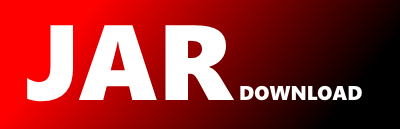
com.pulumi.aws.pipes.inputs.PipeLogConfigurationArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.pipes.inputs;
import com.pulumi.aws.pipes.inputs.PipeLogConfigurationCloudwatchLogsLogDestinationArgs;
import com.pulumi.aws.pipes.inputs.PipeLogConfigurationFirehoseLogDestinationArgs;
import com.pulumi.aws.pipes.inputs.PipeLogConfigurationS3LogDestinationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PipeLogConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final PipeLogConfigurationArgs Empty = new PipeLogConfigurationArgs();
/**
* Amazon CloudWatch Logs logging configuration settings for the pipe. Detailed below.
*
*/
@Import(name="cloudwatchLogsLogDestination")
private @Nullable Output cloudwatchLogsLogDestination;
/**
* @return Amazon CloudWatch Logs logging configuration settings for the pipe. Detailed below.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy