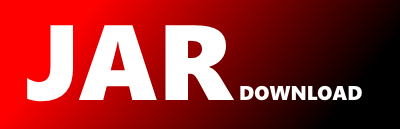
com.pulumi.aws.ssm.inputs.PatchBaselineApprovalRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ssm.inputs;
import com.pulumi.aws.ssm.inputs.PatchBaselineApprovalRulePatchFilterArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PatchBaselineApprovalRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final PatchBaselineApprovalRuleArgs Empty = new PatchBaselineApprovalRuleArgs();
/**
* Number of days after the release date of each patch matched by the rule the patch is marked as approved in the patch baseline. Valid Range: 0 to 100. Conflicts with `approve_until_date`.
*
*/
@Import(name="approveAfterDays")
private @Nullable Output approveAfterDays;
/**
* @return Number of days after the release date of each patch matched by the rule the patch is marked as approved in the patch baseline. Valid Range: 0 to 100. Conflicts with `approve_until_date`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy