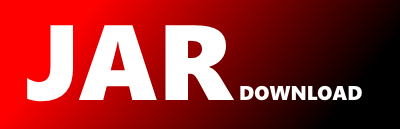
com.pulumi.aws.transfer.inputs.WorkflowOnExceptionStepDecryptStepDetailsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.transfer.inputs;
import com.pulumi.aws.transfer.inputs.WorkflowOnExceptionStepDecryptStepDetailsDestinationFileLocationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class WorkflowOnExceptionStepDecryptStepDetailsArgs extends com.pulumi.resources.ResourceArgs {
public static final WorkflowOnExceptionStepDecryptStepDetailsArgs Empty = new WorkflowOnExceptionStepDecryptStepDetailsArgs();
/**
* Specifies the location for the file being copied. Use ${Transfer:username} in this field to parametrize the destination prefix by username.
*
*/
@Import(name="destinationFileLocation")
private @Nullable Output destinationFileLocation;
/**
* @return Specifies the location for the file being copied. Use ${Transfer:username} in this field to parametrize the destination prefix by username.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy