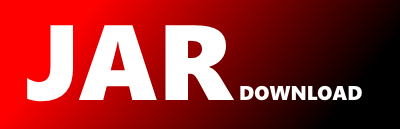
com.pulumi.aws.redshift.inputs.EndpointAuthorizationState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.redshift.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class EndpointAuthorizationState extends com.pulumi.resources.ResourceArgs {
public static final EndpointAuthorizationState Empty = new EndpointAuthorizationState();
/**
* The Amazon Web Services account ID to grant access to.
*
*/
@Import(name="account")
private @Nullable Output account;
/**
* @return The Amazon Web Services account ID to grant access to.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy