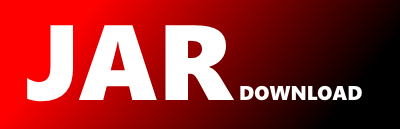
com.pulumi.aws.rds.SnapshotCopy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.rds;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.rds.SnapshotCopyArgs;
import com.pulumi.aws.rds.inputs.SnapshotCopyState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages an RDS database instance snapshot copy. For managing RDS database cluster snapshots, see the `aws.rds.ClusterSnapshot` resource.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.Instance;
* import com.pulumi.aws.rds.InstanceArgs;
* import com.pulumi.aws.rds.Snapshot;
* import com.pulumi.aws.rds.SnapshotArgs;
* import com.pulumi.aws.rds.SnapshotCopy;
* import com.pulumi.aws.rds.SnapshotCopyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Instance("example", InstanceArgs.builder()
* .allocatedStorage(10)
* .engine("mysql")
* .engineVersion("5.6.21")
* .instanceClass("db.t2.micro")
* .dbName("baz")
* .password("barbarbarbar")
* .username("foo")
* .maintenanceWindow("Fri:09:00-Fri:09:30")
* .backupRetentionPeriod(0)
* .parameterGroupName("default.mysql5.6")
* .build());
*
* var exampleSnapshot = new Snapshot("exampleSnapshot", SnapshotArgs.builder()
* .dbInstanceIdentifier(example.identifier())
* .dbSnapshotIdentifier("testsnapshot1234")
* .build());
*
* var exampleSnapshotCopy = new SnapshotCopy("exampleSnapshotCopy", SnapshotCopyArgs.builder()
* .sourceDbSnapshotIdentifier(exampleSnapshot.dbSnapshotArn())
* .targetDbSnapshotIdentifier("testsnapshot1234-copy")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import `aws_db_snapshot_copy` using the snapshot identifier. For example:
*
* ```sh
* $ pulumi import aws:rds/snapshotCopy:SnapshotCopy example my-snapshot
* ```
*
*/
@ResourceType(type="aws:rds/snapshotCopy:SnapshotCopy")
public class SnapshotCopy extends com.pulumi.resources.CustomResource {
/**
* Specifies the allocated storage size in gigabytes (GB).
*
*/
@Export(name="allocatedStorage", refs={Integer.class}, tree="[0]")
private Output allocatedStorage;
/**
* @return Specifies the allocated storage size in gigabytes (GB).
*
*/
public Output allocatedStorage() {
return this.allocatedStorage;
}
/**
* Specifies the name of the Availability Zone the DB instance was located in at the time of the DB snapshot.
*
*/
@Export(name="availabilityZone", refs={String.class}, tree="[0]")
private Output availabilityZone;
/**
* @return Specifies the name of the Availability Zone the DB instance was located in at the time of the DB snapshot.
*
*/
public Output availabilityZone() {
return this.availabilityZone;
}
/**
* Whether to copy existing tags. Defaults to `false`.
*
*/
@Export(name="copyTags", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> copyTags;
/**
* @return Whether to copy existing tags. Defaults to `false`.
*
*/
public Output> copyTags() {
return Codegen.optional(this.copyTags);
}
/**
* The Amazon Resource Name (ARN) for the DB snapshot.
*
*/
@Export(name="dbSnapshotArn", refs={String.class}, tree="[0]")
private Output dbSnapshotArn;
/**
* @return The Amazon Resource Name (ARN) for the DB snapshot.
*
*/
public Output dbSnapshotArn() {
return this.dbSnapshotArn;
}
/**
* The Destination region to place snapshot copy.
*
*/
@Export(name="destinationRegion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> destinationRegion;
/**
* @return The Destination region to place snapshot copy.
*
*/
public Output> destinationRegion() {
return Codegen.optional(this.destinationRegion);
}
/**
* Specifies whether the DB snapshot is encrypted.
*
*/
@Export(name="encrypted", refs={Boolean.class}, tree="[0]")
private Output encrypted;
/**
* @return Specifies whether the DB snapshot is encrypted.
*
*/
public Output encrypted() {
return this.encrypted;
}
/**
* Specifies the name of the database engine.
*
*/
@Export(name="engine", refs={String.class}, tree="[0]")
private Output engine;
/**
* @return Specifies the name of the database engine.
*
*/
public Output engine() {
return this.engine;
}
/**
* Specifies the version of the database engine.
*
*/
@Export(name="engineVersion", refs={String.class}, tree="[0]")
private Output engineVersion;
/**
* @return Specifies the version of the database engine.
*
*/
public Output engineVersion() {
return this.engineVersion;
}
/**
* Specifies the Provisioned IOPS (I/O operations per second) value of the DB instance at the time of the snapshot.
*
*/
@Export(name="iops", refs={Integer.class}, tree="[0]")
private Output iops;
/**
* @return Specifies the Provisioned IOPS (I/O operations per second) value of the DB instance at the time of the snapshot.
*
*/
public Output iops() {
return this.iops;
}
/**
* KMS key ID.
*
*/
@Export(name="kmsKeyId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> kmsKeyId;
/**
* @return KMS key ID.
*
*/
public Output> kmsKeyId() {
return Codegen.optional(this.kmsKeyId);
}
/**
* License model information for the restored DB instance.
*
*/
@Export(name="licenseModel", refs={String.class}, tree="[0]")
private Output licenseModel;
/**
* @return License model information for the restored DB instance.
*
*/
public Output licenseModel() {
return this.licenseModel;
}
/**
* The name of an option group to associate with the copy of the snapshot.
*
*/
@Export(name="optionGroupName", refs={String.class}, tree="[0]")
private Output optionGroupName;
/**
* @return The name of an option group to associate with the copy of the snapshot.
*
*/
public Output optionGroupName() {
return this.optionGroupName;
}
@Export(name="port", refs={Integer.class}, tree="[0]")
private Output port;
public Output port() {
return this.port;
}
/**
* he URL that contains a Signature Version 4 signed request.
*
*/
@Export(name="presignedUrl", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> presignedUrl;
/**
* @return he URL that contains a Signature Version 4 signed request.
*
*/
public Output> presignedUrl() {
return Codegen.optional(this.presignedUrl);
}
/**
* (Optional) List of AWS Account ids to share snapshot with, use `all` to make snaphot public.
*
*/
@Export(name="sharedAccounts", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> sharedAccounts;
/**
* @return (Optional) List of AWS Account ids to share snapshot with, use `all` to make snaphot public.
*
*/
public Output>> sharedAccounts() {
return Codegen.optional(this.sharedAccounts);
}
@Export(name="snapshotType", refs={String.class}, tree="[0]")
private Output snapshotType;
public Output snapshotType() {
return this.snapshotType;
}
/**
* Snapshot identifier of the source snapshot.
*
*/
@Export(name="sourceDbSnapshotIdentifier", refs={String.class}, tree="[0]")
private Output sourceDbSnapshotIdentifier;
/**
* @return Snapshot identifier of the source snapshot.
*
*/
public Output sourceDbSnapshotIdentifier() {
return this.sourceDbSnapshotIdentifier;
}
/**
* The region that the DB snapshot was created in or copied from.
*
*/
@Export(name="sourceRegion", refs={String.class}, tree="[0]")
private Output sourceRegion;
/**
* @return The region that the DB snapshot was created in or copied from.
*
*/
public Output sourceRegion() {
return this.sourceRegion;
}
/**
* Specifies the storage type associated with DB snapshot.
*
*/
@Export(name="storageType", refs={String.class}, tree="[0]")
private Output storageType;
/**
* @return Specifies the storage type associated with DB snapshot.
*
*/
public Output storageType() {
return this.storageType;
}
/**
* Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy