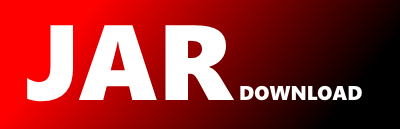
com.pulumi.aws.workspaces.outputs.GetDirectoryWorkspaceAccessProperty Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.workspaces.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetDirectoryWorkspaceAccessProperty {
/**
* @return (Optional) Indicates whether users can use Android devices to access their WorkSpaces.
*
*/
private String deviceTypeAndroid;
/**
* @return (Optional) Indicates whether users can use Chromebooks to access their WorkSpaces.
*
*/
private String deviceTypeChromeos;
/**
* @return (Optional) Indicates whether users can use iOS devices to access their WorkSpaces.
*
*/
private String deviceTypeIos;
/**
* @return (Optional) Indicates whether users can use Linux clients to access their WorkSpaces.
*
*/
private String deviceTypeLinux;
/**
* @return (Optional) Indicates whether users can use macOS clients to access their WorkSpaces.
*
*/
private String deviceTypeOsx;
/**
* @return (Optional) Indicates whether users can access their WorkSpaces through a web browser.
*
*/
private String deviceTypeWeb;
/**
* @return (Optional) Indicates whether users can use Windows clients to access their WorkSpaces.
*
*/
private String deviceTypeWindows;
/**
* @return (Optional) Indicates whether users can use zero client devices to access their WorkSpaces.
*
*/
private String deviceTypeZeroclient;
private GetDirectoryWorkspaceAccessProperty() {}
/**
* @return (Optional) Indicates whether users can use Android devices to access their WorkSpaces.
*
*/
public String deviceTypeAndroid() {
return this.deviceTypeAndroid;
}
/**
* @return (Optional) Indicates whether users can use Chromebooks to access their WorkSpaces.
*
*/
public String deviceTypeChromeos() {
return this.deviceTypeChromeos;
}
/**
* @return (Optional) Indicates whether users can use iOS devices to access their WorkSpaces.
*
*/
public String deviceTypeIos() {
return this.deviceTypeIos;
}
/**
* @return (Optional) Indicates whether users can use Linux clients to access their WorkSpaces.
*
*/
public String deviceTypeLinux() {
return this.deviceTypeLinux;
}
/**
* @return (Optional) Indicates whether users can use macOS clients to access their WorkSpaces.
*
*/
public String deviceTypeOsx() {
return this.deviceTypeOsx;
}
/**
* @return (Optional) Indicates whether users can access their WorkSpaces through a web browser.
*
*/
public String deviceTypeWeb() {
return this.deviceTypeWeb;
}
/**
* @return (Optional) Indicates whether users can use Windows clients to access their WorkSpaces.
*
*/
public String deviceTypeWindows() {
return this.deviceTypeWindows;
}
/**
* @return (Optional) Indicates whether users can use zero client devices to access their WorkSpaces.
*
*/
public String deviceTypeZeroclient() {
return this.deviceTypeZeroclient;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDirectoryWorkspaceAccessProperty defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String deviceTypeAndroid;
private String deviceTypeChromeos;
private String deviceTypeIos;
private String deviceTypeLinux;
private String deviceTypeOsx;
private String deviceTypeWeb;
private String deviceTypeWindows;
private String deviceTypeZeroclient;
public Builder() {}
public Builder(GetDirectoryWorkspaceAccessProperty defaults) {
Objects.requireNonNull(defaults);
this.deviceTypeAndroid = defaults.deviceTypeAndroid;
this.deviceTypeChromeos = defaults.deviceTypeChromeos;
this.deviceTypeIos = defaults.deviceTypeIos;
this.deviceTypeLinux = defaults.deviceTypeLinux;
this.deviceTypeOsx = defaults.deviceTypeOsx;
this.deviceTypeWeb = defaults.deviceTypeWeb;
this.deviceTypeWindows = defaults.deviceTypeWindows;
this.deviceTypeZeroclient = defaults.deviceTypeZeroclient;
}
@CustomType.Setter
public Builder deviceTypeAndroid(String deviceTypeAndroid) {
if (deviceTypeAndroid == null) {
throw new MissingRequiredPropertyException("GetDirectoryWorkspaceAccessProperty", "deviceTypeAndroid");
}
this.deviceTypeAndroid = deviceTypeAndroid;
return this;
}
@CustomType.Setter
public Builder deviceTypeChromeos(String deviceTypeChromeos) {
if (deviceTypeChromeos == null) {
throw new MissingRequiredPropertyException("GetDirectoryWorkspaceAccessProperty", "deviceTypeChromeos");
}
this.deviceTypeChromeos = deviceTypeChromeos;
return this;
}
@CustomType.Setter
public Builder deviceTypeIos(String deviceTypeIos) {
if (deviceTypeIos == null) {
throw new MissingRequiredPropertyException("GetDirectoryWorkspaceAccessProperty", "deviceTypeIos");
}
this.deviceTypeIos = deviceTypeIos;
return this;
}
@CustomType.Setter
public Builder deviceTypeLinux(String deviceTypeLinux) {
if (deviceTypeLinux == null) {
throw new MissingRequiredPropertyException("GetDirectoryWorkspaceAccessProperty", "deviceTypeLinux");
}
this.deviceTypeLinux = deviceTypeLinux;
return this;
}
@CustomType.Setter
public Builder deviceTypeOsx(String deviceTypeOsx) {
if (deviceTypeOsx == null) {
throw new MissingRequiredPropertyException("GetDirectoryWorkspaceAccessProperty", "deviceTypeOsx");
}
this.deviceTypeOsx = deviceTypeOsx;
return this;
}
@CustomType.Setter
public Builder deviceTypeWeb(String deviceTypeWeb) {
if (deviceTypeWeb == null) {
throw new MissingRequiredPropertyException("GetDirectoryWorkspaceAccessProperty", "deviceTypeWeb");
}
this.deviceTypeWeb = deviceTypeWeb;
return this;
}
@CustomType.Setter
public Builder deviceTypeWindows(String deviceTypeWindows) {
if (deviceTypeWindows == null) {
throw new MissingRequiredPropertyException("GetDirectoryWorkspaceAccessProperty", "deviceTypeWindows");
}
this.deviceTypeWindows = deviceTypeWindows;
return this;
}
@CustomType.Setter
public Builder deviceTypeZeroclient(String deviceTypeZeroclient) {
if (deviceTypeZeroclient == null) {
throw new MissingRequiredPropertyException("GetDirectoryWorkspaceAccessProperty", "deviceTypeZeroclient");
}
this.deviceTypeZeroclient = deviceTypeZeroclient;
return this;
}
public GetDirectoryWorkspaceAccessProperty build() {
final var _resultValue = new GetDirectoryWorkspaceAccessProperty();
_resultValue.deviceTypeAndroid = deviceTypeAndroid;
_resultValue.deviceTypeChromeos = deviceTypeChromeos;
_resultValue.deviceTypeIos = deviceTypeIos;
_resultValue.deviceTypeLinux = deviceTypeLinux;
_resultValue.deviceTypeOsx = deviceTypeOsx;
_resultValue.deviceTypeWeb = deviceTypeWeb;
_resultValue.deviceTypeWindows = deviceTypeWindows;
_resultValue.deviceTypeZeroclient = deviceTypeZeroclient;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy