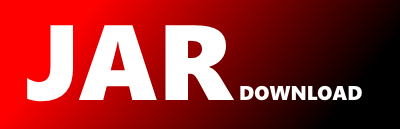
com.pulumi.aws.s3.AccessPoint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.s3;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.s3.AccessPointArgs;
import com.pulumi.aws.s3.inputs.AccessPointState;
import com.pulumi.aws.s3.outputs.AccessPointPublicAccessBlockConfiguration;
import com.pulumi.aws.s3.outputs.AccessPointVpcConfiguration;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a resource to manage an S3 Access Point.
*
* > **NOTE on Access Points and Access Point Policies:** This provider provides both a standalone Access Point Policy resource and an Access Point resource with a resource policy defined in-line. You cannot use an Access Point with in-line resource policy in conjunction with an Access Point Policy resource. Doing so will cause a conflict of policies and will overwrite the access point's resource policy.
*
* > Advanced usage: To use a custom API endpoint for this resource, use the `s3control` endpoint provider configuration), not the `s3` endpoint provider configuration.
*
* > This resource cannot be used with S3 directory buckets.
*
* ## Example Usage
*
* ### AWS Partition General Purpose Bucket
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.s3.AccessPoint;
* import com.pulumi.aws.s3.AccessPointArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new BucketV2("example", BucketV2Args.builder()
* .bucket("example")
* .build());
*
* var exampleAccessPoint = new AccessPoint("exampleAccessPoint", AccessPointArgs.builder()
* .bucket(example.id())
* .name("example")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### S3 on Outposts Bucket
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3control.Bucket;
* import com.pulumi.aws.s3control.BucketArgs;
* import com.pulumi.aws.ec2.Vpc;
* import com.pulumi.aws.ec2.VpcArgs;
* import com.pulumi.aws.s3.AccessPoint;
* import com.pulumi.aws.s3.AccessPointArgs;
* import com.pulumi.aws.s3.inputs.AccessPointVpcConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Bucket("example", BucketArgs.builder()
* .bucket("example")
* .build());
*
* var exampleVpc = new Vpc("exampleVpc", VpcArgs.builder()
* .cidrBlock("10.0.0.0/16")
* .build());
*
* var exampleAccessPoint = new AccessPoint("exampleAccessPoint", AccessPointArgs.builder()
* .bucket(example.arn())
* .name("example")
* .vpcConfiguration(AccessPointVpcConfigurationArgs.builder()
* .vpcId(exampleVpc.id())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Import using the ARN for Access Points associated with an S3 on Outposts Bucket:
*
* __Using `pulumi import` to import.__ For example:
*
* Import using the `account_id` and `name` separated by a colon (`:`) for Access Points associated with an AWS Partition S3 Bucket:
*
* ```sh
* $ pulumi import aws:s3/accessPoint:AccessPoint example 123456789012:example
* ```
* Import using the ARN for Access Points associated with an S3 on Outposts Bucket:
*
* ```sh
* $ pulumi import aws:s3/accessPoint:AccessPoint example arn:aws:s3-outposts:us-east-1:123456789012:outpost/op-1234567890123456/accesspoint/example
* ```
*
*/
@ResourceType(type="aws:s3/accessPoint:AccessPoint")
public class AccessPoint extends com.pulumi.resources.CustomResource {
/**
* AWS account ID for the owner of the bucket for which you want to create an access point. Defaults to automatically determined account ID of the AWS provider.
*
*/
@Export(name="accountId", refs={String.class}, tree="[0]")
private Output accountId;
/**
* @return AWS account ID for the owner of the bucket for which you want to create an access point. Defaults to automatically determined account ID of the AWS provider.
*
*/
public Output accountId() {
return this.accountId;
}
/**
* Alias of the S3 Access Point.
*
*/
@Export(name="alias", refs={String.class}, tree="[0]")
private Output alias;
/**
* @return Alias of the S3 Access Point.
*
*/
public Output alias() {
return this.alias;
}
/**
* ARN of the S3 Access Point.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the S3 Access Point.
*
*/
public Output arn() {
return this.arn;
}
/**
* Name of an AWS Partition S3 General Purpose Bucket or the ARN of S3 on Outposts Bucket that you want to associate this access point with.
*
*/
@Export(name="bucket", refs={String.class}, tree="[0]")
private Output bucket;
/**
* @return Name of an AWS Partition S3 General Purpose Bucket or the ARN of S3 on Outposts Bucket that you want to associate this access point with.
*
*/
public Output bucket() {
return this.bucket;
}
/**
* AWS account ID associated with the S3 bucket associated with this access point.
*
*/
@Export(name="bucketAccountId", refs={String.class}, tree="[0]")
private Output bucketAccountId;
/**
* @return AWS account ID associated with the S3 bucket associated with this access point.
*
*/
public Output bucketAccountId() {
return this.bucketAccountId;
}
/**
* DNS domain name of the S3 Access Point in the format _`name`_-_`account_id`_.s3-accesspoint._region_.amazonaws.com.
* Note: S3 access points only support secure access by HTTPS. HTTP isn't supported.
*
*/
@Export(name="domainName", refs={String.class}, tree="[0]")
private Output domainName;
/**
* @return DNS domain name of the S3 Access Point in the format _`name`_-_`account_id`_.s3-accesspoint._region_.amazonaws.com.
* Note: S3 access points only support secure access by HTTPS. HTTP isn't supported.
*
*/
public Output domainName() {
return this.domainName;
}
/**
* VPC endpoints for the S3 Access Point.
*
*/
@Export(name="endpoints", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy