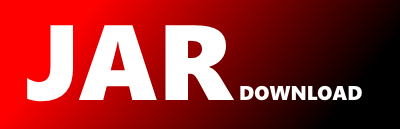
com.pulumi.aws.cloudformation.StackSetInstanceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudformation;
import com.pulumi.aws.cloudformation.inputs.StackSetInstanceDeploymentTargetsArgs;
import com.pulumi.aws.cloudformation.inputs.StackSetInstanceOperationPreferencesArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class StackSetInstanceArgs extends com.pulumi.resources.ResourceArgs {
public static final StackSetInstanceArgs Empty = new StackSetInstanceArgs();
/**
* Target AWS Account ID to create a Stack based on the StackSet. Defaults to current account.
*
*/
@Import(name="accountId")
private @Nullable Output accountId;
/**
* @return Target AWS Account ID to create a Stack based on the StackSet. Defaults to current account.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy