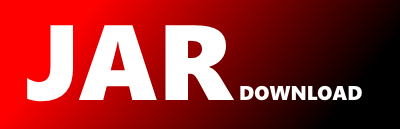
com.pulumi.aws.pipes.outputs.PipeTargetParametersEcsTaskParametersOverridesContainerOverride Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.pipes.outputs;
import com.pulumi.aws.pipes.outputs.PipeTargetParametersEcsTaskParametersOverridesContainerOverrideEnvironment;
import com.pulumi.aws.pipes.outputs.PipeTargetParametersEcsTaskParametersOverridesContainerOverrideEnvironmentFile;
import com.pulumi.aws.pipes.outputs.PipeTargetParametersEcsTaskParametersOverridesContainerOverrideResourceRequirement;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class PipeTargetParametersEcsTaskParametersOverridesContainerOverride {
/**
* @return List of commands to send to the container that overrides the default command from the Docker image or the task definition. You must also specify a container name.
*
*/
private @Nullable List commands;
/**
* @return The number of cpu units reserved for the container, instead of the default value from the task definition. You must also specify a container name.
*
*/
private @Nullable Integer cpu;
/**
* @return A list of files containing the environment variables to pass to a container, instead of the value from the container definition. Detailed below.
*
*/
private @Nullable List environmentFiles;
/**
* @return The environment variables to send to the container. You can add new environment variables, which are added to the container at launch, or you can override the existing environment variables from the Docker image or the task definition. You must also specify a container name. Detailed below.
*
*/
private @Nullable List environments;
/**
* @return The hard limit (in MiB) of memory to present to the container, instead of the default value from the task definition. If your container attempts to exceed the memory specified here, the container is killed. You must also specify a container name.
*
*/
private @Nullable Integer memory;
/**
* @return The soft limit (in MiB) of memory to reserve for the container, instead of the default value from the task definition. You must also specify a container name.
*
*/
private @Nullable Integer memoryReservation;
/**
* @return Name of the pipe. If omitted, the provider will assign a random, unique name. Conflicts with `name_prefix`.
*
*/
private @Nullable String name;
/**
* @return The type and amount of a resource to assign to a container, instead of the default value from the task definition. The only supported resource is a GPU. Detailed below.
*
*/
private @Nullable List resourceRequirements;
private PipeTargetParametersEcsTaskParametersOverridesContainerOverride() {}
/**
* @return List of commands to send to the container that overrides the default command from the Docker image or the task definition. You must also specify a container name.
*
*/
public List commands() {
return this.commands == null ? List.of() : this.commands;
}
/**
* @return The number of cpu units reserved for the container, instead of the default value from the task definition. You must also specify a container name.
*
*/
public Optional cpu() {
return Optional.ofNullable(this.cpu);
}
/**
* @return A list of files containing the environment variables to pass to a container, instead of the value from the container definition. Detailed below.
*
*/
public List environmentFiles() {
return this.environmentFiles == null ? List.of() : this.environmentFiles;
}
/**
* @return The environment variables to send to the container. You can add new environment variables, which are added to the container at launch, or you can override the existing environment variables from the Docker image or the task definition. You must also specify a container name. Detailed below.
*
*/
public List environments() {
return this.environments == null ? List.of() : this.environments;
}
/**
* @return The hard limit (in MiB) of memory to present to the container, instead of the default value from the task definition. If your container attempts to exceed the memory specified here, the container is killed. You must also specify a container name.
*
*/
public Optional memory() {
return Optional.ofNullable(this.memory);
}
/**
* @return The soft limit (in MiB) of memory to reserve for the container, instead of the default value from the task definition. You must also specify a container name.
*
*/
public Optional memoryReservation() {
return Optional.ofNullable(this.memoryReservation);
}
/**
* @return Name of the pipe. If omitted, the provider will assign a random, unique name. Conflicts with `name_prefix`.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The type and amount of a resource to assign to a container, instead of the default value from the task definition. The only supported resource is a GPU. Detailed below.
*
*/
public List resourceRequirements() {
return this.resourceRequirements == null ? List.of() : this.resourceRequirements;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(PipeTargetParametersEcsTaskParametersOverridesContainerOverride defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List commands;
private @Nullable Integer cpu;
private @Nullable List environmentFiles;
private @Nullable List environments;
private @Nullable Integer memory;
private @Nullable Integer memoryReservation;
private @Nullable String name;
private @Nullable List resourceRequirements;
public Builder() {}
public Builder(PipeTargetParametersEcsTaskParametersOverridesContainerOverride defaults) {
Objects.requireNonNull(defaults);
this.commands = defaults.commands;
this.cpu = defaults.cpu;
this.environmentFiles = defaults.environmentFiles;
this.environments = defaults.environments;
this.memory = defaults.memory;
this.memoryReservation = defaults.memoryReservation;
this.name = defaults.name;
this.resourceRequirements = defaults.resourceRequirements;
}
@CustomType.Setter
public Builder commands(@Nullable List commands) {
this.commands = commands;
return this;
}
public Builder commands(String... commands) {
return commands(List.of(commands));
}
@CustomType.Setter
public Builder cpu(@Nullable Integer cpu) {
this.cpu = cpu;
return this;
}
@CustomType.Setter
public Builder environmentFiles(@Nullable List environmentFiles) {
this.environmentFiles = environmentFiles;
return this;
}
public Builder environmentFiles(PipeTargetParametersEcsTaskParametersOverridesContainerOverrideEnvironmentFile... environmentFiles) {
return environmentFiles(List.of(environmentFiles));
}
@CustomType.Setter
public Builder environments(@Nullable List environments) {
this.environments = environments;
return this;
}
public Builder environments(PipeTargetParametersEcsTaskParametersOverridesContainerOverrideEnvironment... environments) {
return environments(List.of(environments));
}
@CustomType.Setter
public Builder memory(@Nullable Integer memory) {
this.memory = memory;
return this;
}
@CustomType.Setter
public Builder memoryReservation(@Nullable Integer memoryReservation) {
this.memoryReservation = memoryReservation;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder resourceRequirements(@Nullable List resourceRequirements) {
this.resourceRequirements = resourceRequirements;
return this;
}
public Builder resourceRequirements(PipeTargetParametersEcsTaskParametersOverridesContainerOverrideResourceRequirement... resourceRequirements) {
return resourceRequirements(List.of(resourceRequirements));
}
public PipeTargetParametersEcsTaskParametersOverridesContainerOverride build() {
final var _resultValue = new PipeTargetParametersEcsTaskParametersOverridesContainerOverride();
_resultValue.commands = commands;
_resultValue.cpu = cpu;
_resultValue.environmentFiles = environmentFiles;
_resultValue.environments = environments;
_resultValue.memory = memory;
_resultValue.memoryReservation = memoryReservation;
_resultValue.name = name;
_resultValue.resourceRequirements = resourceRequirements;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy