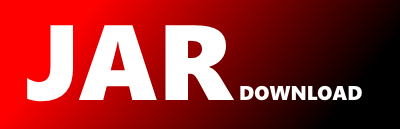
com.pulumi.aws.batch.JobQueue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.batch;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.batch.JobQueueArgs;
import com.pulumi.aws.batch.inputs.JobQueueState;
import com.pulumi.aws.batch.outputs.JobQueueComputeEnvironmentOrder;
import com.pulumi.aws.batch.outputs.JobQueueJobStateTimeLimitAction;
import com.pulumi.aws.batch.outputs.JobQueueTimeouts;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a Batch Job Queue resource.
*
* ## Example Usage
*
* ### Basic Job Queue
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.batch.JobQueue;
* import com.pulumi.aws.batch.JobQueueArgs;
* import com.pulumi.aws.batch.inputs.JobQueueComputeEnvironmentOrderArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var testQueue = new JobQueue("testQueue", JobQueueArgs.builder()
* .name("tf-test-batch-job-queue")
* .state("ENABLED")
* .priority(1)
* .computeEnvironmentOrders(
* JobQueueComputeEnvironmentOrderArgs.builder()
* .order(1)
* .computeEnvironment(testEnvironment1.arn())
* .build(),
* JobQueueComputeEnvironmentOrderArgs.builder()
* .order(2)
* .computeEnvironment(testEnvironment2.arn())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Job Queue with a fair share scheduling policy
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.batch.SchedulingPolicy;
* import com.pulumi.aws.batch.SchedulingPolicyArgs;
* import com.pulumi.aws.batch.inputs.SchedulingPolicyFairSharePolicyArgs;
* import com.pulumi.aws.batch.JobQueue;
* import com.pulumi.aws.batch.JobQueueArgs;
* import com.pulumi.aws.batch.inputs.JobQueueComputeEnvironmentOrderArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new SchedulingPolicy("example", SchedulingPolicyArgs.builder()
* .name("example")
* .fairSharePolicy(SchedulingPolicyFairSharePolicyArgs.builder()
* .computeReservation(1)
* .shareDecaySeconds(3600)
* .shareDistributions(SchedulingPolicyFairSharePolicyShareDistributionArgs.builder()
* .shareIdentifier("A1*")
* .weightFactor(0.1)
* .build())
* .build())
* .build());
*
* var exampleJobQueue = new JobQueue("exampleJobQueue", JobQueueArgs.builder()
* .name("tf-test-batch-job-queue")
* .schedulingPolicyArn(example.arn())
* .state("ENABLED")
* .priority(1)
* .computeEnvironmentOrders(
* JobQueueComputeEnvironmentOrderArgs.builder()
* .order(1)
* .computeEnvironment(testEnvironment1.arn())
* .build(),
* JobQueueComputeEnvironmentOrderArgs.builder()
* .order(2)
* .computeEnvironment(testEnvironment2.arn())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import Batch Job Queue using the `arn`. For example:
*
* ```sh
* $ pulumi import aws:batch/jobQueue:JobQueue test_queue arn:aws:batch:us-east-1:123456789012:job-queue/sample
* ```
*
*/
@ResourceType(type="aws:batch/jobQueue:JobQueue")
public class JobQueue extends com.pulumi.resources.CustomResource {
/**
* The Amazon Resource Name of the job queue.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The Amazon Resource Name of the job queue.
*
*/
public Output arn() {
return this.arn;
}
/**
* The set of compute environments mapped to a job queue and their order relative to each other. The job scheduler uses this parameter to determine which compute environment runs a specific job. Compute environments must be in the VALID state before you can associate them with a job queue. You can associate up to three compute environments with a job queue.
*
*/
@Export(name="computeEnvironmentOrders", refs={List.class,JobQueueComputeEnvironmentOrder.class}, tree="[0,1]")
private Output* @Nullable */ List> computeEnvironmentOrders;
/**
* @return The set of compute environments mapped to a job queue and their order relative to each other. The job scheduler uses this parameter to determine which compute environment runs a specific job. Compute environments must be in the VALID state before you can associate them with a job queue. You can associate up to three compute environments with a job queue.
*
*/
public Output>> computeEnvironmentOrders() {
return Codegen.optional(this.computeEnvironmentOrders);
}
/**
* (Optional) This parameter is deprecated, please use `compute_environment_order` instead. List of compute environment ARNs mapped to a job queue. The position of the compute environments in the list will dictate the order. When importing a AWS Batch Job Queue, the parameter `compute_environments` will always be used over `compute_environment_order`. Please adjust your HCL accordingly.
*
* @deprecated
* This parameter will be replaced by `compute_environment_order`.
*
*/
@Deprecated /* This parameter will be replaced by `compute_environment_order`. */
@Export(name="computeEnvironments", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> computeEnvironments;
/**
* @return (Optional) This parameter is deprecated, please use `compute_environment_order` instead. List of compute environment ARNs mapped to a job queue. The position of the compute environments in the list will dictate the order. When importing a AWS Batch Job Queue, the parameter `compute_environments` will always be used over `compute_environment_order`. Please adjust your HCL accordingly.
*
*/
public Output>> computeEnvironments() {
return Codegen.optional(this.computeEnvironments);
}
/**
* The set of job state time limit actions mapped to a job queue. Specifies an action that AWS Batch will take after the job has remained at the head of the queue in the specified state for longer than the specified time.
*
*/
@Export(name="jobStateTimeLimitActions", refs={List.class,JobQueueJobStateTimeLimitAction.class}, tree="[0,1]")
private Output* @Nullable */ List> jobStateTimeLimitActions;
/**
* @return The set of job state time limit actions mapped to a job queue. Specifies an action that AWS Batch will take after the job has remained at the head of the queue in the specified state for longer than the specified time.
*
*/
public Output>> jobStateTimeLimitActions() {
return Codegen.optional(this.jobStateTimeLimitActions);
}
/**
* Specifies the name of the job queue.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Specifies the name of the job queue.
*
*/
public Output name() {
return this.name;
}
/**
* The priority of the job queue. Job queues with a higher priority
* are evaluated first when associated with the same compute environment.
*
*/
@Export(name="priority", refs={Integer.class}, tree="[0]")
private Output priority;
/**
* @return The priority of the job queue. Job queues with a higher priority
* are evaluated first when associated with the same compute environment.
*
*/
public Output priority() {
return this.priority;
}
/**
* The ARN of the fair share scheduling policy. If this parameter is specified, the job queue uses a fair share scheduling policy. If this parameter isn't specified, the job queue uses a first in, first out (FIFO) scheduling policy. After a job queue is created, you can replace but can't remove the fair share scheduling policy.
*
*/
@Export(name="schedulingPolicyArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> schedulingPolicyArn;
/**
* @return The ARN of the fair share scheduling policy. If this parameter is specified, the job queue uses a fair share scheduling policy. If this parameter isn't specified, the job queue uses a first in, first out (FIFO) scheduling policy. After a job queue is created, you can replace but can't remove the fair share scheduling policy.
*
*/
public Output> schedulingPolicyArn() {
return Codegen.optional(this.schedulingPolicyArn);
}
/**
* The state of the job queue. Must be one of: `ENABLED` or `DISABLED`
*
*/
@Export(name="state", refs={String.class}, tree="[0]")
private Output state;
/**
* @return The state of the job queue. Must be one of: `ENABLED` or `DISABLED`
*
*/
public Output state() {
return this.state;
}
/**
* Key-value map of resource tags. .If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value map of resource tags. .If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy