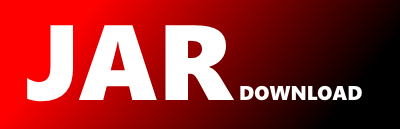
com.pulumi.aws.qldb.Stream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.qldb;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.qldb.StreamArgs;
import com.pulumi.aws.qldb.inputs.StreamState;
import com.pulumi.aws.qldb.outputs.StreamKinesisConfiguration;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an AWS Quantum Ledger Database (QLDB) Stream resource
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.qldb.Stream;
* import com.pulumi.aws.qldb.StreamArgs;
* import com.pulumi.aws.qldb.inputs.StreamKinesisConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Stream("example", StreamArgs.builder()
* .ledgerName("existing-ledger-name")
* .streamName("sample-ledger-stream")
* .roleArn("sample-role-arn")
* .inclusiveStartTime("2021-01-01T00:00:00Z")
* .kinesisConfiguration(StreamKinesisConfigurationArgs.builder()
* .aggregationEnabled(false)
* .streamArn("arn:aws:kinesis:us-east-1:xxxxxxxxxxxx:stream/example-kinesis-stream")
* .build())
* .tags(Map.of("example", "tag"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
*/
@ResourceType(type="aws:qldb/stream:Stream")
public class Stream extends com.pulumi.resources.CustomResource {
/**
* The ARN of the QLDB Stream.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The ARN of the QLDB Stream.
*
*/
public Output arn() {
return this.arn;
}
/**
* The exclusive date and time that specifies when the stream ends. If you don't define this parameter, the stream runs indefinitely until you cancel it. It must be in ISO 8601 date and time format and in Universal Coordinated Time (UTC). For example: `"2019-06-13T21:36:34Z"`.
*
*/
@Export(name="exclusiveEndTime", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> exclusiveEndTime;
/**
* @return The exclusive date and time that specifies when the stream ends. If you don't define this parameter, the stream runs indefinitely until you cancel it. It must be in ISO 8601 date and time format and in Universal Coordinated Time (UTC). For example: `"2019-06-13T21:36:34Z"`.
*
*/
public Output> exclusiveEndTime() {
return Codegen.optional(this.exclusiveEndTime);
}
/**
* The inclusive start date and time from which to start streaming journal data. This parameter must be in ISO 8601 date and time format and in Universal Coordinated Time (UTC). For example: `"2019-06-13T21:36:34Z"`. This cannot be in the future and must be before `exclusive_end_time`. If you provide a value that is before the ledger's `CreationDateTime`, QLDB effectively defaults it to the ledger's `CreationDateTime`.
*
*/
@Export(name="inclusiveStartTime", refs={String.class}, tree="[0]")
private Output inclusiveStartTime;
/**
* @return The inclusive start date and time from which to start streaming journal data. This parameter must be in ISO 8601 date and time format and in Universal Coordinated Time (UTC). For example: `"2019-06-13T21:36:34Z"`. This cannot be in the future and must be before `exclusive_end_time`. If you provide a value that is before the ledger's `CreationDateTime`, QLDB effectively defaults it to the ledger's `CreationDateTime`.
*
*/
public Output inclusiveStartTime() {
return this.inclusiveStartTime;
}
/**
* The configuration settings of the Kinesis Data Streams destination for your stream request. Documented below.
*
*/
@Export(name="kinesisConfiguration", refs={StreamKinesisConfiguration.class}, tree="[0]")
private Output kinesisConfiguration;
/**
* @return The configuration settings of the Kinesis Data Streams destination for your stream request. Documented below.
*
*/
public Output kinesisConfiguration() {
return this.kinesisConfiguration;
}
/**
* The name of the QLDB ledger.
*
*/
@Export(name="ledgerName", refs={String.class}, tree="[0]")
private Output ledgerName;
/**
* @return The name of the QLDB ledger.
*
*/
public Output ledgerName() {
return this.ledgerName;
}
/**
* The Amazon Resource Name (ARN) of the IAM role that grants QLDB permissions for a journal stream to write data records to a Kinesis Data Streams resource.
*
*/
@Export(name="roleArn", refs={String.class}, tree="[0]")
private Output roleArn;
/**
* @return The Amazon Resource Name (ARN) of the IAM role that grants QLDB permissions for a journal stream to write data records to a Kinesis Data Streams resource.
*
*/
public Output roleArn() {
return this.roleArn;
}
/**
* The name that you want to assign to the QLDB journal stream. User-defined names can help identify and indicate the purpose of a stream. Your stream name must be unique among other active streams for a given ledger. Stream names have the same naming constraints as ledger names, as defined in the [Amazon QLDB Developer Guide](https://docs.aws.amazon.com/qldb/latest/developerguide/limits.html#limits.naming).
*
*/
@Export(name="streamName", refs={String.class}, tree="[0]")
private Output streamName;
/**
* @return The name that you want to assign to the QLDB journal stream. User-defined names can help identify and indicate the purpose of a stream. Your stream name must be unique among other active streams for a given ledger. Stream names have the same naming constraints as ledger names, as defined in the [Amazon QLDB Developer Guide](https://docs.aws.amazon.com/qldb/latest/developerguide/limits.html#limits.naming).
*
*/
public Output streamName() {
return this.streamName;
}
/**
* Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy