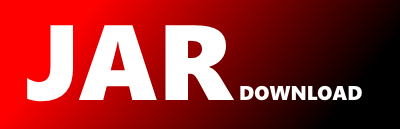
com.pulumi.aws.lakeformation.outputs.PermissionsTableWithColumns Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.lakeformation.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class PermissionsTableWithColumns {
/**
* @return Identifier for the Data Catalog. By default, it is the account ID of the caller.
*
*/
private @Nullable String catalogId;
/**
* @return Set of column names for the table.
*
*/
private @Nullable List columnNames;
/**
* @return Name of the database for the table with columns resource. Unique to the Data Catalog.
*
*/
private String databaseName;
/**
* @return Set of column names for the table to exclude. If `excluded_column_names` is included, `wildcard` must be set to `true` to avoid the provider reporting a difference.
*
*/
private @Nullable List excludedColumnNames;
/**
* @return Name of the table resource.
*
*/
private String name;
/**
* @return Whether to use a column wildcard. If `excluded_column_names` is included, `wildcard` must be set to `true` to avoid the provider reporting a difference.
*
* The following arguments are optional:
*
*/
private @Nullable Boolean wildcard;
private PermissionsTableWithColumns() {}
/**
* @return Identifier for the Data Catalog. By default, it is the account ID of the caller.
*
*/
public Optional catalogId() {
return Optional.ofNullable(this.catalogId);
}
/**
* @return Set of column names for the table.
*
*/
public List columnNames() {
return this.columnNames == null ? List.of() : this.columnNames;
}
/**
* @return Name of the database for the table with columns resource. Unique to the Data Catalog.
*
*/
public String databaseName() {
return this.databaseName;
}
/**
* @return Set of column names for the table to exclude. If `excluded_column_names` is included, `wildcard` must be set to `true` to avoid the provider reporting a difference.
*
*/
public List excludedColumnNames() {
return this.excludedColumnNames == null ? List.of() : this.excludedColumnNames;
}
/**
* @return Name of the table resource.
*
*/
public String name() {
return this.name;
}
/**
* @return Whether to use a column wildcard. If `excluded_column_names` is included, `wildcard` must be set to `true` to avoid the provider reporting a difference.
*
* The following arguments are optional:
*
*/
public Optional wildcard() {
return Optional.ofNullable(this.wildcard);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(PermissionsTableWithColumns defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String catalogId;
private @Nullable List columnNames;
private String databaseName;
private @Nullable List excludedColumnNames;
private String name;
private @Nullable Boolean wildcard;
public Builder() {}
public Builder(PermissionsTableWithColumns defaults) {
Objects.requireNonNull(defaults);
this.catalogId = defaults.catalogId;
this.columnNames = defaults.columnNames;
this.databaseName = defaults.databaseName;
this.excludedColumnNames = defaults.excludedColumnNames;
this.name = defaults.name;
this.wildcard = defaults.wildcard;
}
@CustomType.Setter
public Builder catalogId(@Nullable String catalogId) {
this.catalogId = catalogId;
return this;
}
@CustomType.Setter
public Builder columnNames(@Nullable List columnNames) {
this.columnNames = columnNames;
return this;
}
public Builder columnNames(String... columnNames) {
return columnNames(List.of(columnNames));
}
@CustomType.Setter
public Builder databaseName(String databaseName) {
if (databaseName == null) {
throw new MissingRequiredPropertyException("PermissionsTableWithColumns", "databaseName");
}
this.databaseName = databaseName;
return this;
}
@CustomType.Setter
public Builder excludedColumnNames(@Nullable List excludedColumnNames) {
this.excludedColumnNames = excludedColumnNames;
return this;
}
public Builder excludedColumnNames(String... excludedColumnNames) {
return excludedColumnNames(List.of(excludedColumnNames));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("PermissionsTableWithColumns", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder wildcard(@Nullable Boolean wildcard) {
this.wildcard = wildcard;
return this;
}
public PermissionsTableWithColumns build() {
final var _resultValue = new PermissionsTableWithColumns();
_resultValue.catalogId = catalogId;
_resultValue.columnNames = columnNames;
_resultValue.databaseName = databaseName;
_resultValue.excludedColumnNames = excludedColumnNames;
_resultValue.name = name;
_resultValue.wildcard = wildcard;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy