuration defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String bucketArn;
private @Nullable Integer bufferingInterval;
private @Nullable Integer bufferingSize;
private @Nullable FirehoseDeliveryStreamExtendedS3ConfigurationCloudwatchLoggingOptions cloudwatchLoggingOptions;
private @Nullable String compressionFormat;
private @Nullable String customTimeZone;
private @Nullable FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfiguration dataFormatConversionConfiguration;
private @Nullable FirehoseDeliveryStreamExtendedS3ConfigurationDynamicPartitioningConfiguration dynamicPartitioningConfiguration;
private @Nullable String errorOutputPrefix;
private @Nullable String fileExtension;
private @Nullable String kmsKeyArn;
private @Nullable String prefix;
private @Nullable FirehoseDeliveryStreamExtendedS3ConfigurationProcessingConfiguration processingConfiguration;
private String roleArn;
private @Nullable FirehoseDeliveryStreamExtendedS3ConfigurationS3BackupConfiguration s3BackupConfiguration;
private @Nullable String s3BackupMode;
public Builder() {}
public Builder(FirehoseDeliveryStreamExtendedS3Configuration defaults) {
Objects.requireNonNull(defaults);
this.bucketArn = defaults.bucketArn;
this.bufferingInterval = defaults.bufferingInterval;
this.bufferingSize = defaults.bufferingSize;
this.cloudwatchLoggingOptions = defaults.cloudwatchLoggingOptions;
this.compressionFormat = defaults.compressionFormat;
this.customTimeZone = defaults.customTimeZone;
this.dataFormatConversionConfiguration = defaults.dataFormatConversionConfiguration;
this.dynamicPartitioningConfiguration = defaults.dynamicPartitioningConfiguration;
this.errorOutputPrefix = defaults.errorOutputPrefix;
this.fileExtension = defaults.fileExtension;
this.kmsKeyArn = defaults.kmsKeyArn;
this.prefix = defaults.prefix;
this.processingConfiguration = defaults.processingConfiguration;
this.roleArn = defaults.roleArn;
this.s3BackupConfiguration = defaults.s3BackupConfiguration;
this.s3BackupMode = defaults.s3BackupMode;
}
@CustomType.Setter
public Builder bucketArn(String bucketArn) {
if (bucketArn == null) {
throw new MissingRequiredPropertyException("FirehoseDeliveryStreamExtendedS3Configuration", "bucketArn");
}
this.bucketArn = bucketArn;
return this;
}
@CustomType.Setter
public Builder bufferingInterval(@Nullable Integer bufferingInterval) {
this.bufferingInterval = bufferingInterval;
return this;
}
@CustomType.Setter
public Builder bufferingSize(@Nullable Integer bufferingSize) {
this.bufferingSize = bufferingSize;
return this;
}
@CustomType.Setter
public Builder cloudwatchLoggingOptions(@Nullable FirehoseDeliveryStreamExtendedS3ConfigurationCloudwatchLoggingOptions cloudwatchLoggingOptions) {
this.cloudwatchLoggingOptions = cloudwatchLoggingOptions;
return this;
}
@CustomType.Setter
public Builder compressionFormat(@Nullable String compressionFormat) {
this.compressionFormat = compressionFormat;
return this;
}
@CustomType.Setter
public Builder customTimeZone(@Nullable String customTimeZone) {
this.customTimeZone = customTimeZone;
return this;
}
@CustomType.Setter
public Builder dataFormatConversionConfiguration(@Nullable FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfiguration dataFormatConversionConfiguration) {
this.dataFormatConversionConfiguration = dataFormatConversionConfiguration;
return this;
}
@CustomType.Setter
public Builder dynamicPartitioningConfiguration(@Nullable FirehoseDeliveryStreamExtendedS3ConfigurationDynamicPartitioningConfiguration dynamicPartitioningConfiguration) {
this.dynamicPartitioningConfiguration = dynamicPartitioningConfiguration;
return this;
}
@CustomType.Setter
public Builder errorOutputPrefix(@Nullable String errorOutputPrefix) {
this.errorOutputPrefix = errorOutputPrefix;
return this;
}
@CustomType.Setter
public Builder fileExtension(@Nullable String fileExtension) {
this.fileExtension = fileExtension;
return this;
}
@CustomType.Setter
public Builder kmsKeyArn(@Nullable String kmsKeyArn) {
this.kmsKeyArn = kmsKeyArn;
return this;
}
@CustomType.Setter
public Builder prefix(@Nullable String prefix) {
this.prefix = prefix;
return this;
}
@CustomType.Setter
public Builder processingConfiguration(@Nullable FirehoseDeliveryStreamExtendedS3ConfigurationProcessingConfiguration processingConfiguration) {
this.processingConfiguration = processingConfiguration;
return this;
}
@CustomType.Setter
public Builder roleArn(String roleArn) {
if (roleArn == null) {
throw new MissingRequiredPropertyException("FirehoseDeliveryStreamExtendedS3Configuration", "roleArn");
}
this.roleArn = roleArn;
return this;
}
@CustomType.Setter
public Builder s3BackupConfiguration(@Nullable FirehoseDeliveryStreamExtendedS3ConfigurationS3BackupConfiguration s3BackupConfiguration) {
this.s3BackupConfiguration = s3BackupConfiguration;
return this;
}
@CustomType.Setter
public Builder s3BackupMode(@Nullable String s3BackupMode) {
this.s3BackupMode = s3BackupMode;
return this;
}
public FirehoseDeliveryStreamExtendedS3Configuration build() {
final var _resultValue = new FirehoseDeliveryStreamExtendedS3Configuration();
_resultValue.bucketArn = bucketArn;
_resultValue.bufferingInterval = bufferingInterval;
_resultValue.bufferingSize = bufferingSize;
_resultValue.cloudwatchLoggingOptions = cloudwatchLoggingOptions;
_resultValue.compressionFormat = compressionFormat;
_resultValue.customTimeZone = customTimeZone;
_resultValue.dataFormatConversionConfiguration = dataFormatConversionConfiguration;
_resultValue.dynamicPartitioningConfiguration = dynamicPartitioningConfiguration;
_resultValue.errorOutputPrefix = errorOutputPrefix;
_resultValue.fileExtension = fileExtension;
_resultValue.kmsKeyArn = kmsKeyArn;
_resultValue.prefix = prefix;
_resultValue.processingConfiguration = processingConfiguration;
_resultValue.roleArn = roleArn;
_resultValue.s3BackupConfiguration = s3BackupConfiguration;
_resultValue.s3BackupMode = s3BackupMode;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy