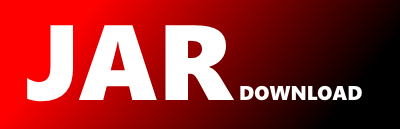
com.pulumi.aws.workspaces.WorkspaceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.workspaces;
import com.pulumi.aws.workspaces.inputs.WorkspaceWorkspacePropertiesArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class WorkspaceArgs extends com.pulumi.resources.ResourceArgs {
public static final WorkspaceArgs Empty = new WorkspaceArgs();
/**
* The ID of the bundle for the WorkSpace.
*
*/
@Import(name="bundleId", required=true)
private Output bundleId;
/**
* @return The ID of the bundle for the WorkSpace.
*
*/
public Output bundleId() {
return this.bundleId;
}
/**
* The ID of the directory for the WorkSpace.
*
*/
@Import(name="directoryId", required=true)
private Output directoryId;
/**
* @return The ID of the directory for the WorkSpace.
*
*/
public Output directoryId() {
return this.directoryId;
}
/**
* Indicates whether the data stored on the root volume is encrypted.
*
*/
@Import(name="rootVolumeEncryptionEnabled")
private @Nullable Output rootVolumeEncryptionEnabled;
/**
* @return Indicates whether the data stored on the root volume is encrypted.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy