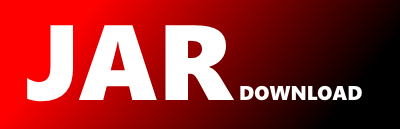
com.pulumi.aws.cloudformation.outputs.StackInstancesOperationPreferences Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudformation.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class StackInstancesOperationPreferences {
/**
* @return How the concurrency level behaves during the operation execution. Valid values are `STRICT_FAILURE_TOLERANCE` and `SOFT_FAILURE_TOLERANCE`.
*
*/
private @Nullable String concurrencyMode;
/**
* @return Number of accounts, per region, for which this operation can fail before CloudFormation stops the operation in that region.
*
*/
private @Nullable Integer failureToleranceCount;
/**
* @return Percentage of accounts, per region, for which this stack operation can fail before CloudFormation stops the operation in that region.
*
*/
private @Nullable Integer failureTolerancePercentage;
/**
* @return Maximum number of accounts in which to perform this operation at one time.
*
*/
private @Nullable Integer maxConcurrentCount;
/**
* @return Maximum percentage of accounts in which to perform this operation at one time.
*
*/
private @Nullable Integer maxConcurrentPercentage;
/**
* @return Concurrency type of deploying stack sets operations in regions, could be in parallel or one region at a time. Valid values are `SEQUENTIAL` and `PARALLEL`.
*
*/
private @Nullable String regionConcurrencyType;
/**
* @return Order of the regions where you want to perform the stack operation.
*
*/
private @Nullable List regionOrders;
private StackInstancesOperationPreferences() {}
/**
* @return How the concurrency level behaves during the operation execution. Valid values are `STRICT_FAILURE_TOLERANCE` and `SOFT_FAILURE_TOLERANCE`.
*
*/
public Optional concurrencyMode() {
return Optional.ofNullable(this.concurrencyMode);
}
/**
* @return Number of accounts, per region, for which this operation can fail before CloudFormation stops the operation in that region.
*
*/
public Optional failureToleranceCount() {
return Optional.ofNullable(this.failureToleranceCount);
}
/**
* @return Percentage of accounts, per region, for which this stack operation can fail before CloudFormation stops the operation in that region.
*
*/
public Optional failureTolerancePercentage() {
return Optional.ofNullable(this.failureTolerancePercentage);
}
/**
* @return Maximum number of accounts in which to perform this operation at one time.
*
*/
public Optional maxConcurrentCount() {
return Optional.ofNullable(this.maxConcurrentCount);
}
/**
* @return Maximum percentage of accounts in which to perform this operation at one time.
*
*/
public Optional maxConcurrentPercentage() {
return Optional.ofNullable(this.maxConcurrentPercentage);
}
/**
* @return Concurrency type of deploying stack sets operations in regions, could be in parallel or one region at a time. Valid values are `SEQUENTIAL` and `PARALLEL`.
*
*/
public Optional regionConcurrencyType() {
return Optional.ofNullable(this.regionConcurrencyType);
}
/**
* @return Order of the regions where you want to perform the stack operation.
*
*/
public List regionOrders() {
return this.regionOrders == null ? List.of() : this.regionOrders;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(StackInstancesOperationPreferences defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String concurrencyMode;
private @Nullable Integer failureToleranceCount;
private @Nullable Integer failureTolerancePercentage;
private @Nullable Integer maxConcurrentCount;
private @Nullable Integer maxConcurrentPercentage;
private @Nullable String regionConcurrencyType;
private @Nullable List regionOrders;
public Builder() {}
public Builder(StackInstancesOperationPreferences defaults) {
Objects.requireNonNull(defaults);
this.concurrencyMode = defaults.concurrencyMode;
this.failureToleranceCount = defaults.failureToleranceCount;
this.failureTolerancePercentage = defaults.failureTolerancePercentage;
this.maxConcurrentCount = defaults.maxConcurrentCount;
this.maxConcurrentPercentage = defaults.maxConcurrentPercentage;
this.regionConcurrencyType = defaults.regionConcurrencyType;
this.regionOrders = defaults.regionOrders;
}
@CustomType.Setter
public Builder concurrencyMode(@Nullable String concurrencyMode) {
this.concurrencyMode = concurrencyMode;
return this;
}
@CustomType.Setter
public Builder failureToleranceCount(@Nullable Integer failureToleranceCount) {
this.failureToleranceCount = failureToleranceCount;
return this;
}
@CustomType.Setter
public Builder failureTolerancePercentage(@Nullable Integer failureTolerancePercentage) {
this.failureTolerancePercentage = failureTolerancePercentage;
return this;
}
@CustomType.Setter
public Builder maxConcurrentCount(@Nullable Integer maxConcurrentCount) {
this.maxConcurrentCount = maxConcurrentCount;
return this;
}
@CustomType.Setter
public Builder maxConcurrentPercentage(@Nullable Integer maxConcurrentPercentage) {
this.maxConcurrentPercentage = maxConcurrentPercentage;
return this;
}
@CustomType.Setter
public Builder regionConcurrencyType(@Nullable String regionConcurrencyType) {
this.regionConcurrencyType = regionConcurrencyType;
return this;
}
@CustomType.Setter
public Builder regionOrders(@Nullable List regionOrders) {
this.regionOrders = regionOrders;
return this;
}
public Builder regionOrders(String... regionOrders) {
return regionOrders(List.of(regionOrders));
}
public StackInstancesOperationPreferences build() {
final var _resultValue = new StackInstancesOperationPreferences();
_resultValue.concurrencyMode = concurrencyMode;
_resultValue.failureToleranceCount = failureToleranceCount;
_resultValue.failureTolerancePercentage = failureTolerancePercentage;
_resultValue.maxConcurrentCount = maxConcurrentCount;
_resultValue.maxConcurrentPercentage = maxConcurrentPercentage;
_resultValue.regionConcurrencyType = regionConcurrencyType;
_resultValue.regionOrders = regionOrders;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy