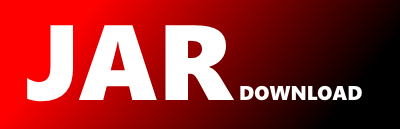
com.pulumi.aws.budgets.inputs.BudgetActionState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.budgets.inputs;
import com.pulumi.aws.budgets.inputs.BudgetActionActionThresholdArgs;
import com.pulumi.aws.budgets.inputs.BudgetActionDefinitionArgs;
import com.pulumi.aws.budgets.inputs.BudgetActionSubscriberArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BudgetActionState extends com.pulumi.resources.ResourceArgs {
public static final BudgetActionState Empty = new BudgetActionState();
/**
* The ID of the target account for budget. Will use current user's account_id by default if omitted.
*
*/
@Import(name="accountId")
private @Nullable Output accountId;
/**
* @return The ID of the target account for budget. Will use current user's account_id by default if omitted.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy