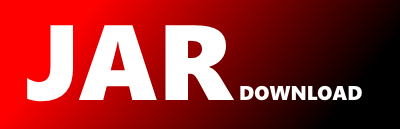
com.pulumi.aws.cloudcontrol.inputs.ResourceState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudcontrol.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ResourceState extends com.pulumi.resources.ResourceArgs {
public static final ResourceState Empty = new ResourceState();
/**
* JSON string matching the CloudFormation resource type schema with desired configuration.
*
*/
@Import(name="desiredState")
private @Nullable Output desiredState;
/**
* @return JSON string matching the CloudFormation resource type schema with desired configuration.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy