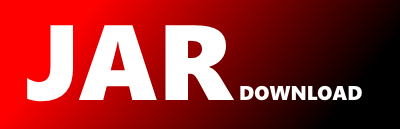
com.pulumi.aws.securitylake.inputs.SubscriberState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.securitylake.inputs;
import com.pulumi.aws.securitylake.inputs.SubscriberSourceArgs;
import com.pulumi.aws.securitylake.inputs.SubscriberSubscriberIdentityArgs;
import com.pulumi.aws.securitylake.inputs.SubscriberTimeoutsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SubscriberState extends com.pulumi.resources.ResourceArgs {
public static final SubscriberState Empty = new SubscriberState();
/**
* The Amazon S3 or Lake Formation access type.
*
*/
@Import(name="accessType")
private @Nullable Output accessType;
/**
* @return The Amazon S3 or Lake Formation access type.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy