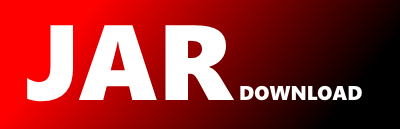
com.pulumi.aws.xray.SamplingRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.xray;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.xray.SamplingRuleArgs;
import com.pulumi.aws.xray.inputs.SamplingRuleState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Creates and manages an AWS XRay Sampling Rule.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.xray.SamplingRule;
* import com.pulumi.aws.xray.SamplingRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new SamplingRule("example", SamplingRuleArgs.builder()
* .ruleName("example")
* .priority(9999)
* .version(1)
* .reservoirSize(1)
* .fixedRate(0.05)
* .urlPath("*")
* .host("*")
* .httpMethod("*")
* .serviceType("*")
* .serviceName("*")
* .resourceArn("*")
* .attributes(Map.of("Hello", "Tris"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import XRay Sampling Rules using the name. For example:
*
* ```sh
* $ pulumi import aws:xray/samplingRule:SamplingRule example example
* ```
*
*/
@ResourceType(type="aws:xray/samplingRule:SamplingRule")
public class SamplingRule extends com.pulumi.resources.CustomResource {
/**
* The ARN of the sampling rule.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The ARN of the sampling rule.
*
*/
public Output arn() {
return this.arn;
}
/**
* Matches attributes derived from the request.
*
*/
@Export(name="attributes", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> attributes;
/**
* @return Matches attributes derived from the request.
*
*/
public Output>> attributes() {
return Codegen.optional(this.attributes);
}
/**
* The percentage of matching requests to instrument, after the reservoir is exhausted.
*
*/
@Export(name="fixedRate", refs={Double.class}, tree="[0]")
private Output fixedRate;
/**
* @return The percentage of matching requests to instrument, after the reservoir is exhausted.
*
*/
public Output fixedRate() {
return this.fixedRate;
}
/**
* Matches the hostname from a request URL.
*
*/
@Export(name="host", refs={String.class}, tree="[0]")
private Output host;
/**
* @return Matches the hostname from a request URL.
*
*/
public Output host() {
return this.host;
}
/**
* Matches the HTTP method of a request.
*
*/
@Export(name="httpMethod", refs={String.class}, tree="[0]")
private Output httpMethod;
/**
* @return Matches the HTTP method of a request.
*
*/
public Output httpMethod() {
return this.httpMethod;
}
/**
* The priority of the sampling rule.
*
*/
@Export(name="priority", refs={Integer.class}, tree="[0]")
private Output priority;
/**
* @return The priority of the sampling rule.
*
*/
public Output priority() {
return this.priority;
}
/**
* A fixed number of matching requests to instrument per second, prior to applying the fixed rate. The reservoir is not used directly by services, but applies to all services using the rule collectively.
*
*/
@Export(name="reservoirSize", refs={Integer.class}, tree="[0]")
private Output reservoirSize;
/**
* @return A fixed number of matching requests to instrument per second, prior to applying the fixed rate. The reservoir is not used directly by services, but applies to all services using the rule collectively.
*
*/
public Output reservoirSize() {
return this.reservoirSize;
}
/**
* Matches the ARN of the AWS resource on which the service runs.
*
*/
@Export(name="resourceArn", refs={String.class}, tree="[0]")
private Output resourceArn;
/**
* @return Matches the ARN of the AWS resource on which the service runs.
*
*/
public Output resourceArn() {
return this.resourceArn;
}
/**
* The name of the sampling rule.
*
*/
@Export(name="ruleName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> ruleName;
/**
* @return The name of the sampling rule.
*
*/
public Output> ruleName() {
return Codegen.optional(this.ruleName);
}
/**
* Matches the `name` that the service uses to identify itself in segments.
*
*/
@Export(name="serviceName", refs={String.class}, tree="[0]")
private Output serviceName;
/**
* @return Matches the `name` that the service uses to identify itself in segments.
*
*/
public Output serviceName() {
return this.serviceName;
}
/**
* Matches the `origin` that the service uses to identify its type in segments.
*
*/
@Export(name="serviceType", refs={String.class}, tree="[0]")
private Output serviceType;
/**
* @return Matches the `origin` that the service uses to identify its type in segments.
*
*/
public Output serviceType() {
return this.serviceType;
}
/**
* Key-value mapping of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value mapping of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy