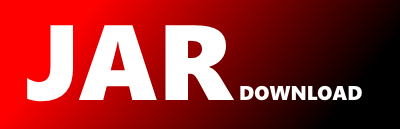
com.pulumi.aws.quicksight.AccountSubscription Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.quicksight;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.quicksight.AccountSubscriptionArgs;
import com.pulumi.aws.quicksight.inputs.AccountSubscriptionState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Resource for managing an AWS QuickSight Account Subscription.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.quicksight.AccountSubscription;
* import com.pulumi.aws.quicksight.AccountSubscriptionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App }{{@code
* public static void main(String[] args) }{{@code
* Pulumi.run(App::stack);
* }}{@code
*
* public static void stack(Context ctx) }{{@code
* var subscription = new AccountSubscription("subscription", AccountSubscriptionArgs.builder()
* .accountName("quicksight-pulumi")
* .authenticationMethod("IAM_AND_QUICKSIGHT")
* .edition("ENTERPRISE")
* .notificationEmail("notification}{@literal @}{@code email.com")
* .build());
*
* }}{@code
* }}{@code
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* You cannot import this resource.
*
*/
@ResourceType(type="aws:quicksight/accountSubscription:AccountSubscription")
public class AccountSubscription extends com.pulumi.resources.CustomResource {
/**
* Name of your Amazon QuickSight account. This name is unique over all of AWS, and it appears only when users sign in.
*
*/
@Export(name="accountName", refs={String.class}, tree="[0]")
private Output accountName;
/**
* @return Name of your Amazon QuickSight account. This name is unique over all of AWS, and it appears only when users sign in.
*
*/
public Output accountName() {
return this.accountName;
}
/**
* Status of the Amazon QuickSight account's subscription.
*
*/
@Export(name="accountSubscriptionStatus", refs={String.class}, tree="[0]")
private Output accountSubscriptionStatus;
/**
* @return Status of the Amazon QuickSight account's subscription.
*
*/
public Output accountSubscriptionStatus() {
return this.accountSubscriptionStatus;
}
/**
* Name of your Active Directory. This field is required if `ACTIVE_DIRECTORY` is the selected authentication method of the new Amazon QuickSight account.
*
*/
@Export(name="activeDirectoryName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> activeDirectoryName;
/**
* @return Name of your Active Directory. This field is required if `ACTIVE_DIRECTORY` is the selected authentication method of the new Amazon QuickSight account.
*
*/
public Output> activeDirectoryName() {
return Codegen.optional(this.activeDirectoryName);
}
/**
* Admin group associated with your Active Directory. This field is required if `ACTIVE_DIRECTORY` is the selected authentication method of the new Amazon QuickSight account.
*
*/
@Export(name="adminGroups", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> adminGroups;
/**
* @return Admin group associated with your Active Directory. This field is required if `ACTIVE_DIRECTORY` is the selected authentication method of the new Amazon QuickSight account.
*
*/
public Output>> adminGroups() {
return Codegen.optional(this.adminGroups);
}
/**
* Method that you want to use to authenticate your Amazon QuickSight account. Currently, the valid values for this parameter are `IAM_AND_QUICKSIGHT`, `IAM_ONLY`, `IAM_IDENTITY_CENTER`, and `ACTIVE_DIRECTORY`.
*
*/
@Export(name="authenticationMethod", refs={String.class}, tree="[0]")
private Output authenticationMethod;
/**
* @return Method that you want to use to authenticate your Amazon QuickSight account. Currently, the valid values for this parameter are `IAM_AND_QUICKSIGHT`, `IAM_ONLY`, `IAM_IDENTITY_CENTER`, and `ACTIVE_DIRECTORY`.
*
*/
public Output authenticationMethod() {
return this.authenticationMethod;
}
/**
* Author group associated with your Active Directory.
*
*/
@Export(name="authorGroups", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> authorGroups;
/**
* @return Author group associated with your Active Directory.
*
*/
public Output>> authorGroups() {
return Codegen.optional(this.authorGroups);
}
/**
* AWS account ID hosting the QuickSight account. Default to provider account.
*
*/
@Export(name="awsAccountId", refs={String.class}, tree="[0]")
private Output awsAccountId;
/**
* @return AWS account ID hosting the QuickSight account. Default to provider account.
*
*/
public Output awsAccountId() {
return this.awsAccountId;
}
/**
* A 10-digit phone number for the author of the Amazon QuickSight account to use for future communications. This field is required if `ENTERPPRISE_AND_Q` is the selected edition of the new Amazon QuickSight account.
*
*/
@Export(name="contactNumber", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> contactNumber;
/**
* @return A 10-digit phone number for the author of the Amazon QuickSight account to use for future communications. This field is required if `ENTERPPRISE_AND_Q` is the selected edition of the new Amazon QuickSight account.
*
*/
public Output> contactNumber() {
return Codegen.optional(this.contactNumber);
}
/**
* Active Directory ID that is associated with your Amazon QuickSight account.
*
*/
@Export(name="directoryId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> directoryId;
/**
* @return Active Directory ID that is associated with your Amazon QuickSight account.
*
*/
public Output> directoryId() {
return Codegen.optional(this.directoryId);
}
/**
* Edition of Amazon QuickSight that you want your account to have. Currently, you can choose from `STANDARD`, `ENTERPRISE` or `ENTERPRISE_AND_Q`.
*
*/
@Export(name="edition", refs={String.class}, tree="[0]")
private Output edition;
/**
* @return Edition of Amazon QuickSight that you want your account to have. Currently, you can choose from `STANDARD`, `ENTERPRISE` or `ENTERPRISE_AND_Q`.
*
*/
public Output edition() {
return this.edition;
}
/**
* Email address of the author of the Amazon QuickSight account to use for future communications. This field is required if `ENTERPPRISE_AND_Q` is the selected edition of the new Amazon QuickSight account.
*
*/
@Export(name="emailAddress", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> emailAddress;
/**
* @return Email address of the author of the Amazon QuickSight account to use for future communications. This field is required if `ENTERPPRISE_AND_Q` is the selected edition of the new Amazon QuickSight account.
*
*/
public Output> emailAddress() {
return Codegen.optional(this.emailAddress);
}
/**
* First name of the author of the Amazon QuickSight account to use for future communications. This field is required if `ENTERPPRISE_AND_Q` is the selected edition of the new Amazon QuickSight account.
*
*/
@Export(name="firstName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> firstName;
/**
* @return First name of the author of the Amazon QuickSight account to use for future communications. This field is required if `ENTERPPRISE_AND_Q` is the selected edition of the new Amazon QuickSight account.
*
*/
public Output> firstName() {
return Codegen.optional(this.firstName);
}
/**
* The Amazon Resource Name (ARN) for the IAM Identity Center instance.
*
*/
@Export(name="iamIdentityCenterInstanceArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> iamIdentityCenterInstanceArn;
/**
* @return The Amazon Resource Name (ARN) for the IAM Identity Center instance.
*
*/
public Output> iamIdentityCenterInstanceArn() {
return Codegen.optional(this.iamIdentityCenterInstanceArn);
}
/**
* Last name of the author of the Amazon QuickSight account to use for future communications. This field is required if `ENTERPPRISE_AND_Q` is the selected edition of the new Amazon QuickSight account.
*
*/
@Export(name="lastName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> lastName;
/**
* @return Last name of the author of the Amazon QuickSight account to use for future communications. This field is required if `ENTERPPRISE_AND_Q` is the selected edition of the new Amazon QuickSight account.
*
*/
public Output> lastName() {
return Codegen.optional(this.lastName);
}
/**
* Email address that you want Amazon QuickSight to send notifications to regarding your Amazon QuickSight account or Amazon QuickSight subscription.
*
* The following arguments are optional:
*
*/
@Export(name="notificationEmail", refs={String.class}, tree="[0]")
private Output notificationEmail;
/**
* @return Email address that you want Amazon QuickSight to send notifications to regarding your Amazon QuickSight account or Amazon QuickSight subscription.
*
* The following arguments are optional:
*
*/
public Output notificationEmail() {
return this.notificationEmail;
}
/**
* Reader group associated with your Active Direcrtory.
*
*/
@Export(name="readerGroups", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> readerGroups;
/**
* @return Reader group associated with your Active Direcrtory.
*
*/
public Output>> readerGroups() {
return Codegen.optional(this.readerGroups);
}
/**
* Realm of the Active Directory that is associated with your Amazon QuickSight account.
*
*/
@Export(name="realm", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> realm;
/**
* @return Realm of the Active Directory that is associated with your Amazon QuickSight account.
*
*/
public Output> realm() {
return Codegen.optional(this.realm);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public AccountSubscription(java.lang.String name) {
this(name, AccountSubscriptionArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public AccountSubscription(java.lang.String name, AccountSubscriptionArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public AccountSubscription(java.lang.String name, AccountSubscriptionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:quicksight/accountSubscription:AccountSubscription", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private AccountSubscription(java.lang.String name, Output id, @Nullable AccountSubscriptionState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:quicksight/accountSubscription:AccountSubscription", name, state, makeResourceOptions(options, id), false);
}
private static AccountSubscriptionArgs makeArgs(AccountSubscriptionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? AccountSubscriptionArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static AccountSubscription get(java.lang.String name, Output id, @Nullable AccountSubscriptionState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new AccountSubscription(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy