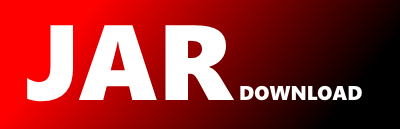
com.pulumi.aws.rum.inputs.AppMonitorState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.rum.inputs;
import com.pulumi.aws.rum.inputs.AppMonitorAppMonitorConfigurationArgs;
import com.pulumi.aws.rum.inputs.AppMonitorCustomEventsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AppMonitorState extends com.pulumi.resources.ResourceArgs {
public static final AppMonitorState Empty = new AppMonitorState();
/**
* configuration data for the app monitor. See app_monitor_configuration below.
*
*/
@Import(name="appMonitorConfiguration")
private @Nullable Output appMonitorConfiguration;
/**
* @return configuration data for the app monitor. See app_monitor_configuration below.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy