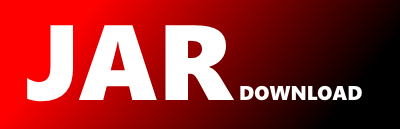
com.pulumi.aws.codebuild.outputs.ProjectArtifacts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.codebuild.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ProjectArtifacts {
/**
* @return Artifact identifier. Must be the same specified inside the AWS CodeBuild build specification.
*
*/
private @Nullable String artifactIdentifier;
/**
* @return Specifies the bucket owner's access for objects that another account uploads to their Amazon S3 bucket. By default, only the account that uploads the objects to the bucket has access to these objects. This property allows you to give the bucket owner access to these objects. Valid values are `NONE`, `READ_ONLY`, and `FULL`. your CodeBuild service role must have the `s3:PutBucketAcl` permission. This permission allows CodeBuild to modify the access control list for the bucket.
*
*/
private @Nullable String bucketOwnerAccess;
/**
* @return Whether to disable encrypting output artifacts. If `type` is set to `NO_ARTIFACTS`, this value is ignored. Defaults to `false`.
*
*/
private @Nullable Boolean encryptionDisabled;
/**
* @return Information about the build output artifact location. If `type` is set to `CODEPIPELINE` or `NO_ARTIFACTS`, this value is ignored. If `type` is set to `S3`, this is the name of the output bucket.
*
*/
private @Nullable String location;
/**
* @return Name of the project. If `type` is set to `S3`, this is the name of the output artifact object
*
*/
private @Nullable String name;
/**
* @return Namespace to use in storing build artifacts. If `type` is set to `S3`, then valid values are `BUILD_ID`, `NONE`.
*
*/
private @Nullable String namespaceType;
/**
* @return Whether a name specified in the build specification overrides the artifact name.
*
*/
private @Nullable Boolean overrideArtifactName;
/**
* @return Type of build output artifact to create. If `type` is set to `S3`, valid values are `NONE`, `ZIP`
*
*/
private @Nullable String packaging;
/**
* @return If `type` is set to `S3`, this is the path to the output artifact.
*
*/
private @Nullable String path;
/**
* @return Build output artifact's type. Valid values: `CODEPIPELINE`, `NO_ARTIFACTS`, `S3`.
*
*/
private String type;
private ProjectArtifacts() {}
/**
* @return Artifact identifier. Must be the same specified inside the AWS CodeBuild build specification.
*
*/
public Optional artifactIdentifier() {
return Optional.ofNullable(this.artifactIdentifier);
}
/**
* @return Specifies the bucket owner's access for objects that another account uploads to their Amazon S3 bucket. By default, only the account that uploads the objects to the bucket has access to these objects. This property allows you to give the bucket owner access to these objects. Valid values are `NONE`, `READ_ONLY`, and `FULL`. your CodeBuild service role must have the `s3:PutBucketAcl` permission. This permission allows CodeBuild to modify the access control list for the bucket.
*
*/
public Optional bucketOwnerAccess() {
return Optional.ofNullable(this.bucketOwnerAccess);
}
/**
* @return Whether to disable encrypting output artifacts. If `type` is set to `NO_ARTIFACTS`, this value is ignored. Defaults to `false`.
*
*/
public Optional encryptionDisabled() {
return Optional.ofNullable(this.encryptionDisabled);
}
/**
* @return Information about the build output artifact location. If `type` is set to `CODEPIPELINE` or `NO_ARTIFACTS`, this value is ignored. If `type` is set to `S3`, this is the name of the output bucket.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return Name of the project. If `type` is set to `S3`, this is the name of the output artifact object
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return Namespace to use in storing build artifacts. If `type` is set to `S3`, then valid values are `BUILD_ID`, `NONE`.
*
*/
public Optional namespaceType() {
return Optional.ofNullable(this.namespaceType);
}
/**
* @return Whether a name specified in the build specification overrides the artifact name.
*
*/
public Optional overrideArtifactName() {
return Optional.ofNullable(this.overrideArtifactName);
}
/**
* @return Type of build output artifact to create. If `type` is set to `S3`, valid values are `NONE`, `ZIP`
*
*/
public Optional packaging() {
return Optional.ofNullable(this.packaging);
}
/**
* @return If `type` is set to `S3`, this is the path to the output artifact.
*
*/
public Optional path() {
return Optional.ofNullable(this.path);
}
/**
* @return Build output artifact's type. Valid values: `CODEPIPELINE`, `NO_ARTIFACTS`, `S3`.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ProjectArtifacts defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String artifactIdentifier;
private @Nullable String bucketOwnerAccess;
private @Nullable Boolean encryptionDisabled;
private @Nullable String location;
private @Nullable String name;
private @Nullable String namespaceType;
private @Nullable Boolean overrideArtifactName;
private @Nullable String packaging;
private @Nullable String path;
private String type;
public Builder() {}
public Builder(ProjectArtifacts defaults) {
Objects.requireNonNull(defaults);
this.artifactIdentifier = defaults.artifactIdentifier;
this.bucketOwnerAccess = defaults.bucketOwnerAccess;
this.encryptionDisabled = defaults.encryptionDisabled;
this.location = defaults.location;
this.name = defaults.name;
this.namespaceType = defaults.namespaceType;
this.overrideArtifactName = defaults.overrideArtifactName;
this.packaging = defaults.packaging;
this.path = defaults.path;
this.type = defaults.type;
}
@CustomType.Setter
public Builder artifactIdentifier(@Nullable String artifactIdentifier) {
this.artifactIdentifier = artifactIdentifier;
return this;
}
@CustomType.Setter
public Builder bucketOwnerAccess(@Nullable String bucketOwnerAccess) {
this.bucketOwnerAccess = bucketOwnerAccess;
return this;
}
@CustomType.Setter
public Builder encryptionDisabled(@Nullable Boolean encryptionDisabled) {
this.encryptionDisabled = encryptionDisabled;
return this;
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder namespaceType(@Nullable String namespaceType) {
this.namespaceType = namespaceType;
return this;
}
@CustomType.Setter
public Builder overrideArtifactName(@Nullable Boolean overrideArtifactName) {
this.overrideArtifactName = overrideArtifactName;
return this;
}
@CustomType.Setter
public Builder packaging(@Nullable String packaging) {
this.packaging = packaging;
return this;
}
@CustomType.Setter
public Builder path(@Nullable String path) {
this.path = path;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("ProjectArtifacts", "type");
}
this.type = type;
return this;
}
public ProjectArtifacts build() {
final var _resultValue = new ProjectArtifacts();
_resultValue.artifactIdentifier = artifactIdentifier;
_resultValue.bucketOwnerAccess = bucketOwnerAccess;
_resultValue.encryptionDisabled = encryptionDisabled;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.namespaceType = namespaceType;
_resultValue.overrideArtifactName = overrideArtifactName;
_resultValue.packaging = packaging;
_resultValue.path = path;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy