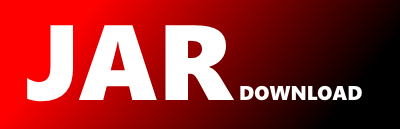
com.pulumi.aws.cognito.inputs.UserPoolUICustomizationState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cognito.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class UserPoolUICustomizationState extends com.pulumi.resources.ResourceArgs {
public static final UserPoolUICustomizationState Empty = new UserPoolUICustomizationState();
/**
* The client ID for the client app. Defaults to `ALL`. If `ALL` is specified, the `css` and/or `image_file` settings will be used for every client that has no UI customization set previously.
*
*/
@Import(name="clientId")
private @Nullable Output clientId;
/**
* @return The client ID for the client app. Defaults to `ALL`. If `ALL` is specified, the `css` and/or `image_file` settings will be used for every client that has no UI customization set previously.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy