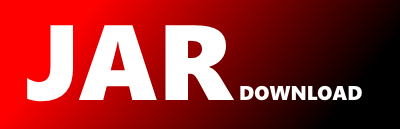
com.pulumi.aws.wafregional.inputs.SizeConstraintSetSizeConstraintArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.wafregional.inputs;
import com.pulumi.aws.wafregional.inputs.SizeConstraintSetSizeConstraintFieldToMatchArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
public final class SizeConstraintSetSizeConstraintArgs extends com.pulumi.resources.ResourceArgs {
public static final SizeConstraintSetSizeConstraintArgs Empty = new SizeConstraintSetSizeConstraintArgs();
/**
* The type of comparison you want to perform.
* e.g., `EQ`, `NE`, `LT`, `GT`.
* See [docs](https://docs.aws.amazon.com/waf/latest/APIReference/API_wafRegional_SizeConstraint.html) for all supported values.
*
*/
@Import(name="comparisonOperator", required=true)
private Output comparisonOperator;
/**
* @return The type of comparison you want to perform.
* e.g., `EQ`, `NE`, `LT`, `GT`.
* See [docs](https://docs.aws.amazon.com/waf/latest/APIReference/API_wafRegional_SizeConstraint.html) for all supported values.
*
*/
public Output comparisonOperator() {
return this.comparisonOperator;
}
/**
* Specifies where in a web request to look for the size constraint.
*
*/
@Import(name="fieldToMatch", required=true)
private Output fieldToMatch;
/**
* @return Specifies where in a web request to look for the size constraint.
*
*/
public Output fieldToMatch() {
return this.fieldToMatch;
}
/**
* The size in bytes that you want to compare against the size of the specified `field_to_match`.
* Valid values are between 0 - 21474836480 bytes (0 - 20 GB).
*
*/
@Import(name="size", required=true)
private Output size;
/**
* @return The size in bytes that you want to compare against the size of the specified `field_to_match`.
* Valid values are between 0 - 21474836480 bytes (0 - 20 GB).
*
*/
public Output size() {
return this.size;
}
/**
* Text transformations used to eliminate unusual formatting that attackers use in web requests in an effort to bypass AWS WAF.
* If you specify a transformation, AWS WAF performs the transformation on `field_to_match` before inspecting a request for a match.
* e.g., `CMD_LINE`, `HTML_ENTITY_DECODE` or `NONE`.
* See [docs](http://docs.aws.amazon.com/waf/latest/APIReference/API_SizeConstraint.html#WAF-Type-SizeConstraint-TextTransformation)
* for all supported values.
* **Note:** if you choose `BODY` as `type`, you must choose `NONE` because CloudFront forwards only the first 8192 bytes for inspection.
*
*/
@Import(name="textTransformation", required=true)
private Output textTransformation;
/**
* @return Text transformations used to eliminate unusual formatting that attackers use in web requests in an effort to bypass AWS WAF.
* If you specify a transformation, AWS WAF performs the transformation on `field_to_match` before inspecting a request for a match.
* e.g., `CMD_LINE`, `HTML_ENTITY_DECODE` or `NONE`.
* See [docs](http://docs.aws.amazon.com/waf/latest/APIReference/API_SizeConstraint.html#WAF-Type-SizeConstraint-TextTransformation)
* for all supported values.
* **Note:** if you choose `BODY` as `type`, you must choose `NONE` because CloudFront forwards only the first 8192 bytes for inspection.
*
*/
public Output textTransformation() {
return this.textTransformation;
}
private SizeConstraintSetSizeConstraintArgs() {}
private SizeConstraintSetSizeConstraintArgs(SizeConstraintSetSizeConstraintArgs $) {
this.comparisonOperator = $.comparisonOperator;
this.fieldToMatch = $.fieldToMatch;
this.size = $.size;
this.textTransformation = $.textTransformation;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SizeConstraintSetSizeConstraintArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private SizeConstraintSetSizeConstraintArgs $;
public Builder() {
$ = new SizeConstraintSetSizeConstraintArgs();
}
public Builder(SizeConstraintSetSizeConstraintArgs defaults) {
$ = new SizeConstraintSetSizeConstraintArgs(Objects.requireNonNull(defaults));
}
/**
* @param comparisonOperator The type of comparison you want to perform.
* e.g., `EQ`, `NE`, `LT`, `GT`.
* See [docs](https://docs.aws.amazon.com/waf/latest/APIReference/API_wafRegional_SizeConstraint.html) for all supported values.
*
* @return builder
*
*/
public Builder comparisonOperator(Output comparisonOperator) {
$.comparisonOperator = comparisonOperator;
return this;
}
/**
* @param comparisonOperator The type of comparison you want to perform.
* e.g., `EQ`, `NE`, `LT`, `GT`.
* See [docs](https://docs.aws.amazon.com/waf/latest/APIReference/API_wafRegional_SizeConstraint.html) for all supported values.
*
* @return builder
*
*/
public Builder comparisonOperator(String comparisonOperator) {
return comparisonOperator(Output.of(comparisonOperator));
}
/**
* @param fieldToMatch Specifies where in a web request to look for the size constraint.
*
* @return builder
*
*/
public Builder fieldToMatch(Output fieldToMatch) {
$.fieldToMatch = fieldToMatch;
return this;
}
/**
* @param fieldToMatch Specifies where in a web request to look for the size constraint.
*
* @return builder
*
*/
public Builder fieldToMatch(SizeConstraintSetSizeConstraintFieldToMatchArgs fieldToMatch) {
return fieldToMatch(Output.of(fieldToMatch));
}
/**
* @param size The size in bytes that you want to compare against the size of the specified `field_to_match`.
* Valid values are between 0 - 21474836480 bytes (0 - 20 GB).
*
* @return builder
*
*/
public Builder size(Output size) {
$.size = size;
return this;
}
/**
* @param size The size in bytes that you want to compare against the size of the specified `field_to_match`.
* Valid values are between 0 - 21474836480 bytes (0 - 20 GB).
*
* @return builder
*
*/
public Builder size(Integer size) {
return size(Output.of(size));
}
/**
* @param textTransformation Text transformations used to eliminate unusual formatting that attackers use in web requests in an effort to bypass AWS WAF.
* If you specify a transformation, AWS WAF performs the transformation on `field_to_match` before inspecting a request for a match.
* e.g., `CMD_LINE`, `HTML_ENTITY_DECODE` or `NONE`.
* See [docs](http://docs.aws.amazon.com/waf/latest/APIReference/API_SizeConstraint.html#WAF-Type-SizeConstraint-TextTransformation)
* for all supported values.
* **Note:** if you choose `BODY` as `type`, you must choose `NONE` because CloudFront forwards only the first 8192 bytes for inspection.
*
* @return builder
*
*/
public Builder textTransformation(Output textTransformation) {
$.textTransformation = textTransformation;
return this;
}
/**
* @param textTransformation Text transformations used to eliminate unusual formatting that attackers use in web requests in an effort to bypass AWS WAF.
* If you specify a transformation, AWS WAF performs the transformation on `field_to_match` before inspecting a request for a match.
* e.g., `CMD_LINE`, `HTML_ENTITY_DECODE` or `NONE`.
* See [docs](http://docs.aws.amazon.com/waf/latest/APIReference/API_SizeConstraint.html#WAF-Type-SizeConstraint-TextTransformation)
* for all supported values.
* **Note:** if you choose `BODY` as `type`, you must choose `NONE` because CloudFront forwards only the first 8192 bytes for inspection.
*
* @return builder
*
*/
public Builder textTransformation(String textTransformation) {
return textTransformation(Output.of(textTransformation));
}
public SizeConstraintSetSizeConstraintArgs build() {
if ($.comparisonOperator == null) {
throw new MissingRequiredPropertyException("SizeConstraintSetSizeConstraintArgs", "comparisonOperator");
}
if ($.fieldToMatch == null) {
throw new MissingRequiredPropertyException("SizeConstraintSetSizeConstraintArgs", "fieldToMatch");
}
if ($.size == null) {
throw new MissingRequiredPropertyException("SizeConstraintSetSizeConstraintArgs", "size");
}
if ($.textTransformation == null) {
throw new MissingRequiredPropertyException("SizeConstraintSetSizeConstraintArgs", "textTransformation");
}
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy