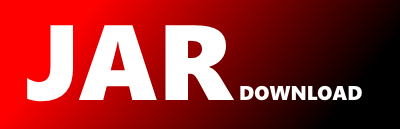
com.pulumi.aws.rds.outputs.ProxyDefaultTargetGroupConnectionPoolConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.rds.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ProxyDefaultTargetGroupConnectionPoolConfig {
/**
* @return The number of seconds for a proxy to wait for a connection to become available in the connection pool. Only applies when the proxy has opened its maximum number of connections and all connections are busy with client sessions.
*
*/
private @Nullable Integer connectionBorrowTimeout;
/**
* @return One or more SQL statements for the proxy to run when opening each new database connection. Typically used with `SET` statements to make sure that each connection has identical settings such as time zone and character set. This setting is empty by default. For multiple statements, use semicolons as the separator. You can also include multiple variables in a single `SET` statement, such as `SET x=1, y=2`.
*
*/
private @Nullable String initQuery;
/**
* @return The maximum size of the connection pool for each target in a target group. For Aurora MySQL, it is expressed as a percentage of the max_connections setting for the RDS DB instance or Aurora DB cluster used by the target group.
*
*/
private @Nullable Integer maxConnectionsPercent;
/**
* @return Controls how actively the proxy closes idle database connections in the connection pool. A high value enables the proxy to leave a high percentage of idle connections open. A low value causes the proxy to close idle client connections and return the underlying database connections to the connection pool. For Aurora MySQL, it is expressed as a percentage of the max_connections setting for the RDS DB instance or Aurora DB cluster used by the target group.
*
*/
private @Nullable Integer maxIdleConnectionsPercent;
/**
* @return Each item in the list represents a class of SQL operations that normally cause all later statements in a session using a proxy to be pinned to the same underlying database connection. Including an item in the list exempts that class of SQL operations from the pinning behavior. This setting is only supported for MySQL engine family databases. Currently, the only allowed value is `EXCLUDE_VARIABLE_SETS`.
*
*/
private @Nullable List sessionPinningFilters;
private ProxyDefaultTargetGroupConnectionPoolConfig() {}
/**
* @return The number of seconds for a proxy to wait for a connection to become available in the connection pool. Only applies when the proxy has opened its maximum number of connections and all connections are busy with client sessions.
*
*/
public Optional connectionBorrowTimeout() {
return Optional.ofNullable(this.connectionBorrowTimeout);
}
/**
* @return One or more SQL statements for the proxy to run when opening each new database connection. Typically used with `SET` statements to make sure that each connection has identical settings such as time zone and character set. This setting is empty by default. For multiple statements, use semicolons as the separator. You can also include multiple variables in a single `SET` statement, such as `SET x=1, y=2`.
*
*/
public Optional initQuery() {
return Optional.ofNullable(this.initQuery);
}
/**
* @return The maximum size of the connection pool for each target in a target group. For Aurora MySQL, it is expressed as a percentage of the max_connections setting for the RDS DB instance or Aurora DB cluster used by the target group.
*
*/
public Optional maxConnectionsPercent() {
return Optional.ofNullable(this.maxConnectionsPercent);
}
/**
* @return Controls how actively the proxy closes idle database connections in the connection pool. A high value enables the proxy to leave a high percentage of idle connections open. A low value causes the proxy to close idle client connections and return the underlying database connections to the connection pool. For Aurora MySQL, it is expressed as a percentage of the max_connections setting for the RDS DB instance or Aurora DB cluster used by the target group.
*
*/
public Optional maxIdleConnectionsPercent() {
return Optional.ofNullable(this.maxIdleConnectionsPercent);
}
/**
* @return Each item in the list represents a class of SQL operations that normally cause all later statements in a session using a proxy to be pinned to the same underlying database connection. Including an item in the list exempts that class of SQL operations from the pinning behavior. This setting is only supported for MySQL engine family databases. Currently, the only allowed value is `EXCLUDE_VARIABLE_SETS`.
*
*/
public List sessionPinningFilters() {
return this.sessionPinningFilters == null ? List.of() : this.sessionPinningFilters;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ProxyDefaultTargetGroupConnectionPoolConfig defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer connectionBorrowTimeout;
private @Nullable String initQuery;
private @Nullable Integer maxConnectionsPercent;
private @Nullable Integer maxIdleConnectionsPercent;
private @Nullable List sessionPinningFilters;
public Builder() {}
public Builder(ProxyDefaultTargetGroupConnectionPoolConfig defaults) {
Objects.requireNonNull(defaults);
this.connectionBorrowTimeout = defaults.connectionBorrowTimeout;
this.initQuery = defaults.initQuery;
this.maxConnectionsPercent = defaults.maxConnectionsPercent;
this.maxIdleConnectionsPercent = defaults.maxIdleConnectionsPercent;
this.sessionPinningFilters = defaults.sessionPinningFilters;
}
@CustomType.Setter
public Builder connectionBorrowTimeout(@Nullable Integer connectionBorrowTimeout) {
this.connectionBorrowTimeout = connectionBorrowTimeout;
return this;
}
@CustomType.Setter
public Builder initQuery(@Nullable String initQuery) {
this.initQuery = initQuery;
return this;
}
@CustomType.Setter
public Builder maxConnectionsPercent(@Nullable Integer maxConnectionsPercent) {
this.maxConnectionsPercent = maxConnectionsPercent;
return this;
}
@CustomType.Setter
public Builder maxIdleConnectionsPercent(@Nullable Integer maxIdleConnectionsPercent) {
this.maxIdleConnectionsPercent = maxIdleConnectionsPercent;
return this;
}
@CustomType.Setter
public Builder sessionPinningFilters(@Nullable List sessionPinningFilters) {
this.sessionPinningFilters = sessionPinningFilters;
return this;
}
public Builder sessionPinningFilters(String... sessionPinningFilters) {
return sessionPinningFilters(List.of(sessionPinningFilters));
}
public ProxyDefaultTargetGroupConnectionPoolConfig build() {
final var _resultValue = new ProxyDefaultTargetGroupConnectionPoolConfig();
_resultValue.connectionBorrowTimeout = connectionBorrowTimeout;
_resultValue.initQuery = initQuery;
_resultValue.maxConnectionsPercent = maxConnectionsPercent;
_resultValue.maxIdleConnectionsPercent = maxIdleConnectionsPercent;
_resultValue.sessionPinningFilters = sessionPinningFilters;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy