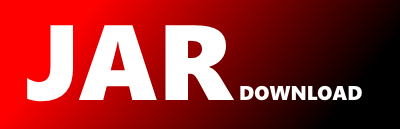
com.pulumi.aws.networkfirewall.inputs.RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.networkfirewall.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
public final class RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs extends com.pulumi.resources.ResourceArgs {
public static final RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs Empty = new RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs();
/**
* The destination IP address or address range to inspect for, in CIDR notation. To match with any address, specify `ANY`.
*
*/
@Import(name="destination", required=true)
private Output destination;
/**
* @return The destination IP address or address range to inspect for, in CIDR notation. To match with any address, specify `ANY`.
*
*/
public Output destination() {
return this.destination;
}
/**
* The destination port to inspect for. To match with any address, specify `ANY`.
*
*/
@Import(name="destinationPort", required=true)
private Output destinationPort;
/**
* @return The destination port to inspect for. To match with any address, specify `ANY`.
*
*/
public Output destinationPort() {
return this.destinationPort;
}
/**
* The direction of traffic flow to inspect. Valid values: `ANY` or `FORWARD`.
*
*/
@Import(name="direction", required=true)
private Output direction;
/**
* @return The direction of traffic flow to inspect. Valid values: `ANY` or `FORWARD`.
*
*/
public Output direction() {
return this.direction;
}
/**
* The protocol to inspect. Valid values: `IP`, `TCP`, `UDP`, `ICMP`, `HTTP`, `FTP`, `TLS`, `SMB`, `DNS`, `DCERPC`, `SSH`, `SMTP`, `IMAP`, `MSN`, `KRB5`, `IKEV2`, `TFTP`, `NTP`, `DHCP`.
*
*/
@Import(name="protocol", required=true)
private Output protocol;
/**
* @return The protocol to inspect. Valid values: `IP`, `TCP`, `UDP`, `ICMP`, `HTTP`, `FTP`, `TLS`, `SMB`, `DNS`, `DCERPC`, `SSH`, `SMTP`, `IMAP`, `MSN`, `KRB5`, `IKEV2`, `TFTP`, `NTP`, `DHCP`.
*
*/
public Output protocol() {
return this.protocol;
}
/**
* The source IP address or address range for, in CIDR notation. To match with any address, specify `ANY`.
*
*/
@Import(name="source", required=true)
private Output source;
/**
* @return The source IP address or address range for, in CIDR notation. To match with any address, specify `ANY`.
*
*/
public Output source() {
return this.source;
}
/**
* The source port to inspect for. To match with any address, specify `ANY`.
*
*/
@Import(name="sourcePort", required=true)
private Output sourcePort;
/**
* @return The source port to inspect for. To match with any address, specify `ANY`.
*
*/
public Output sourcePort() {
return this.sourcePort;
}
private RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs() {}
private RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs(RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs $) {
this.destination = $.destination;
this.destinationPort = $.destinationPort;
this.direction = $.direction;
this.protocol = $.protocol;
this.source = $.source;
this.sourcePort = $.sourcePort;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs $;
public Builder() {
$ = new RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs();
}
public Builder(RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs defaults) {
$ = new RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs(Objects.requireNonNull(defaults));
}
/**
* @param destination The destination IP address or address range to inspect for, in CIDR notation. To match with any address, specify `ANY`.
*
* @return builder
*
*/
public Builder destination(Output destination) {
$.destination = destination;
return this;
}
/**
* @param destination The destination IP address or address range to inspect for, in CIDR notation. To match with any address, specify `ANY`.
*
* @return builder
*
*/
public Builder destination(String destination) {
return destination(Output.of(destination));
}
/**
* @param destinationPort The destination port to inspect for. To match with any address, specify `ANY`.
*
* @return builder
*
*/
public Builder destinationPort(Output destinationPort) {
$.destinationPort = destinationPort;
return this;
}
/**
* @param destinationPort The destination port to inspect for. To match with any address, specify `ANY`.
*
* @return builder
*
*/
public Builder destinationPort(String destinationPort) {
return destinationPort(Output.of(destinationPort));
}
/**
* @param direction The direction of traffic flow to inspect. Valid values: `ANY` or `FORWARD`.
*
* @return builder
*
*/
public Builder direction(Output direction) {
$.direction = direction;
return this;
}
/**
* @param direction The direction of traffic flow to inspect. Valid values: `ANY` or `FORWARD`.
*
* @return builder
*
*/
public Builder direction(String direction) {
return direction(Output.of(direction));
}
/**
* @param protocol The protocol to inspect. Valid values: `IP`, `TCP`, `UDP`, `ICMP`, `HTTP`, `FTP`, `TLS`, `SMB`, `DNS`, `DCERPC`, `SSH`, `SMTP`, `IMAP`, `MSN`, `KRB5`, `IKEV2`, `TFTP`, `NTP`, `DHCP`.
*
* @return builder
*
*/
public Builder protocol(Output protocol) {
$.protocol = protocol;
return this;
}
/**
* @param protocol The protocol to inspect. Valid values: `IP`, `TCP`, `UDP`, `ICMP`, `HTTP`, `FTP`, `TLS`, `SMB`, `DNS`, `DCERPC`, `SSH`, `SMTP`, `IMAP`, `MSN`, `KRB5`, `IKEV2`, `TFTP`, `NTP`, `DHCP`.
*
* @return builder
*
*/
public Builder protocol(String protocol) {
return protocol(Output.of(protocol));
}
/**
* @param source The source IP address or address range for, in CIDR notation. To match with any address, specify `ANY`.
*
* @return builder
*
*/
public Builder source(Output source) {
$.source = source;
return this;
}
/**
* @param source The source IP address or address range for, in CIDR notation. To match with any address, specify `ANY`.
*
* @return builder
*
*/
public Builder source(String source) {
return source(Output.of(source));
}
/**
* @param sourcePort The source port to inspect for. To match with any address, specify `ANY`.
*
* @return builder
*
*/
public Builder sourcePort(Output sourcePort) {
$.sourcePort = sourcePort;
return this;
}
/**
* @param sourcePort The source port to inspect for. To match with any address, specify `ANY`.
*
* @return builder
*
*/
public Builder sourcePort(String sourcePort) {
return sourcePort(Output.of(sourcePort));
}
public RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs build() {
if ($.destination == null) {
throw new MissingRequiredPropertyException("RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs", "destination");
}
if ($.destinationPort == null) {
throw new MissingRequiredPropertyException("RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs", "destinationPort");
}
if ($.direction == null) {
throw new MissingRequiredPropertyException("RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs", "direction");
}
if ($.protocol == null) {
throw new MissingRequiredPropertyException("RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs", "protocol");
}
if ($.source == null) {
throw new MissingRequiredPropertyException("RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs", "source");
}
if ($.sourcePort == null) {
throw new MissingRequiredPropertyException("RuleGroupRuleGroupRulesSourceStatefulRuleHeaderArgs", "sourcePort");
}
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy