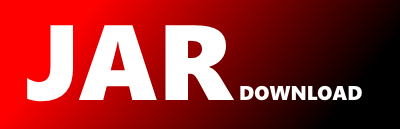
com.pulumi.aws.cloudformation.Stack Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudformation;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.cloudformation.StackArgs;
import com.pulumi.aws.cloudformation.inputs.StackState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a CloudFormation Stack resource.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudformation.Stack;
* import com.pulumi.aws.cloudformation.StackArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var network = new Stack("network", StackArgs.builder()
* .name("networking-stack")
* .parameters(Map.of("VPCCidr", "10.0.0.0/16"))
* .templateBody(serializeJson(
* jsonObject(
* jsonProperty("Parameters", jsonObject(
* jsonProperty("VPCCidr", jsonObject(
* jsonProperty("Type", "String"),
* jsonProperty("Default", "10.0.0.0/16"),
* jsonProperty("Description", "Enter the CIDR block for the VPC. Default is 10.0.0.0/16.")
* ))
* )),
* jsonProperty("Resources", jsonObject(
* jsonProperty("myVpc", jsonObject(
* jsonProperty("Type", "AWS::EC2::VPC"),
* jsonProperty("Properties", jsonObject(
* jsonProperty("CidrBlock", jsonObject(
* jsonProperty("Ref", "VPCCidr")
* )),
* jsonProperty("Tags", jsonArray(jsonObject(
* jsonProperty("Key", "Name"),
* jsonProperty("Value", "Primary_CF_VPC")
* )))
* ))
* ))
* ))
* )))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import Cloudformation Stacks using the `name`. For example:
*
* ```sh
* $ pulumi import aws:cloudformation/stack:Stack stack networking-stack
* ```
*
*/
@ResourceType(type="aws:cloudformation/stack:Stack")
public class Stack extends com.pulumi.resources.CustomResource {
/**
* A list of capabilities.
* Valid values: `CAPABILITY_IAM`, `CAPABILITY_NAMED_IAM`, or `CAPABILITY_AUTO_EXPAND`
*
*/
@Export(name="capabilities", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> capabilities;
/**
* @return A list of capabilities.
* Valid values: `CAPABILITY_IAM`, `CAPABILITY_NAMED_IAM`, or `CAPABILITY_AUTO_EXPAND`
*
*/
public Output>> capabilities() {
return Codegen.optional(this.capabilities);
}
/**
* Set to true to disable rollback of the stack if stack creation failed.
* Conflicts with `on_failure`.
*
*/
@Export(name="disableRollback", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> disableRollback;
/**
* @return Set to true to disable rollback of the stack if stack creation failed.
* Conflicts with `on_failure`.
*
*/
public Output> disableRollback() {
return Codegen.optional(this.disableRollback);
}
/**
* The ARN of an IAM role that AWS CloudFormation assumes to create the stack. If you don't specify a value, AWS CloudFormation uses the role that was previously associated with the stack. If no role is available, AWS CloudFormation uses a temporary session that is generated from your user credentials.
*
*/
@Export(name="iamRoleArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> iamRoleArn;
/**
* @return The ARN of an IAM role that AWS CloudFormation assumes to create the stack. If you don't specify a value, AWS CloudFormation uses the role that was previously associated with the stack. If no role is available, AWS CloudFormation uses a temporary session that is generated from your user credentials.
*
*/
public Output> iamRoleArn() {
return Codegen.optional(this.iamRoleArn);
}
/**
* Stack name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Stack name.
*
*/
public Output name() {
return this.name;
}
/**
* A list of SNS topic ARNs to publish stack related events.
*
*/
@Export(name="notificationArns", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> notificationArns;
/**
* @return A list of SNS topic ARNs to publish stack related events.
*
*/
public Output>> notificationArns() {
return Codegen.optional(this.notificationArns);
}
/**
* Action to be taken if stack creation fails. This must be
* one of: `DO_NOTHING`, `ROLLBACK`, or `DELETE`. Conflicts with `disable_rollback`.
*
*/
@Export(name="onFailure", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> onFailure;
/**
* @return Action to be taken if stack creation fails. This must be
* one of: `DO_NOTHING`, `ROLLBACK`, or `DELETE`. Conflicts with `disable_rollback`.
*
*/
public Output> onFailure() {
return Codegen.optional(this.onFailure);
}
/**
* A map of outputs from the stack.
*
*/
@Export(name="outputs", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy