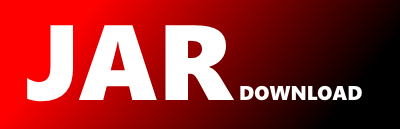
com.pulumi.aws.waf.outputs.ByteMatchSetByteMatchTuple Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.waf.outputs;
import com.pulumi.aws.waf.outputs.ByteMatchSetByteMatchTupleFieldToMatch;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ByteMatchSetByteMatchTuple {
/**
* @return The part of a web request that you want to search, such as a specified header or a query string.
*
*/
private ByteMatchSetByteMatchTupleFieldToMatch fieldToMatch;
/**
* @return Within the portion of a web request that you want to search
* (for example, in the query string, if any), specify where you want to search.
* e.g., `CONTAINS`, `CONTAINS_WORD` or `EXACTLY`.
* See [docs](http://docs.aws.amazon.com/waf/latest/APIReference/API_ByteMatchTuple.html#WAF-Type-ByteMatchTuple-PositionalConstraint)
* for all supported values.
*
*/
private String positionalConstraint;
/**
* @return The value that you want to search for within the field specified by `field_to_match`, e.g., `badrefer1`.
* See [docs](https://docs.aws.amazon.com/waf/latest/APIReference/API_waf_ByteMatchTuple.html)
* for all supported values.
*
*/
private @Nullable String targetString;
/**
* @return Text transformations used to eliminate unusual formatting that attackers use in web requests in an effort to bypass AWS WAF.
* If you specify a transformation, AWS WAF performs the transformation on `target_string` before inspecting a request for a match.
* e.g., `CMD_LINE`, `HTML_ENTITY_DECODE` or `NONE`.
* See [docs](http://docs.aws.amazon.com/waf/latest/APIReference/API_ByteMatchTuple.html#WAF-Type-ByteMatchTuple-TextTransformation)
* for all supported values.
*
*/
private String textTransformation;
private ByteMatchSetByteMatchTuple() {}
/**
* @return The part of a web request that you want to search, such as a specified header or a query string.
*
*/
public ByteMatchSetByteMatchTupleFieldToMatch fieldToMatch() {
return this.fieldToMatch;
}
/**
* @return Within the portion of a web request that you want to search
* (for example, in the query string, if any), specify where you want to search.
* e.g., `CONTAINS`, `CONTAINS_WORD` or `EXACTLY`.
* See [docs](http://docs.aws.amazon.com/waf/latest/APIReference/API_ByteMatchTuple.html#WAF-Type-ByteMatchTuple-PositionalConstraint)
* for all supported values.
*
*/
public String positionalConstraint() {
return this.positionalConstraint;
}
/**
* @return The value that you want to search for within the field specified by `field_to_match`, e.g., `badrefer1`.
* See [docs](https://docs.aws.amazon.com/waf/latest/APIReference/API_waf_ByteMatchTuple.html)
* for all supported values.
*
*/
public Optional targetString() {
return Optional.ofNullable(this.targetString);
}
/**
* @return Text transformations used to eliminate unusual formatting that attackers use in web requests in an effort to bypass AWS WAF.
* If you specify a transformation, AWS WAF performs the transformation on `target_string` before inspecting a request for a match.
* e.g., `CMD_LINE`, `HTML_ENTITY_DECODE` or `NONE`.
* See [docs](http://docs.aws.amazon.com/waf/latest/APIReference/API_ByteMatchTuple.html#WAF-Type-ByteMatchTuple-TextTransformation)
* for all supported values.
*
*/
public String textTransformation() {
return this.textTransformation;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ByteMatchSetByteMatchTuple defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private ByteMatchSetByteMatchTupleFieldToMatch fieldToMatch;
private String positionalConstraint;
private @Nullable String targetString;
private String textTransformation;
public Builder() {}
public Builder(ByteMatchSetByteMatchTuple defaults) {
Objects.requireNonNull(defaults);
this.fieldToMatch = defaults.fieldToMatch;
this.positionalConstraint = defaults.positionalConstraint;
this.targetString = defaults.targetString;
this.textTransformation = defaults.textTransformation;
}
@CustomType.Setter
public Builder fieldToMatch(ByteMatchSetByteMatchTupleFieldToMatch fieldToMatch) {
if (fieldToMatch == null) {
throw new MissingRequiredPropertyException("ByteMatchSetByteMatchTuple", "fieldToMatch");
}
this.fieldToMatch = fieldToMatch;
return this;
}
@CustomType.Setter
public Builder positionalConstraint(String positionalConstraint) {
if (positionalConstraint == null) {
throw new MissingRequiredPropertyException("ByteMatchSetByteMatchTuple", "positionalConstraint");
}
this.positionalConstraint = positionalConstraint;
return this;
}
@CustomType.Setter
public Builder targetString(@Nullable String targetString) {
this.targetString = targetString;
return this;
}
@CustomType.Setter
public Builder textTransformation(String textTransformation) {
if (textTransformation == null) {
throw new MissingRequiredPropertyException("ByteMatchSetByteMatchTuple", "textTransformation");
}
this.textTransformation = textTransformation;
return this;
}
public ByteMatchSetByteMatchTuple build() {
final var _resultValue = new ByteMatchSetByteMatchTuple();
_resultValue.fieldToMatch = fieldToMatch;
_resultValue.positionalConstraint = positionalConstraint;
_resultValue.targetString = targetString;
_resultValue.textTransformation = textTransformation;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy